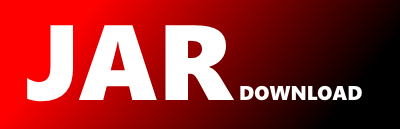
com.pulumi.azurenative.peering.PeeringFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.peering;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.peering.inputs.GetConnectionMonitorTestArgs;
import com.pulumi.azurenative.peering.inputs.GetConnectionMonitorTestPlainArgs;
import com.pulumi.azurenative.peering.inputs.GetPeerAsnArgs;
import com.pulumi.azurenative.peering.inputs.GetPeerAsnPlainArgs;
import com.pulumi.azurenative.peering.inputs.GetPeeringArgs;
import com.pulumi.azurenative.peering.inputs.GetPeeringPlainArgs;
import com.pulumi.azurenative.peering.inputs.GetPeeringServiceArgs;
import com.pulumi.azurenative.peering.inputs.GetPeeringServicePlainArgs;
import com.pulumi.azurenative.peering.inputs.GetPrefixArgs;
import com.pulumi.azurenative.peering.inputs.GetPrefixPlainArgs;
import com.pulumi.azurenative.peering.inputs.GetRegisteredAsnArgs;
import com.pulumi.azurenative.peering.inputs.GetRegisteredAsnPlainArgs;
import com.pulumi.azurenative.peering.inputs.GetRegisteredPrefixArgs;
import com.pulumi.azurenative.peering.inputs.GetRegisteredPrefixPlainArgs;
import com.pulumi.azurenative.peering.outputs.GetConnectionMonitorTestResult;
import com.pulumi.azurenative.peering.outputs.GetPeerAsnResult;
import com.pulumi.azurenative.peering.outputs.GetPeeringResult;
import com.pulumi.azurenative.peering.outputs.GetPeeringServiceResult;
import com.pulumi.azurenative.peering.outputs.GetPrefixResult;
import com.pulumi.azurenative.peering.outputs.GetRegisteredAsnResult;
import com.pulumi.azurenative.peering.outputs.GetRegisteredPrefixResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class PeeringFunctions {
/**
* Gets an existing connection monitor test with the specified name under the given subscription, resource group and peering service.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getConnectionMonitorTest(GetConnectionMonitorTestArgs args) {
return getConnectionMonitorTest(args, InvokeOptions.Empty);
}
/**
* Gets an existing connection monitor test with the specified name under the given subscription, resource group and peering service.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getConnectionMonitorTestPlain(GetConnectionMonitorTestPlainArgs args) {
return getConnectionMonitorTestPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing connection monitor test with the specified name under the given subscription, resource group and peering service.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getConnectionMonitorTest(GetConnectionMonitorTestArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:peering:getConnectionMonitorTest", TypeShape.of(GetConnectionMonitorTestResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing connection monitor test with the specified name under the given subscription, resource group and peering service.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getConnectionMonitorTestPlain(GetConnectionMonitorTestPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:peering:getConnectionMonitorTest", TypeShape.of(GetConnectionMonitorTestResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the peer ASN with the specified name under the given subscription.
* Azure REST API version: 2022-10-01.
*
* Other available API versions: 2019-09-01-preview, 2021-01-01.
*
*/
public static Output getPeerAsn(GetPeerAsnArgs args) {
return getPeerAsn(args, InvokeOptions.Empty);
}
/**
* Gets the peer ASN with the specified name under the given subscription.
* Azure REST API version: 2022-10-01.
*
* Other available API versions: 2019-09-01-preview, 2021-01-01.
*
*/
public static CompletableFuture getPeerAsnPlain(GetPeerAsnPlainArgs args) {
return getPeerAsnPlain(args, InvokeOptions.Empty);
}
/**
* Gets the peer ASN with the specified name under the given subscription.
* Azure REST API version: 2022-10-01.
*
* Other available API versions: 2019-09-01-preview, 2021-01-01.
*
*/
public static Output getPeerAsn(GetPeerAsnArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:peering:getPeerAsn", TypeShape.of(GetPeerAsnResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the peer ASN with the specified name under the given subscription.
* Azure REST API version: 2022-10-01.
*
* Other available API versions: 2019-09-01-preview, 2021-01-01.
*
*/
public static CompletableFuture getPeerAsnPlain(GetPeerAsnPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:peering:getPeerAsn", TypeShape.of(GetPeerAsnResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing peering with the specified name under the given subscription and resource group.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getPeering(GetPeeringArgs args) {
return getPeering(args, InvokeOptions.Empty);
}
/**
* Gets an existing peering with the specified name under the given subscription and resource group.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getPeeringPlain(GetPeeringPlainArgs args) {
return getPeeringPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing peering with the specified name under the given subscription and resource group.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getPeering(GetPeeringArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:peering:getPeering", TypeShape.of(GetPeeringResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing peering with the specified name under the given subscription and resource group.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getPeeringPlain(GetPeeringPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:peering:getPeering", TypeShape.of(GetPeeringResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing peering service with the specified name under the given subscription and resource group.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getPeeringService(GetPeeringServiceArgs args) {
return getPeeringService(args, InvokeOptions.Empty);
}
/**
* Gets an existing peering service with the specified name under the given subscription and resource group.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getPeeringServicePlain(GetPeeringServicePlainArgs args) {
return getPeeringServicePlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing peering service with the specified name under the given subscription and resource group.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getPeeringService(GetPeeringServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:peering:getPeeringService", TypeShape.of(GetPeeringServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing peering service with the specified name under the given subscription and resource group.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getPeeringServicePlain(GetPeeringServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:peering:getPeeringService", TypeShape.of(GetPeeringServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing prefix with the specified name under the given subscription, resource group and peering service.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getPrefix(GetPrefixArgs args) {
return getPrefix(args, InvokeOptions.Empty);
}
/**
* Gets an existing prefix with the specified name under the given subscription, resource group and peering service.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getPrefixPlain(GetPrefixPlainArgs args) {
return getPrefixPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing prefix with the specified name under the given subscription, resource group and peering service.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getPrefix(GetPrefixArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:peering:getPrefix", TypeShape.of(GetPrefixResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing prefix with the specified name under the given subscription, resource group and peering service.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getPrefixPlain(GetPrefixPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:peering:getPrefix", TypeShape.of(GetPrefixResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing registered ASN with the specified name under the given subscription, resource group and peering.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getRegisteredAsn(GetRegisteredAsnArgs args) {
return getRegisteredAsn(args, InvokeOptions.Empty);
}
/**
* Gets an existing registered ASN with the specified name under the given subscription, resource group and peering.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getRegisteredAsnPlain(GetRegisteredAsnPlainArgs args) {
return getRegisteredAsnPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing registered ASN with the specified name under the given subscription, resource group and peering.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getRegisteredAsn(GetRegisteredAsnArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:peering:getRegisteredAsn", TypeShape.of(GetRegisteredAsnResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing registered ASN with the specified name under the given subscription, resource group and peering.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getRegisteredAsnPlain(GetRegisteredAsnPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:peering:getRegisteredAsn", TypeShape.of(GetRegisteredAsnResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing registered prefix with the specified name under the given subscription, resource group and peering.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getRegisteredPrefix(GetRegisteredPrefixArgs args) {
return getRegisteredPrefix(args, InvokeOptions.Empty);
}
/**
* Gets an existing registered prefix with the specified name under the given subscription, resource group and peering.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getRegisteredPrefixPlain(GetRegisteredPrefixPlainArgs args) {
return getRegisteredPrefixPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing registered prefix with the specified name under the given subscription, resource group and peering.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getRegisteredPrefix(GetRegisteredPrefixArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:peering:getRegisteredPrefix", TypeShape.of(GetRegisteredPrefixResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing registered prefix with the specified name under the given subscription, resource group and peering.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getRegisteredPrefixPlain(GetRegisteredPrefixPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:peering:getRegisteredPrefix", TypeShape.of(GetRegisteredPrefixResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy