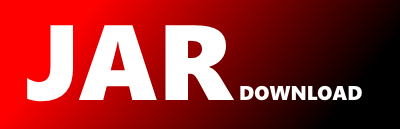
com.pulumi.azurenative.resources.inputs.DenySettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.resources.inputs;
import com.pulumi.azurenative.resources.enums.DenySettingsMode;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Defines how resources deployed by the deployment stack are locked.
*
*/
public final class DenySettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final DenySettingsArgs Empty = new DenySettingsArgs();
/**
* DenySettings will be applied to child scopes.
*
*/
@Import(name="applyToChildScopes")
private @Nullable Output applyToChildScopes;
/**
* @return DenySettings will be applied to child scopes.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy