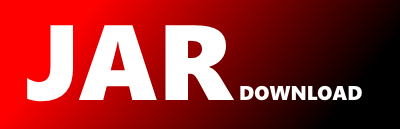
com.pulumi.azurenative.recoveryservices.outputs.AzureSQLAGWorkloadContainerProtectionContainerResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.AzureWorkloadContainerExtendedInfoResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureSQLAGWorkloadContainerProtectionContainerResponse {
/**
* @return Type of backup management for the container.
*
*/
private @Nullable String backupManagementType;
/**
* @return Type of the container. The value of this property for: 1. Compute Azure VM is Microsoft.Compute/virtualMachines 2.
* Classic Compute Azure VM is Microsoft.ClassicCompute/virtualMachines 3. Windows machines (like MAB, DPM etc) is
* Windows 4. Azure SQL instance is AzureSqlContainer. 5. Storage containers is StorageContainer. 6. Azure workload
* Backup is VMAppContainer
* Expected value is 'SQLAGWorkLoadContainer'.
*
*/
private String containerType;
/**
* @return Additional details of a workload container.
*
*/
private @Nullable AzureWorkloadContainerExtendedInfoResponse extendedInfo;
/**
* @return Friendly name of the container.
*
*/
private @Nullable String friendlyName;
/**
* @return Status of health of the container.
*
*/
private @Nullable String healthStatus;
/**
* @return Time stamp when this container was updated.
*
*/
private @Nullable String lastUpdatedTime;
/**
* @return Re-Do Operation
*
*/
private @Nullable String operationType;
/**
* @return Type of the protectable object associated with this container
*
*/
private @Nullable String protectableObjectType;
/**
* @return Status of registration of the container with the Recovery Services Vault.
*
*/
private @Nullable String registrationStatus;
/**
* @return ARM ID of the virtual machine represented by this Azure Workload Container
*
*/
private @Nullable String sourceResourceId;
/**
* @return Workload type for which registration was sent.
*
*/
private @Nullable String workloadType;
private AzureSQLAGWorkloadContainerProtectionContainerResponse() {}
/**
* @return Type of backup management for the container.
*
*/
public Optional backupManagementType() {
return Optional.ofNullable(this.backupManagementType);
}
/**
* @return Type of the container. The value of this property for: 1. Compute Azure VM is Microsoft.Compute/virtualMachines 2.
* Classic Compute Azure VM is Microsoft.ClassicCompute/virtualMachines 3. Windows machines (like MAB, DPM etc) is
* Windows 4. Azure SQL instance is AzureSqlContainer. 5. Storage containers is StorageContainer. 6. Azure workload
* Backup is VMAppContainer
* Expected value is 'SQLAGWorkLoadContainer'.
*
*/
public String containerType() {
return this.containerType;
}
/**
* @return Additional details of a workload container.
*
*/
public Optional extendedInfo() {
return Optional.ofNullable(this.extendedInfo);
}
/**
* @return Friendly name of the container.
*
*/
public Optional friendlyName() {
return Optional.ofNullable(this.friendlyName);
}
/**
* @return Status of health of the container.
*
*/
public Optional healthStatus() {
return Optional.ofNullable(this.healthStatus);
}
/**
* @return Time stamp when this container was updated.
*
*/
public Optional lastUpdatedTime() {
return Optional.ofNullable(this.lastUpdatedTime);
}
/**
* @return Re-Do Operation
*
*/
public Optional operationType() {
return Optional.ofNullable(this.operationType);
}
/**
* @return Type of the protectable object associated with this container
*
*/
public Optional protectableObjectType() {
return Optional.ofNullable(this.protectableObjectType);
}
/**
* @return Status of registration of the container with the Recovery Services Vault.
*
*/
public Optional registrationStatus() {
return Optional.ofNullable(this.registrationStatus);
}
/**
* @return ARM ID of the virtual machine represented by this Azure Workload Container
*
*/
public Optional sourceResourceId() {
return Optional.ofNullable(this.sourceResourceId);
}
/**
* @return Workload type for which registration was sent.
*
*/
public Optional workloadType() {
return Optional.ofNullable(this.workloadType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureSQLAGWorkloadContainerProtectionContainerResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String backupManagementType;
private String containerType;
private @Nullable AzureWorkloadContainerExtendedInfoResponse extendedInfo;
private @Nullable String friendlyName;
private @Nullable String healthStatus;
private @Nullable String lastUpdatedTime;
private @Nullable String operationType;
private @Nullable String protectableObjectType;
private @Nullable String registrationStatus;
private @Nullable String sourceResourceId;
private @Nullable String workloadType;
public Builder() {}
public Builder(AzureSQLAGWorkloadContainerProtectionContainerResponse defaults) {
Objects.requireNonNull(defaults);
this.backupManagementType = defaults.backupManagementType;
this.containerType = defaults.containerType;
this.extendedInfo = defaults.extendedInfo;
this.friendlyName = defaults.friendlyName;
this.healthStatus = defaults.healthStatus;
this.lastUpdatedTime = defaults.lastUpdatedTime;
this.operationType = defaults.operationType;
this.protectableObjectType = defaults.protectableObjectType;
this.registrationStatus = defaults.registrationStatus;
this.sourceResourceId = defaults.sourceResourceId;
this.workloadType = defaults.workloadType;
}
@CustomType.Setter
public Builder backupManagementType(@Nullable String backupManagementType) {
this.backupManagementType = backupManagementType;
return this;
}
@CustomType.Setter
public Builder containerType(String containerType) {
if (containerType == null) {
throw new MissingRequiredPropertyException("AzureSQLAGWorkloadContainerProtectionContainerResponse", "containerType");
}
this.containerType = containerType;
return this;
}
@CustomType.Setter
public Builder extendedInfo(@Nullable AzureWorkloadContainerExtendedInfoResponse extendedInfo) {
this.extendedInfo = extendedInfo;
return this;
}
@CustomType.Setter
public Builder friendlyName(@Nullable String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
@CustomType.Setter
public Builder healthStatus(@Nullable String healthStatus) {
this.healthStatus = healthStatus;
return this;
}
@CustomType.Setter
public Builder lastUpdatedTime(@Nullable String lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
return this;
}
@CustomType.Setter
public Builder operationType(@Nullable String operationType) {
this.operationType = operationType;
return this;
}
@CustomType.Setter
public Builder protectableObjectType(@Nullable String protectableObjectType) {
this.protectableObjectType = protectableObjectType;
return this;
}
@CustomType.Setter
public Builder registrationStatus(@Nullable String registrationStatus) {
this.registrationStatus = registrationStatus;
return this;
}
@CustomType.Setter
public Builder sourceResourceId(@Nullable String sourceResourceId) {
this.sourceResourceId = sourceResourceId;
return this;
}
@CustomType.Setter
public Builder workloadType(@Nullable String workloadType) {
this.workloadType = workloadType;
return this;
}
public AzureSQLAGWorkloadContainerProtectionContainerResponse build() {
final var _resultValue = new AzureSQLAGWorkloadContainerProtectionContainerResponse();
_resultValue.backupManagementType = backupManagementType;
_resultValue.containerType = containerType;
_resultValue.extendedInfo = extendedInfo;
_resultValue.friendlyName = friendlyName;
_resultValue.healthStatus = healthStatus;
_resultValue.lastUpdatedTime = lastUpdatedTime;
_resultValue.operationType = operationType;
_resultValue.protectableObjectType = protectableObjectType;
_resultValue.registrationStatus = registrationStatus;
_resultValue.sourceResourceId = sourceResourceId;
_resultValue.workloadType = workloadType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy