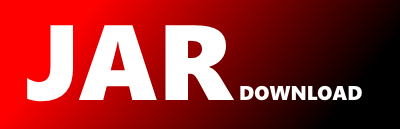
com.pulumi.azurenative.securityinsights.ScheduledAlertRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights;
import com.pulumi.azurenative.securityinsights.enums.AlertSeverity;
import com.pulumi.azurenative.securityinsights.enums.AttackTactic;
import com.pulumi.azurenative.securityinsights.enums.TriggerOperator;
import com.pulumi.azurenative.securityinsights.inputs.AlertDetailsOverrideArgs;
import com.pulumi.azurenative.securityinsights.inputs.EntityMappingArgs;
import com.pulumi.azurenative.securityinsights.inputs.EventGroupingSettingsArgs;
import com.pulumi.azurenative.securityinsights.inputs.IncidentConfigurationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ScheduledAlertRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final ScheduledAlertRuleArgs Empty = new ScheduledAlertRuleArgs();
/**
* The alert details override settings
*
*/
@Import(name="alertDetailsOverride")
private @Nullable Output alertDetailsOverride;
/**
* @return The alert details override settings
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy