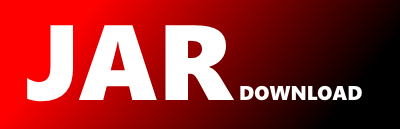
com.pulumi.azurenative.scvmm.outputs.VirtualDiskResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.scvmm.outputs;
import com.pulumi.azurenative.scvmm.outputs.StorageQoSPolicyDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VirtualDiskResponse {
/**
* @return Gets or sets the disk bus.
*
*/
private @Nullable Integer bus;
/**
* @return Gets or sets the disk bus type.
*
*/
private @Nullable String busType;
/**
* @return Gets or sets a value indicating diff disk.
*
*/
private @Nullable String createDiffDisk;
/**
* @return Gets or sets the disk id.
*
*/
private @Nullable String diskId;
/**
* @return Gets or sets the disk total size.
*
*/
private @Nullable Integer diskSizeGB;
/**
* @return Gets the display name of the virtual disk as shown in the vmmServer. This is the fallback label for a disk when the name is not set.
*
*/
private String displayName;
/**
* @return Gets or sets the disk lun.
*
*/
private @Nullable Integer lun;
/**
* @return Gets or sets the max disk size.
*
*/
private Integer maxDiskSizeGB;
/**
* @return Gets or sets the name of the disk.
*
*/
private @Nullable String name;
/**
* @return The QoS policy for the disk.
*
*/
private @Nullable StorageQoSPolicyDetailsResponse storageQoSPolicy;
/**
* @return Gets or sets the disk id in the template.
*
*/
private @Nullable String templateDiskId;
/**
* @return Gets the disk vhd format type.
*
*/
private String vhdFormatType;
/**
* @return Gets or sets the disk vhd type.
*
*/
private @Nullable String vhdType;
/**
* @return Gets or sets the disk volume type.
*
*/
private String volumeType;
private VirtualDiskResponse() {}
/**
* @return Gets or sets the disk bus.
*
*/
public Optional bus() {
return Optional.ofNullable(this.bus);
}
/**
* @return Gets or sets the disk bus type.
*
*/
public Optional busType() {
return Optional.ofNullable(this.busType);
}
/**
* @return Gets or sets a value indicating diff disk.
*
*/
public Optional createDiffDisk() {
return Optional.ofNullable(this.createDiffDisk);
}
/**
* @return Gets or sets the disk id.
*
*/
public Optional diskId() {
return Optional.ofNullable(this.diskId);
}
/**
* @return Gets or sets the disk total size.
*
*/
public Optional diskSizeGB() {
return Optional.ofNullable(this.diskSizeGB);
}
/**
* @return Gets the display name of the virtual disk as shown in the vmmServer. This is the fallback label for a disk when the name is not set.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Gets or sets the disk lun.
*
*/
public Optional lun() {
return Optional.ofNullable(this.lun);
}
/**
* @return Gets or sets the max disk size.
*
*/
public Integer maxDiskSizeGB() {
return this.maxDiskSizeGB;
}
/**
* @return Gets or sets the name of the disk.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The QoS policy for the disk.
*
*/
public Optional storageQoSPolicy() {
return Optional.ofNullable(this.storageQoSPolicy);
}
/**
* @return Gets or sets the disk id in the template.
*
*/
public Optional templateDiskId() {
return Optional.ofNullable(this.templateDiskId);
}
/**
* @return Gets the disk vhd format type.
*
*/
public String vhdFormatType() {
return this.vhdFormatType;
}
/**
* @return Gets or sets the disk vhd type.
*
*/
public Optional vhdType() {
return Optional.ofNullable(this.vhdType);
}
/**
* @return Gets or sets the disk volume type.
*
*/
public String volumeType() {
return this.volumeType;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VirtualDiskResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer bus;
private @Nullable String busType;
private @Nullable String createDiffDisk;
private @Nullable String diskId;
private @Nullable Integer diskSizeGB;
private String displayName;
private @Nullable Integer lun;
private Integer maxDiskSizeGB;
private @Nullable String name;
private @Nullable StorageQoSPolicyDetailsResponse storageQoSPolicy;
private @Nullable String templateDiskId;
private String vhdFormatType;
private @Nullable String vhdType;
private String volumeType;
public Builder() {}
public Builder(VirtualDiskResponse defaults) {
Objects.requireNonNull(defaults);
this.bus = defaults.bus;
this.busType = defaults.busType;
this.createDiffDisk = defaults.createDiffDisk;
this.diskId = defaults.diskId;
this.diskSizeGB = defaults.diskSizeGB;
this.displayName = defaults.displayName;
this.lun = defaults.lun;
this.maxDiskSizeGB = defaults.maxDiskSizeGB;
this.name = defaults.name;
this.storageQoSPolicy = defaults.storageQoSPolicy;
this.templateDiskId = defaults.templateDiskId;
this.vhdFormatType = defaults.vhdFormatType;
this.vhdType = defaults.vhdType;
this.volumeType = defaults.volumeType;
}
@CustomType.Setter
public Builder bus(@Nullable Integer bus) {
this.bus = bus;
return this;
}
@CustomType.Setter
public Builder busType(@Nullable String busType) {
this.busType = busType;
return this;
}
@CustomType.Setter
public Builder createDiffDisk(@Nullable String createDiffDisk) {
this.createDiffDisk = createDiffDisk;
return this;
}
@CustomType.Setter
public Builder diskId(@Nullable String diskId) {
this.diskId = diskId;
return this;
}
@CustomType.Setter
public Builder diskSizeGB(@Nullable Integer diskSizeGB) {
this.diskSizeGB = diskSizeGB;
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("VirtualDiskResponse", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder lun(@Nullable Integer lun) {
this.lun = lun;
return this;
}
@CustomType.Setter
public Builder maxDiskSizeGB(Integer maxDiskSizeGB) {
if (maxDiskSizeGB == null) {
throw new MissingRequiredPropertyException("VirtualDiskResponse", "maxDiskSizeGB");
}
this.maxDiskSizeGB = maxDiskSizeGB;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder storageQoSPolicy(@Nullable StorageQoSPolicyDetailsResponse storageQoSPolicy) {
this.storageQoSPolicy = storageQoSPolicy;
return this;
}
@CustomType.Setter
public Builder templateDiskId(@Nullable String templateDiskId) {
this.templateDiskId = templateDiskId;
return this;
}
@CustomType.Setter
public Builder vhdFormatType(String vhdFormatType) {
if (vhdFormatType == null) {
throw new MissingRequiredPropertyException("VirtualDiskResponse", "vhdFormatType");
}
this.vhdFormatType = vhdFormatType;
return this;
}
@CustomType.Setter
public Builder vhdType(@Nullable String vhdType) {
this.vhdType = vhdType;
return this;
}
@CustomType.Setter
public Builder volumeType(String volumeType) {
if (volumeType == null) {
throw new MissingRequiredPropertyException("VirtualDiskResponse", "volumeType");
}
this.volumeType = volumeType;
return this;
}
public VirtualDiskResponse build() {
final var _resultValue = new VirtualDiskResponse();
_resultValue.bus = bus;
_resultValue.busType = busType;
_resultValue.createDiffDisk = createDiffDisk;
_resultValue.diskId = diskId;
_resultValue.diskSizeGB = diskSizeGB;
_resultValue.displayName = displayName;
_resultValue.lun = lun;
_resultValue.maxDiskSizeGB = maxDiskSizeGB;
_resultValue.name = name;
_resultValue.storageQoSPolicy = storageQoSPolicy;
_resultValue.templateDiskId = templateDiskId;
_resultValue.vhdFormatType = vhdFormatType;
_resultValue.vhdType = vhdType;
_resultValue.volumeType = volumeType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy