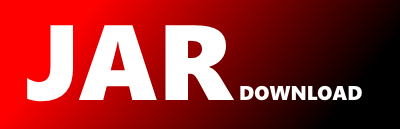
com.pulumi.azurenative.scvmm.VirtualMachineInstanceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.scvmm;
import com.pulumi.azurenative.scvmm.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.scvmm.inputs.HardwareProfileArgs;
import com.pulumi.azurenative.scvmm.inputs.InfrastructureProfileArgs;
import com.pulumi.azurenative.scvmm.inputs.NetworkProfileArgs;
import com.pulumi.azurenative.scvmm.inputs.OsProfileForVMInstanceArgs;
import com.pulumi.azurenative.scvmm.inputs.StorageProfileArgs;
import com.pulumi.azurenative.scvmm.inputs.VirtualMachineInstancePropertiesAvailabilitySetsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VirtualMachineInstanceArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualMachineInstanceArgs Empty = new VirtualMachineInstanceArgs();
/**
* Availability Sets in vm.
*
*/
@Import(name="availabilitySets")
private @Nullable Output> availabilitySets;
/**
* @return Availability Sets in vm.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy