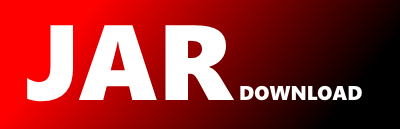
com.pulumi.azurenative.recoveryservices.outputs.HyperVReplicaBasePolicyDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class HyperVReplicaBasePolicyDetailsResponse {
/**
* @return A value indicating the authentication type.
*
*/
private @Nullable Integer allowedAuthenticationType;
/**
* @return A value indicating the application consistent frequency.
*
*/
private @Nullable Integer applicationConsistentSnapshotFrequencyInHours;
/**
* @return A value indicating whether compression has to be enabled.
*
*/
private @Nullable String compression;
/**
* @return A value indicating whether IR is online.
*
*/
private @Nullable String initialReplicationMethod;
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'HyperVReplicaBasePolicyDetails'.
*
*/
private String instanceType;
/**
* @return A value indicating the offline IR export path.
*
*/
private @Nullable String offlineReplicationExportPath;
/**
* @return A value indicating the offline IR import path.
*
*/
private @Nullable String offlineReplicationImportPath;
/**
* @return A value indicating the online IR start time.
*
*/
private @Nullable String onlineReplicationStartTime;
/**
* @return A value indicating the number of recovery points.
*
*/
private @Nullable Integer recoveryPoints;
/**
* @return A value indicating whether the VM has to be auto deleted. Supported Values: String.Empty, None, OnRecoveryCloud.
*
*/
private @Nullable String replicaDeletionOption;
/**
* @return A value indicating the recovery HTTPS port.
*
*/
private @Nullable Integer replicationPort;
private HyperVReplicaBasePolicyDetailsResponse() {}
/**
* @return A value indicating the authentication type.
*
*/
public Optional allowedAuthenticationType() {
return Optional.ofNullable(this.allowedAuthenticationType);
}
/**
* @return A value indicating the application consistent frequency.
*
*/
public Optional applicationConsistentSnapshotFrequencyInHours() {
return Optional.ofNullable(this.applicationConsistentSnapshotFrequencyInHours);
}
/**
* @return A value indicating whether compression has to be enabled.
*
*/
public Optional compression() {
return Optional.ofNullable(this.compression);
}
/**
* @return A value indicating whether IR is online.
*
*/
public Optional initialReplicationMethod() {
return Optional.ofNullable(this.initialReplicationMethod);
}
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'HyperVReplicaBasePolicyDetails'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return A value indicating the offline IR export path.
*
*/
public Optional offlineReplicationExportPath() {
return Optional.ofNullable(this.offlineReplicationExportPath);
}
/**
* @return A value indicating the offline IR import path.
*
*/
public Optional offlineReplicationImportPath() {
return Optional.ofNullable(this.offlineReplicationImportPath);
}
/**
* @return A value indicating the online IR start time.
*
*/
public Optional onlineReplicationStartTime() {
return Optional.ofNullable(this.onlineReplicationStartTime);
}
/**
* @return A value indicating the number of recovery points.
*
*/
public Optional recoveryPoints() {
return Optional.ofNullable(this.recoveryPoints);
}
/**
* @return A value indicating whether the VM has to be auto deleted. Supported Values: String.Empty, None, OnRecoveryCloud.
*
*/
public Optional replicaDeletionOption() {
return Optional.ofNullable(this.replicaDeletionOption);
}
/**
* @return A value indicating the recovery HTTPS port.
*
*/
public Optional replicationPort() {
return Optional.ofNullable(this.replicationPort);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(HyperVReplicaBasePolicyDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer allowedAuthenticationType;
private @Nullable Integer applicationConsistentSnapshotFrequencyInHours;
private @Nullable String compression;
private @Nullable String initialReplicationMethod;
private String instanceType;
private @Nullable String offlineReplicationExportPath;
private @Nullable String offlineReplicationImportPath;
private @Nullable String onlineReplicationStartTime;
private @Nullable Integer recoveryPoints;
private @Nullable String replicaDeletionOption;
private @Nullable Integer replicationPort;
public Builder() {}
public Builder(HyperVReplicaBasePolicyDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.allowedAuthenticationType = defaults.allowedAuthenticationType;
this.applicationConsistentSnapshotFrequencyInHours = defaults.applicationConsistentSnapshotFrequencyInHours;
this.compression = defaults.compression;
this.initialReplicationMethod = defaults.initialReplicationMethod;
this.instanceType = defaults.instanceType;
this.offlineReplicationExportPath = defaults.offlineReplicationExportPath;
this.offlineReplicationImportPath = defaults.offlineReplicationImportPath;
this.onlineReplicationStartTime = defaults.onlineReplicationStartTime;
this.recoveryPoints = defaults.recoveryPoints;
this.replicaDeletionOption = defaults.replicaDeletionOption;
this.replicationPort = defaults.replicationPort;
}
@CustomType.Setter
public Builder allowedAuthenticationType(@Nullable Integer allowedAuthenticationType) {
this.allowedAuthenticationType = allowedAuthenticationType;
return this;
}
@CustomType.Setter
public Builder applicationConsistentSnapshotFrequencyInHours(@Nullable Integer applicationConsistentSnapshotFrequencyInHours) {
this.applicationConsistentSnapshotFrequencyInHours = applicationConsistentSnapshotFrequencyInHours;
return this;
}
@CustomType.Setter
public Builder compression(@Nullable String compression) {
this.compression = compression;
return this;
}
@CustomType.Setter
public Builder initialReplicationMethod(@Nullable String initialReplicationMethod) {
this.initialReplicationMethod = initialReplicationMethod;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("HyperVReplicaBasePolicyDetailsResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder offlineReplicationExportPath(@Nullable String offlineReplicationExportPath) {
this.offlineReplicationExportPath = offlineReplicationExportPath;
return this;
}
@CustomType.Setter
public Builder offlineReplicationImportPath(@Nullable String offlineReplicationImportPath) {
this.offlineReplicationImportPath = offlineReplicationImportPath;
return this;
}
@CustomType.Setter
public Builder onlineReplicationStartTime(@Nullable String onlineReplicationStartTime) {
this.onlineReplicationStartTime = onlineReplicationStartTime;
return this;
}
@CustomType.Setter
public Builder recoveryPoints(@Nullable Integer recoveryPoints) {
this.recoveryPoints = recoveryPoints;
return this;
}
@CustomType.Setter
public Builder replicaDeletionOption(@Nullable String replicaDeletionOption) {
this.replicaDeletionOption = replicaDeletionOption;
return this;
}
@CustomType.Setter
public Builder replicationPort(@Nullable Integer replicationPort) {
this.replicationPort = replicationPort;
return this;
}
public HyperVReplicaBasePolicyDetailsResponse build() {
final var _resultValue = new HyperVReplicaBasePolicyDetailsResponse();
_resultValue.allowedAuthenticationType = allowedAuthenticationType;
_resultValue.applicationConsistentSnapshotFrequencyInHours = applicationConsistentSnapshotFrequencyInHours;
_resultValue.compression = compression;
_resultValue.initialReplicationMethod = initialReplicationMethod;
_resultValue.instanceType = instanceType;
_resultValue.offlineReplicationExportPath = offlineReplicationExportPath;
_resultValue.offlineReplicationImportPath = offlineReplicationImportPath;
_resultValue.onlineReplicationStartTime = onlineReplicationStartTime;
_resultValue.recoveryPoints = recoveryPoints;
_resultValue.replicaDeletionOption = replicaDeletionOption;
_resultValue.replicationPort = replicationPort;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy