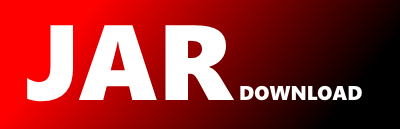
com.pulumi.azurenative.recoveryservices.outputs.VMwareCbtProtectedDiskDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VMwareCbtProtectedDiskDetailsResponse {
/**
* @return The disk capacity in bytes.
*
*/
private Double capacityInBytes;
/**
* @return The DiskEncryptionSet ARM Id.
*
*/
private String diskEncryptionSetId;
/**
* @return The disk id.
*
*/
private String diskId;
/**
* @return The disk name.
*
*/
private String diskName;
/**
* @return The disk path.
*
*/
private String diskPath;
/**
* @return The disk type.
*
*/
private @Nullable String diskType;
/**
* @return A value indicating whether the disk is the OS disk.
*
*/
private String isOSDisk;
/**
* @return The log storage account ARM Id.
*
*/
private String logStorageAccountId;
/**
* @return The key vault secret name of the log storage account.
*
*/
private String logStorageAccountSasSecretName;
/**
* @return The uri of the seed blob.
*
*/
private String seedBlobUri;
/**
* @return The ARM Id of the seed managed disk.
*
*/
private String seedManagedDiskId;
/**
* @return The uri of the target blob.
*
*/
private String targetBlobUri;
/**
* @return The name for the target managed disk.
*
*/
private @Nullable String targetDiskName;
/**
* @return The ARM Id of the target managed disk.
*
*/
private String targetManagedDiskId;
private VMwareCbtProtectedDiskDetailsResponse() {}
/**
* @return The disk capacity in bytes.
*
*/
public Double capacityInBytes() {
return this.capacityInBytes;
}
/**
* @return The DiskEncryptionSet ARM Id.
*
*/
public String diskEncryptionSetId() {
return this.diskEncryptionSetId;
}
/**
* @return The disk id.
*
*/
public String diskId() {
return this.diskId;
}
/**
* @return The disk name.
*
*/
public String diskName() {
return this.diskName;
}
/**
* @return The disk path.
*
*/
public String diskPath() {
return this.diskPath;
}
/**
* @return The disk type.
*
*/
public Optional diskType() {
return Optional.ofNullable(this.diskType);
}
/**
* @return A value indicating whether the disk is the OS disk.
*
*/
public String isOSDisk() {
return this.isOSDisk;
}
/**
* @return The log storage account ARM Id.
*
*/
public String logStorageAccountId() {
return this.logStorageAccountId;
}
/**
* @return The key vault secret name of the log storage account.
*
*/
public String logStorageAccountSasSecretName() {
return this.logStorageAccountSasSecretName;
}
/**
* @return The uri of the seed blob.
*
*/
public String seedBlobUri() {
return this.seedBlobUri;
}
/**
* @return The ARM Id of the seed managed disk.
*
*/
public String seedManagedDiskId() {
return this.seedManagedDiskId;
}
/**
* @return The uri of the target blob.
*
*/
public String targetBlobUri() {
return this.targetBlobUri;
}
/**
* @return The name for the target managed disk.
*
*/
public Optional targetDiskName() {
return Optional.ofNullable(this.targetDiskName);
}
/**
* @return The ARM Id of the target managed disk.
*
*/
public String targetManagedDiskId() {
return this.targetManagedDiskId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VMwareCbtProtectedDiskDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double capacityInBytes;
private String diskEncryptionSetId;
private String diskId;
private String diskName;
private String diskPath;
private @Nullable String diskType;
private String isOSDisk;
private String logStorageAccountId;
private String logStorageAccountSasSecretName;
private String seedBlobUri;
private String seedManagedDiskId;
private String targetBlobUri;
private @Nullable String targetDiskName;
private String targetManagedDiskId;
public Builder() {}
public Builder(VMwareCbtProtectedDiskDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.capacityInBytes = defaults.capacityInBytes;
this.diskEncryptionSetId = defaults.diskEncryptionSetId;
this.diskId = defaults.diskId;
this.diskName = defaults.diskName;
this.diskPath = defaults.diskPath;
this.diskType = defaults.diskType;
this.isOSDisk = defaults.isOSDisk;
this.logStorageAccountId = defaults.logStorageAccountId;
this.logStorageAccountSasSecretName = defaults.logStorageAccountSasSecretName;
this.seedBlobUri = defaults.seedBlobUri;
this.seedManagedDiskId = defaults.seedManagedDiskId;
this.targetBlobUri = defaults.targetBlobUri;
this.targetDiskName = defaults.targetDiskName;
this.targetManagedDiskId = defaults.targetManagedDiskId;
}
@CustomType.Setter
public Builder capacityInBytes(Double capacityInBytes) {
if (capacityInBytes == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "capacityInBytes");
}
this.capacityInBytes = capacityInBytes;
return this;
}
@CustomType.Setter
public Builder diskEncryptionSetId(String diskEncryptionSetId) {
if (diskEncryptionSetId == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "diskEncryptionSetId");
}
this.diskEncryptionSetId = diskEncryptionSetId;
return this;
}
@CustomType.Setter
public Builder diskId(String diskId) {
if (diskId == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "diskId");
}
this.diskId = diskId;
return this;
}
@CustomType.Setter
public Builder diskName(String diskName) {
if (diskName == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "diskName");
}
this.diskName = diskName;
return this;
}
@CustomType.Setter
public Builder diskPath(String diskPath) {
if (diskPath == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "diskPath");
}
this.diskPath = diskPath;
return this;
}
@CustomType.Setter
public Builder diskType(@Nullable String diskType) {
this.diskType = diskType;
return this;
}
@CustomType.Setter
public Builder isOSDisk(String isOSDisk) {
if (isOSDisk == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "isOSDisk");
}
this.isOSDisk = isOSDisk;
return this;
}
@CustomType.Setter
public Builder logStorageAccountId(String logStorageAccountId) {
if (logStorageAccountId == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "logStorageAccountId");
}
this.logStorageAccountId = logStorageAccountId;
return this;
}
@CustomType.Setter
public Builder logStorageAccountSasSecretName(String logStorageAccountSasSecretName) {
if (logStorageAccountSasSecretName == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "logStorageAccountSasSecretName");
}
this.logStorageAccountSasSecretName = logStorageAccountSasSecretName;
return this;
}
@CustomType.Setter
public Builder seedBlobUri(String seedBlobUri) {
if (seedBlobUri == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "seedBlobUri");
}
this.seedBlobUri = seedBlobUri;
return this;
}
@CustomType.Setter
public Builder seedManagedDiskId(String seedManagedDiskId) {
if (seedManagedDiskId == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "seedManagedDiskId");
}
this.seedManagedDiskId = seedManagedDiskId;
return this;
}
@CustomType.Setter
public Builder targetBlobUri(String targetBlobUri) {
if (targetBlobUri == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "targetBlobUri");
}
this.targetBlobUri = targetBlobUri;
return this;
}
@CustomType.Setter
public Builder targetDiskName(@Nullable String targetDiskName) {
this.targetDiskName = targetDiskName;
return this;
}
@CustomType.Setter
public Builder targetManagedDiskId(String targetManagedDiskId) {
if (targetManagedDiskId == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectedDiskDetailsResponse", "targetManagedDiskId");
}
this.targetManagedDiskId = targetManagedDiskId;
return this;
}
public VMwareCbtProtectedDiskDetailsResponse build() {
final var _resultValue = new VMwareCbtProtectedDiskDetailsResponse();
_resultValue.capacityInBytes = capacityInBytes;
_resultValue.diskEncryptionSetId = diskEncryptionSetId;
_resultValue.diskId = diskId;
_resultValue.diskName = diskName;
_resultValue.diskPath = diskPath;
_resultValue.diskType = diskType;
_resultValue.isOSDisk = isOSDisk;
_resultValue.logStorageAccountId = logStorageAccountId;
_resultValue.logStorageAccountSasSecretName = logStorageAccountSasSecretName;
_resultValue.seedBlobUri = seedBlobUri;
_resultValue.seedManagedDiskId = seedManagedDiskId;
_resultValue.targetBlobUri = targetBlobUri;
_resultValue.targetDiskName = targetDiskName;
_resultValue.targetManagedDiskId = targetManagedDiskId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy