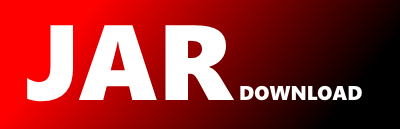
com.pulumi.azurenative.recoveryservices.outputs.A2AProtectedManagedDiskDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class A2AProtectedManagedDiskDetailsResponse {
/**
* @return The disk level operations list.
*
*/
private @Nullable List allowedDiskLevelOperation;
/**
* @return The data pending at source virtual machine in MB.
*
*/
private @Nullable Double dataPendingAtSourceAgentInMB;
/**
* @return The data pending for replication in MB at staging account.
*
*/
private @Nullable Double dataPendingInStagingStorageAccountInMB;
/**
* @return The KeyVault resource id for secret (BEK).
*
*/
private @Nullable String dekKeyVaultArmId;
/**
* @return The disk capacity in bytes.
*
*/
private @Nullable Double diskCapacityInBytes;
/**
* @return The managed disk Arm id.
*
*/
private @Nullable String diskId;
/**
* @return The disk name.
*
*/
private @Nullable String diskName;
/**
* @return The disk state.
*
*/
private @Nullable String diskState;
/**
* @return The type of disk.
*
*/
private @Nullable String diskType;
/**
* @return The failover name for the managed disk.
*
*/
private @Nullable String failoverDiskName;
/**
* @return A value indicating whether vm has encrypted os disk or not.
*
*/
private @Nullable Boolean isDiskEncrypted;
/**
* @return A value indicating whether disk key got encrypted or not.
*
*/
private @Nullable Boolean isDiskKeyEncrypted;
/**
* @return The KeyVault resource id for key (KEK).
*
*/
private @Nullable String kekKeyVaultArmId;
/**
* @return The key URL / identifier (KEK).
*
*/
private @Nullable String keyIdentifier;
/**
* @return The type of the monitoring job. The progress is contained in MonitoringPercentageCompletion property.
*
*/
private @Nullable String monitoringJobType;
/**
* @return The percentage of the monitoring job. The type of the monitoring job is defined by MonitoringJobType property.
*
*/
private @Nullable Integer monitoringPercentageCompletion;
/**
* @return The primary disk encryption set Id.
*
*/
private @Nullable String primaryDiskEncryptionSetId;
/**
* @return The primary staging storage account.
*
*/
private @Nullable String primaryStagingAzureStorageAccountId;
/**
* @return The recovery disk encryption set Id.
*
*/
private @Nullable String recoveryDiskEncryptionSetId;
/**
* @return Recovery original target disk Arm Id.
*
*/
private @Nullable String recoveryOrignalTargetDiskId;
/**
* @return The replica disk type. Its an optional value and will be same as source disk type if not user provided.
*
*/
private @Nullable String recoveryReplicaDiskAccountType;
/**
* @return Recovery replica disk Arm Id.
*
*/
private @Nullable String recoveryReplicaDiskId;
/**
* @return The recovery disk resource group Arm Id.
*
*/
private @Nullable String recoveryResourceGroupId;
/**
* @return The target disk type after failover. Its an optional value and will be same as source disk type if not user provided.
*
*/
private @Nullable String recoveryTargetDiskAccountType;
/**
* @return Recovery target disk Arm Id.
*
*/
private @Nullable String recoveryTargetDiskId;
/**
* @return A value indicating whether resync is required for this disk.
*
*/
private @Nullable Boolean resyncRequired;
/**
* @return The secret URL / identifier (BEK).
*
*/
private @Nullable String secretIdentifier;
/**
* @return The test failover name for the managed disk.
*
*/
private @Nullable String tfoDiskName;
private A2AProtectedManagedDiskDetailsResponse() {}
/**
* @return The disk level operations list.
*
*/
public List allowedDiskLevelOperation() {
return this.allowedDiskLevelOperation == null ? List.of() : this.allowedDiskLevelOperation;
}
/**
* @return The data pending at source virtual machine in MB.
*
*/
public Optional dataPendingAtSourceAgentInMB() {
return Optional.ofNullable(this.dataPendingAtSourceAgentInMB);
}
/**
* @return The data pending for replication in MB at staging account.
*
*/
public Optional dataPendingInStagingStorageAccountInMB() {
return Optional.ofNullable(this.dataPendingInStagingStorageAccountInMB);
}
/**
* @return The KeyVault resource id for secret (BEK).
*
*/
public Optional dekKeyVaultArmId() {
return Optional.ofNullable(this.dekKeyVaultArmId);
}
/**
* @return The disk capacity in bytes.
*
*/
public Optional diskCapacityInBytes() {
return Optional.ofNullable(this.diskCapacityInBytes);
}
/**
* @return The managed disk Arm id.
*
*/
public Optional diskId() {
return Optional.ofNullable(this.diskId);
}
/**
* @return The disk name.
*
*/
public Optional diskName() {
return Optional.ofNullable(this.diskName);
}
/**
* @return The disk state.
*
*/
public Optional diskState() {
return Optional.ofNullable(this.diskState);
}
/**
* @return The type of disk.
*
*/
public Optional diskType() {
return Optional.ofNullable(this.diskType);
}
/**
* @return The failover name for the managed disk.
*
*/
public Optional failoverDiskName() {
return Optional.ofNullable(this.failoverDiskName);
}
/**
* @return A value indicating whether vm has encrypted os disk or not.
*
*/
public Optional isDiskEncrypted() {
return Optional.ofNullable(this.isDiskEncrypted);
}
/**
* @return A value indicating whether disk key got encrypted or not.
*
*/
public Optional isDiskKeyEncrypted() {
return Optional.ofNullable(this.isDiskKeyEncrypted);
}
/**
* @return The KeyVault resource id for key (KEK).
*
*/
public Optional kekKeyVaultArmId() {
return Optional.ofNullable(this.kekKeyVaultArmId);
}
/**
* @return The key URL / identifier (KEK).
*
*/
public Optional keyIdentifier() {
return Optional.ofNullable(this.keyIdentifier);
}
/**
* @return The type of the monitoring job. The progress is contained in MonitoringPercentageCompletion property.
*
*/
public Optional monitoringJobType() {
return Optional.ofNullable(this.monitoringJobType);
}
/**
* @return The percentage of the monitoring job. The type of the monitoring job is defined by MonitoringJobType property.
*
*/
public Optional monitoringPercentageCompletion() {
return Optional.ofNullable(this.monitoringPercentageCompletion);
}
/**
* @return The primary disk encryption set Id.
*
*/
public Optional primaryDiskEncryptionSetId() {
return Optional.ofNullable(this.primaryDiskEncryptionSetId);
}
/**
* @return The primary staging storage account.
*
*/
public Optional primaryStagingAzureStorageAccountId() {
return Optional.ofNullable(this.primaryStagingAzureStorageAccountId);
}
/**
* @return The recovery disk encryption set Id.
*
*/
public Optional recoveryDiskEncryptionSetId() {
return Optional.ofNullable(this.recoveryDiskEncryptionSetId);
}
/**
* @return Recovery original target disk Arm Id.
*
*/
public Optional recoveryOrignalTargetDiskId() {
return Optional.ofNullable(this.recoveryOrignalTargetDiskId);
}
/**
* @return The replica disk type. Its an optional value and will be same as source disk type if not user provided.
*
*/
public Optional recoveryReplicaDiskAccountType() {
return Optional.ofNullable(this.recoveryReplicaDiskAccountType);
}
/**
* @return Recovery replica disk Arm Id.
*
*/
public Optional recoveryReplicaDiskId() {
return Optional.ofNullable(this.recoveryReplicaDiskId);
}
/**
* @return The recovery disk resource group Arm Id.
*
*/
public Optional recoveryResourceGroupId() {
return Optional.ofNullable(this.recoveryResourceGroupId);
}
/**
* @return The target disk type after failover. Its an optional value and will be same as source disk type if not user provided.
*
*/
public Optional recoveryTargetDiskAccountType() {
return Optional.ofNullable(this.recoveryTargetDiskAccountType);
}
/**
* @return Recovery target disk Arm Id.
*
*/
public Optional recoveryTargetDiskId() {
return Optional.ofNullable(this.recoveryTargetDiskId);
}
/**
* @return A value indicating whether resync is required for this disk.
*
*/
public Optional resyncRequired() {
return Optional.ofNullable(this.resyncRequired);
}
/**
* @return The secret URL / identifier (BEK).
*
*/
public Optional secretIdentifier() {
return Optional.ofNullable(this.secretIdentifier);
}
/**
* @return The test failover name for the managed disk.
*
*/
public Optional tfoDiskName() {
return Optional.ofNullable(this.tfoDiskName);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(A2AProtectedManagedDiskDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List allowedDiskLevelOperation;
private @Nullable Double dataPendingAtSourceAgentInMB;
private @Nullable Double dataPendingInStagingStorageAccountInMB;
private @Nullable String dekKeyVaultArmId;
private @Nullable Double diskCapacityInBytes;
private @Nullable String diskId;
private @Nullable String diskName;
private @Nullable String diskState;
private @Nullable String diskType;
private @Nullable String failoverDiskName;
private @Nullable Boolean isDiskEncrypted;
private @Nullable Boolean isDiskKeyEncrypted;
private @Nullable String kekKeyVaultArmId;
private @Nullable String keyIdentifier;
private @Nullable String monitoringJobType;
private @Nullable Integer monitoringPercentageCompletion;
private @Nullable String primaryDiskEncryptionSetId;
private @Nullable String primaryStagingAzureStorageAccountId;
private @Nullable String recoveryDiskEncryptionSetId;
private @Nullable String recoveryOrignalTargetDiskId;
private @Nullable String recoveryReplicaDiskAccountType;
private @Nullable String recoveryReplicaDiskId;
private @Nullable String recoveryResourceGroupId;
private @Nullable String recoveryTargetDiskAccountType;
private @Nullable String recoveryTargetDiskId;
private @Nullable Boolean resyncRequired;
private @Nullable String secretIdentifier;
private @Nullable String tfoDiskName;
public Builder() {}
public Builder(A2AProtectedManagedDiskDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.allowedDiskLevelOperation = defaults.allowedDiskLevelOperation;
this.dataPendingAtSourceAgentInMB = defaults.dataPendingAtSourceAgentInMB;
this.dataPendingInStagingStorageAccountInMB = defaults.dataPendingInStagingStorageAccountInMB;
this.dekKeyVaultArmId = defaults.dekKeyVaultArmId;
this.diskCapacityInBytes = defaults.diskCapacityInBytes;
this.diskId = defaults.diskId;
this.diskName = defaults.diskName;
this.diskState = defaults.diskState;
this.diskType = defaults.diskType;
this.failoverDiskName = defaults.failoverDiskName;
this.isDiskEncrypted = defaults.isDiskEncrypted;
this.isDiskKeyEncrypted = defaults.isDiskKeyEncrypted;
this.kekKeyVaultArmId = defaults.kekKeyVaultArmId;
this.keyIdentifier = defaults.keyIdentifier;
this.monitoringJobType = defaults.monitoringJobType;
this.monitoringPercentageCompletion = defaults.monitoringPercentageCompletion;
this.primaryDiskEncryptionSetId = defaults.primaryDiskEncryptionSetId;
this.primaryStagingAzureStorageAccountId = defaults.primaryStagingAzureStorageAccountId;
this.recoveryDiskEncryptionSetId = defaults.recoveryDiskEncryptionSetId;
this.recoveryOrignalTargetDiskId = defaults.recoveryOrignalTargetDiskId;
this.recoveryReplicaDiskAccountType = defaults.recoveryReplicaDiskAccountType;
this.recoveryReplicaDiskId = defaults.recoveryReplicaDiskId;
this.recoveryResourceGroupId = defaults.recoveryResourceGroupId;
this.recoveryTargetDiskAccountType = defaults.recoveryTargetDiskAccountType;
this.recoveryTargetDiskId = defaults.recoveryTargetDiskId;
this.resyncRequired = defaults.resyncRequired;
this.secretIdentifier = defaults.secretIdentifier;
this.tfoDiskName = defaults.tfoDiskName;
}
@CustomType.Setter
public Builder allowedDiskLevelOperation(@Nullable List allowedDiskLevelOperation) {
this.allowedDiskLevelOperation = allowedDiskLevelOperation;
return this;
}
public Builder allowedDiskLevelOperation(String... allowedDiskLevelOperation) {
return allowedDiskLevelOperation(List.of(allowedDiskLevelOperation));
}
@CustomType.Setter
public Builder dataPendingAtSourceAgentInMB(@Nullable Double dataPendingAtSourceAgentInMB) {
this.dataPendingAtSourceAgentInMB = dataPendingAtSourceAgentInMB;
return this;
}
@CustomType.Setter
public Builder dataPendingInStagingStorageAccountInMB(@Nullable Double dataPendingInStagingStorageAccountInMB) {
this.dataPendingInStagingStorageAccountInMB = dataPendingInStagingStorageAccountInMB;
return this;
}
@CustomType.Setter
public Builder dekKeyVaultArmId(@Nullable String dekKeyVaultArmId) {
this.dekKeyVaultArmId = dekKeyVaultArmId;
return this;
}
@CustomType.Setter
public Builder diskCapacityInBytes(@Nullable Double diskCapacityInBytes) {
this.diskCapacityInBytes = diskCapacityInBytes;
return this;
}
@CustomType.Setter
public Builder diskId(@Nullable String diskId) {
this.diskId = diskId;
return this;
}
@CustomType.Setter
public Builder diskName(@Nullable String diskName) {
this.diskName = diskName;
return this;
}
@CustomType.Setter
public Builder diskState(@Nullable String diskState) {
this.diskState = diskState;
return this;
}
@CustomType.Setter
public Builder diskType(@Nullable String diskType) {
this.diskType = diskType;
return this;
}
@CustomType.Setter
public Builder failoverDiskName(@Nullable String failoverDiskName) {
this.failoverDiskName = failoverDiskName;
return this;
}
@CustomType.Setter
public Builder isDiskEncrypted(@Nullable Boolean isDiskEncrypted) {
this.isDiskEncrypted = isDiskEncrypted;
return this;
}
@CustomType.Setter
public Builder isDiskKeyEncrypted(@Nullable Boolean isDiskKeyEncrypted) {
this.isDiskKeyEncrypted = isDiskKeyEncrypted;
return this;
}
@CustomType.Setter
public Builder kekKeyVaultArmId(@Nullable String kekKeyVaultArmId) {
this.kekKeyVaultArmId = kekKeyVaultArmId;
return this;
}
@CustomType.Setter
public Builder keyIdentifier(@Nullable String keyIdentifier) {
this.keyIdentifier = keyIdentifier;
return this;
}
@CustomType.Setter
public Builder monitoringJobType(@Nullable String monitoringJobType) {
this.monitoringJobType = monitoringJobType;
return this;
}
@CustomType.Setter
public Builder monitoringPercentageCompletion(@Nullable Integer monitoringPercentageCompletion) {
this.monitoringPercentageCompletion = monitoringPercentageCompletion;
return this;
}
@CustomType.Setter
public Builder primaryDiskEncryptionSetId(@Nullable String primaryDiskEncryptionSetId) {
this.primaryDiskEncryptionSetId = primaryDiskEncryptionSetId;
return this;
}
@CustomType.Setter
public Builder primaryStagingAzureStorageAccountId(@Nullable String primaryStagingAzureStorageAccountId) {
this.primaryStagingAzureStorageAccountId = primaryStagingAzureStorageAccountId;
return this;
}
@CustomType.Setter
public Builder recoveryDiskEncryptionSetId(@Nullable String recoveryDiskEncryptionSetId) {
this.recoveryDiskEncryptionSetId = recoveryDiskEncryptionSetId;
return this;
}
@CustomType.Setter
public Builder recoveryOrignalTargetDiskId(@Nullable String recoveryOrignalTargetDiskId) {
this.recoveryOrignalTargetDiskId = recoveryOrignalTargetDiskId;
return this;
}
@CustomType.Setter
public Builder recoveryReplicaDiskAccountType(@Nullable String recoveryReplicaDiskAccountType) {
this.recoveryReplicaDiskAccountType = recoveryReplicaDiskAccountType;
return this;
}
@CustomType.Setter
public Builder recoveryReplicaDiskId(@Nullable String recoveryReplicaDiskId) {
this.recoveryReplicaDiskId = recoveryReplicaDiskId;
return this;
}
@CustomType.Setter
public Builder recoveryResourceGroupId(@Nullable String recoveryResourceGroupId) {
this.recoveryResourceGroupId = recoveryResourceGroupId;
return this;
}
@CustomType.Setter
public Builder recoveryTargetDiskAccountType(@Nullable String recoveryTargetDiskAccountType) {
this.recoveryTargetDiskAccountType = recoveryTargetDiskAccountType;
return this;
}
@CustomType.Setter
public Builder recoveryTargetDiskId(@Nullable String recoveryTargetDiskId) {
this.recoveryTargetDiskId = recoveryTargetDiskId;
return this;
}
@CustomType.Setter
public Builder resyncRequired(@Nullable Boolean resyncRequired) {
this.resyncRequired = resyncRequired;
return this;
}
@CustomType.Setter
public Builder secretIdentifier(@Nullable String secretIdentifier) {
this.secretIdentifier = secretIdentifier;
return this;
}
@CustomType.Setter
public Builder tfoDiskName(@Nullable String tfoDiskName) {
this.tfoDiskName = tfoDiskName;
return this;
}
public A2AProtectedManagedDiskDetailsResponse build() {
final var _resultValue = new A2AProtectedManagedDiskDetailsResponse();
_resultValue.allowedDiskLevelOperation = allowedDiskLevelOperation;
_resultValue.dataPendingAtSourceAgentInMB = dataPendingAtSourceAgentInMB;
_resultValue.dataPendingInStagingStorageAccountInMB = dataPendingInStagingStorageAccountInMB;
_resultValue.dekKeyVaultArmId = dekKeyVaultArmId;
_resultValue.diskCapacityInBytes = diskCapacityInBytes;
_resultValue.diskId = diskId;
_resultValue.diskName = diskName;
_resultValue.diskState = diskState;
_resultValue.diskType = diskType;
_resultValue.failoverDiskName = failoverDiskName;
_resultValue.isDiskEncrypted = isDiskEncrypted;
_resultValue.isDiskKeyEncrypted = isDiskKeyEncrypted;
_resultValue.kekKeyVaultArmId = kekKeyVaultArmId;
_resultValue.keyIdentifier = keyIdentifier;
_resultValue.monitoringJobType = monitoringJobType;
_resultValue.monitoringPercentageCompletion = monitoringPercentageCompletion;
_resultValue.primaryDiskEncryptionSetId = primaryDiskEncryptionSetId;
_resultValue.primaryStagingAzureStorageAccountId = primaryStagingAzureStorageAccountId;
_resultValue.recoveryDiskEncryptionSetId = recoveryDiskEncryptionSetId;
_resultValue.recoveryOrignalTargetDiskId = recoveryOrignalTargetDiskId;
_resultValue.recoveryReplicaDiskAccountType = recoveryReplicaDiskAccountType;
_resultValue.recoveryReplicaDiskId = recoveryReplicaDiskId;
_resultValue.recoveryResourceGroupId = recoveryResourceGroupId;
_resultValue.recoveryTargetDiskAccountType = recoveryTargetDiskAccountType;
_resultValue.recoveryTargetDiskId = recoveryTargetDiskId;
_resultValue.resyncRequired = resyncRequired;
_resultValue.secretIdentifier = secretIdentifier;
_resultValue.tfoDiskName = tfoDiskName;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy