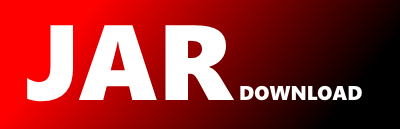
com.pulumi.azurenative.orbital.inputs.ContactProfileLinkArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.orbital.inputs;
import com.pulumi.azurenative.orbital.enums.Direction;
import com.pulumi.azurenative.orbital.enums.Polarization;
import com.pulumi.azurenative.orbital.inputs.ContactProfileLinkChannelArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Contact Profile Link.
*
*/
public final class ContactProfileLinkArgs extends com.pulumi.resources.ResourceArgs {
public static final ContactProfileLinkArgs Empty = new ContactProfileLinkArgs();
/**
* Contact Profile Link Channel.
*
*/
@Import(name="channels", required=true)
private Output> channels;
/**
* @return Contact Profile Link Channel.
*
*/
public Output> channels() {
return this.channels;
}
/**
* Direction (Uplink or Downlink).
*
*/
@Import(name="direction", required=true)
private Output> direction;
/**
* @return Direction (Uplink or Downlink).
*
*/
public Output> direction() {
return this.direction;
}
/**
* Effective Isotropic Radiated Power (EIRP) in dBW. It is the required EIRP by the customer. Not used yet.
*
*/
@Import(name="eirpdBW")
private @Nullable Output eirpdBW;
/**
* @return Effective Isotropic Radiated Power (EIRP) in dBW. It is the required EIRP by the customer. Not used yet.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy