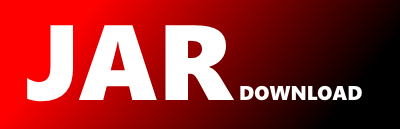
com.pulumi.azurenative.resources.AzurePowerShellScriptArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.resources;
import com.pulumi.azurenative.resources.enums.CleanupOptions;
import com.pulumi.azurenative.resources.inputs.ContainerConfigurationArgs;
import com.pulumi.azurenative.resources.inputs.EnvironmentVariableArgs;
import com.pulumi.azurenative.resources.inputs.ManagedServiceIdentityArgs;
import com.pulumi.azurenative.resources.inputs.StorageAccountConfigurationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AzurePowerShellScriptArgs extends com.pulumi.resources.ResourceArgs {
public static final AzurePowerShellScriptArgs Empty = new AzurePowerShellScriptArgs();
/**
* Command line arguments to pass to the script. Arguments are separated by spaces. ex: -Name blue* -Location 'West US 2'
*
*/
@Import(name="arguments")
private @Nullable Output arguments;
/**
* @return Command line arguments to pass to the script. Arguments are separated by spaces. ex: -Name blue* -Location 'West US 2'
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy