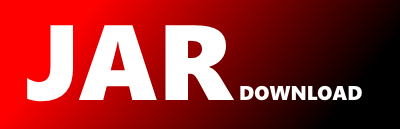
com.pulumi.azurenative.sql.outputs.GetDatabaseResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql.outputs;
import com.pulumi.azurenative.sql.outputs.DatabaseIdentityResponse;
import com.pulumi.azurenative.sql.outputs.SkuResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDatabaseResult {
/**
* @return Time in minutes after which database is automatically paused. A value of -1 means that automatic pause is disabled
*
*/
private @Nullable Integer autoPauseDelay;
/**
* @return Collation of the metadata catalog.
*
*/
private @Nullable String catalogCollation;
/**
* @return The collation of the database.
*
*/
private @Nullable String collation;
/**
* @return The creation date of the database (ISO8601 format).
*
*/
private String creationDate;
/**
* @return The storage account type used to store backups for this database.
*
*/
private String currentBackupStorageRedundancy;
/**
* @return The current service level objective name of the database.
*
*/
private String currentServiceObjectiveName;
/**
* @return The name and tier of the SKU.
*
*/
private SkuResponse currentSku;
/**
* @return The ID of the database.
*
*/
private String databaseId;
/**
* @return The default secondary region for this database.
*
*/
private String defaultSecondaryLocation;
/**
* @return This records the earliest start date and time that restore is available for this database (ISO8601 format).
*
*/
private String earliestRestoreDate;
/**
* @return The resource identifier of the elastic pool containing this database.
*
*/
private @Nullable String elasticPoolId;
/**
* @return Failover Group resource identifier that this database belongs to.
*
*/
private String failoverGroupId;
/**
* @return The Client id used for cross tenant per database CMK scenario
*
*/
private @Nullable String federatedClientId;
/**
* @return The number of secondary replicas associated with the database that are used to provide high availability. Not applicable to a Hyperscale database within an elastic pool.
*
*/
private @Nullable Integer highAvailabilityReplicaCount;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return The Azure Active Directory identity of the database.
*
*/
private @Nullable DatabaseIdentityResponse identity;
/**
* @return Infra encryption is enabled for this database.
*
*/
private Boolean isInfraEncryptionEnabled;
/**
* @return Whether or not this database is a ledger database, which means all tables in the database are ledger tables. Note: the value of this property cannot be changed after the database has been created.
*
*/
private @Nullable Boolean isLedgerOn;
/**
* @return Kind of database. This is metadata used for the Azure portal experience.
*
*/
private String kind;
/**
* @return The license type to apply for this database. `LicenseIncluded` if you need a license, or `BasePrice` if you have a license and are eligible for the Azure Hybrid Benefit.
*
*/
private @Nullable String licenseType;
/**
* @return Resource location.
*
*/
private String location;
/**
* @return Maintenance configuration id assigned to the database. This configuration defines the period when the maintenance updates will occur.
*
*/
private @Nullable String maintenanceConfigurationId;
/**
* @return Resource that manages the database.
*
*/
private String managedBy;
/**
* @return The max log size for this database.
*
*/
private Double maxLogSizeBytes;
/**
* @return The max size of the database expressed in bytes.
*
*/
private @Nullable Double maxSizeBytes;
/**
* @return Minimal capacity that database will always have allocated, if not paused
*
*/
private @Nullable Double minCapacity;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return The date when database was paused by user configuration or action(ISO8601 format). Null if the database is ready.
*
*/
private String pausedDate;
/**
* @return The state of read-only routing. If enabled, connections that have application intent set to readonly in their connection string may be routed to a readonly secondary replica in the same region. Not applicable to a Hyperscale database within an elastic pool.
*
*/
private @Nullable String readScale;
/**
* @return The storage account type to be used to store backups for this database.
*
*/
private @Nullable String requestedBackupStorageRedundancy;
/**
* @return The requested service level objective name of the database.
*
*/
private String requestedServiceObjectiveName;
/**
* @return The date when database was resumed by user action or database login (ISO8601 format). Null if the database is paused.
*
*/
private String resumedDate;
/**
* @return The secondary type of the database if it is a secondary. Valid values are Geo and Named.
*
*/
private @Nullable String secondaryType;
/**
* @return The database SKU.
*
* The list of SKUs may vary by region and support offer. To determine the SKUs (including the SKU name, tier/edition, family, and capacity) that are available to your subscription in an Azure region, use the `Capabilities_ListByLocation` REST API or one of the following commands:
*
*/
private @Nullable SkuResponse sku;
/**
* @return The status of the database.
*
*/
private String status;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return Whether or not this database is zone redundant, which means the replicas of this database will be spread across multiple availability zones.
*
*/
private @Nullable Boolean zoneRedundant;
private GetDatabaseResult() {}
/**
* @return Time in minutes after which database is automatically paused. A value of -1 means that automatic pause is disabled
*
*/
public Optional autoPauseDelay() {
return Optional.ofNullable(this.autoPauseDelay);
}
/**
* @return Collation of the metadata catalog.
*
*/
public Optional catalogCollation() {
return Optional.ofNullable(this.catalogCollation);
}
/**
* @return The collation of the database.
*
*/
public Optional collation() {
return Optional.ofNullable(this.collation);
}
/**
* @return The creation date of the database (ISO8601 format).
*
*/
public String creationDate() {
return this.creationDate;
}
/**
* @return The storage account type used to store backups for this database.
*
*/
public String currentBackupStorageRedundancy() {
return this.currentBackupStorageRedundancy;
}
/**
* @return The current service level objective name of the database.
*
*/
public String currentServiceObjectiveName() {
return this.currentServiceObjectiveName;
}
/**
* @return The name and tier of the SKU.
*
*/
public SkuResponse currentSku() {
return this.currentSku;
}
/**
* @return The ID of the database.
*
*/
public String databaseId() {
return this.databaseId;
}
/**
* @return The default secondary region for this database.
*
*/
public String defaultSecondaryLocation() {
return this.defaultSecondaryLocation;
}
/**
* @return This records the earliest start date and time that restore is available for this database (ISO8601 format).
*
*/
public String earliestRestoreDate() {
return this.earliestRestoreDate;
}
/**
* @return The resource identifier of the elastic pool containing this database.
*
*/
public Optional elasticPoolId() {
return Optional.ofNullable(this.elasticPoolId);
}
/**
* @return Failover Group resource identifier that this database belongs to.
*
*/
public String failoverGroupId() {
return this.failoverGroupId;
}
/**
* @return The Client id used for cross tenant per database CMK scenario
*
*/
public Optional federatedClientId() {
return Optional.ofNullable(this.federatedClientId);
}
/**
* @return The number of secondary replicas associated with the database that are used to provide high availability. Not applicable to a Hyperscale database within an elastic pool.
*
*/
public Optional highAvailabilityReplicaCount() {
return Optional.ofNullable(this.highAvailabilityReplicaCount);
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return The Azure Active Directory identity of the database.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return Infra encryption is enabled for this database.
*
*/
public Boolean isInfraEncryptionEnabled() {
return this.isInfraEncryptionEnabled;
}
/**
* @return Whether or not this database is a ledger database, which means all tables in the database are ledger tables. Note: the value of this property cannot be changed after the database has been created.
*
*/
public Optional isLedgerOn() {
return Optional.ofNullable(this.isLedgerOn);
}
/**
* @return Kind of database. This is metadata used for the Azure portal experience.
*
*/
public String kind() {
return this.kind;
}
/**
* @return The license type to apply for this database. `LicenseIncluded` if you need a license, or `BasePrice` if you have a license and are eligible for the Azure Hybrid Benefit.
*
*/
public Optional licenseType() {
return Optional.ofNullable(this.licenseType);
}
/**
* @return Resource location.
*
*/
public String location() {
return this.location;
}
/**
* @return Maintenance configuration id assigned to the database. This configuration defines the period when the maintenance updates will occur.
*
*/
public Optional maintenanceConfigurationId() {
return Optional.ofNullable(this.maintenanceConfigurationId);
}
/**
* @return Resource that manages the database.
*
*/
public String managedBy() {
return this.managedBy;
}
/**
* @return The max log size for this database.
*
*/
public Double maxLogSizeBytes() {
return this.maxLogSizeBytes;
}
/**
* @return The max size of the database expressed in bytes.
*
*/
public Optional maxSizeBytes() {
return Optional.ofNullable(this.maxSizeBytes);
}
/**
* @return Minimal capacity that database will always have allocated, if not paused
*
*/
public Optional minCapacity() {
return Optional.ofNullable(this.minCapacity);
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The date when database was paused by user configuration or action(ISO8601 format). Null if the database is ready.
*
*/
public String pausedDate() {
return this.pausedDate;
}
/**
* @return The state of read-only routing. If enabled, connections that have application intent set to readonly in their connection string may be routed to a readonly secondary replica in the same region. Not applicable to a Hyperscale database within an elastic pool.
*
*/
public Optional readScale() {
return Optional.ofNullable(this.readScale);
}
/**
* @return The storage account type to be used to store backups for this database.
*
*/
public Optional requestedBackupStorageRedundancy() {
return Optional.ofNullable(this.requestedBackupStorageRedundancy);
}
/**
* @return The requested service level objective name of the database.
*
*/
public String requestedServiceObjectiveName() {
return this.requestedServiceObjectiveName;
}
/**
* @return The date when database was resumed by user action or database login (ISO8601 format). Null if the database is paused.
*
*/
public String resumedDate() {
return this.resumedDate;
}
/**
* @return The secondary type of the database if it is a secondary. Valid values are Geo and Named.
*
*/
public Optional secondaryType() {
return Optional.ofNullable(this.secondaryType);
}
/**
* @return The database SKU.
*
* The list of SKUs may vary by region and support offer. To determine the SKUs (including the SKU name, tier/edition, family, and capacity) that are available to your subscription in an Azure region, use the `Capabilities_ListByLocation` REST API or one of the following commands:
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return The status of the database.
*
*/
public String status() {
return this.status;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return Whether or not this database is zone redundant, which means the replicas of this database will be spread across multiple availability zones.
*
*/
public Optional zoneRedundant() {
return Optional.ofNullable(this.zoneRedundant);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDatabaseResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer autoPauseDelay;
private @Nullable String catalogCollation;
private @Nullable String collation;
private String creationDate;
private String currentBackupStorageRedundancy;
private String currentServiceObjectiveName;
private SkuResponse currentSku;
private String databaseId;
private String defaultSecondaryLocation;
private String earliestRestoreDate;
private @Nullable String elasticPoolId;
private String failoverGroupId;
private @Nullable String federatedClientId;
private @Nullable Integer highAvailabilityReplicaCount;
private String id;
private @Nullable DatabaseIdentityResponse identity;
private Boolean isInfraEncryptionEnabled;
private @Nullable Boolean isLedgerOn;
private String kind;
private @Nullable String licenseType;
private String location;
private @Nullable String maintenanceConfigurationId;
private String managedBy;
private Double maxLogSizeBytes;
private @Nullable Double maxSizeBytes;
private @Nullable Double minCapacity;
private String name;
private String pausedDate;
private @Nullable String readScale;
private @Nullable String requestedBackupStorageRedundancy;
private String requestedServiceObjectiveName;
private String resumedDate;
private @Nullable String secondaryType;
private @Nullable SkuResponse sku;
private String status;
private @Nullable Map tags;
private String type;
private @Nullable Boolean zoneRedundant;
public Builder() {}
public Builder(GetDatabaseResult defaults) {
Objects.requireNonNull(defaults);
this.autoPauseDelay = defaults.autoPauseDelay;
this.catalogCollation = defaults.catalogCollation;
this.collation = defaults.collation;
this.creationDate = defaults.creationDate;
this.currentBackupStorageRedundancy = defaults.currentBackupStorageRedundancy;
this.currentServiceObjectiveName = defaults.currentServiceObjectiveName;
this.currentSku = defaults.currentSku;
this.databaseId = defaults.databaseId;
this.defaultSecondaryLocation = defaults.defaultSecondaryLocation;
this.earliestRestoreDate = defaults.earliestRestoreDate;
this.elasticPoolId = defaults.elasticPoolId;
this.failoverGroupId = defaults.failoverGroupId;
this.federatedClientId = defaults.federatedClientId;
this.highAvailabilityReplicaCount = defaults.highAvailabilityReplicaCount;
this.id = defaults.id;
this.identity = defaults.identity;
this.isInfraEncryptionEnabled = defaults.isInfraEncryptionEnabled;
this.isLedgerOn = defaults.isLedgerOn;
this.kind = defaults.kind;
this.licenseType = defaults.licenseType;
this.location = defaults.location;
this.maintenanceConfigurationId = defaults.maintenanceConfigurationId;
this.managedBy = defaults.managedBy;
this.maxLogSizeBytes = defaults.maxLogSizeBytes;
this.maxSizeBytes = defaults.maxSizeBytes;
this.minCapacity = defaults.minCapacity;
this.name = defaults.name;
this.pausedDate = defaults.pausedDate;
this.readScale = defaults.readScale;
this.requestedBackupStorageRedundancy = defaults.requestedBackupStorageRedundancy;
this.requestedServiceObjectiveName = defaults.requestedServiceObjectiveName;
this.resumedDate = defaults.resumedDate;
this.secondaryType = defaults.secondaryType;
this.sku = defaults.sku;
this.status = defaults.status;
this.tags = defaults.tags;
this.type = defaults.type;
this.zoneRedundant = defaults.zoneRedundant;
}
@CustomType.Setter
public Builder autoPauseDelay(@Nullable Integer autoPauseDelay) {
this.autoPauseDelay = autoPauseDelay;
return this;
}
@CustomType.Setter
public Builder catalogCollation(@Nullable String catalogCollation) {
this.catalogCollation = catalogCollation;
return this;
}
@CustomType.Setter
public Builder collation(@Nullable String collation) {
this.collation = collation;
return this;
}
@CustomType.Setter
public Builder creationDate(String creationDate) {
if (creationDate == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "creationDate");
}
this.creationDate = creationDate;
return this;
}
@CustomType.Setter
public Builder currentBackupStorageRedundancy(String currentBackupStorageRedundancy) {
if (currentBackupStorageRedundancy == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "currentBackupStorageRedundancy");
}
this.currentBackupStorageRedundancy = currentBackupStorageRedundancy;
return this;
}
@CustomType.Setter
public Builder currentServiceObjectiveName(String currentServiceObjectiveName) {
if (currentServiceObjectiveName == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "currentServiceObjectiveName");
}
this.currentServiceObjectiveName = currentServiceObjectiveName;
return this;
}
@CustomType.Setter
public Builder currentSku(SkuResponse currentSku) {
if (currentSku == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "currentSku");
}
this.currentSku = currentSku;
return this;
}
@CustomType.Setter
public Builder databaseId(String databaseId) {
if (databaseId == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "databaseId");
}
this.databaseId = databaseId;
return this;
}
@CustomType.Setter
public Builder defaultSecondaryLocation(String defaultSecondaryLocation) {
if (defaultSecondaryLocation == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "defaultSecondaryLocation");
}
this.defaultSecondaryLocation = defaultSecondaryLocation;
return this;
}
@CustomType.Setter
public Builder earliestRestoreDate(String earliestRestoreDate) {
if (earliestRestoreDate == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "earliestRestoreDate");
}
this.earliestRestoreDate = earliestRestoreDate;
return this;
}
@CustomType.Setter
public Builder elasticPoolId(@Nullable String elasticPoolId) {
this.elasticPoolId = elasticPoolId;
return this;
}
@CustomType.Setter
public Builder failoverGroupId(String failoverGroupId) {
if (failoverGroupId == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "failoverGroupId");
}
this.failoverGroupId = failoverGroupId;
return this;
}
@CustomType.Setter
public Builder federatedClientId(@Nullable String federatedClientId) {
this.federatedClientId = federatedClientId;
return this;
}
@CustomType.Setter
public Builder highAvailabilityReplicaCount(@Nullable Integer highAvailabilityReplicaCount) {
this.highAvailabilityReplicaCount = highAvailabilityReplicaCount;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable DatabaseIdentityResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder isInfraEncryptionEnabled(Boolean isInfraEncryptionEnabled) {
if (isInfraEncryptionEnabled == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "isInfraEncryptionEnabled");
}
this.isInfraEncryptionEnabled = isInfraEncryptionEnabled;
return this;
}
@CustomType.Setter
public Builder isLedgerOn(@Nullable Boolean isLedgerOn) {
this.isLedgerOn = isLedgerOn;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder licenseType(@Nullable String licenseType) {
this.licenseType = licenseType;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder maintenanceConfigurationId(@Nullable String maintenanceConfigurationId) {
this.maintenanceConfigurationId = maintenanceConfigurationId;
return this;
}
@CustomType.Setter
public Builder managedBy(String managedBy) {
if (managedBy == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "managedBy");
}
this.managedBy = managedBy;
return this;
}
@CustomType.Setter
public Builder maxLogSizeBytes(Double maxLogSizeBytes) {
if (maxLogSizeBytes == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "maxLogSizeBytes");
}
this.maxLogSizeBytes = maxLogSizeBytes;
return this;
}
@CustomType.Setter
public Builder maxSizeBytes(@Nullable Double maxSizeBytes) {
this.maxSizeBytes = maxSizeBytes;
return this;
}
@CustomType.Setter
public Builder minCapacity(@Nullable Double minCapacity) {
this.minCapacity = minCapacity;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder pausedDate(String pausedDate) {
if (pausedDate == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "pausedDate");
}
this.pausedDate = pausedDate;
return this;
}
@CustomType.Setter
public Builder readScale(@Nullable String readScale) {
this.readScale = readScale;
return this;
}
@CustomType.Setter
public Builder requestedBackupStorageRedundancy(@Nullable String requestedBackupStorageRedundancy) {
this.requestedBackupStorageRedundancy = requestedBackupStorageRedundancy;
return this;
}
@CustomType.Setter
public Builder requestedServiceObjectiveName(String requestedServiceObjectiveName) {
if (requestedServiceObjectiveName == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "requestedServiceObjectiveName");
}
this.requestedServiceObjectiveName = requestedServiceObjectiveName;
return this;
}
@CustomType.Setter
public Builder resumedDate(String resumedDate) {
if (resumedDate == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "resumedDate");
}
this.resumedDate = resumedDate;
return this;
}
@CustomType.Setter
public Builder secondaryType(@Nullable String secondaryType) {
this.secondaryType = secondaryType;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable SkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDatabaseResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder zoneRedundant(@Nullable Boolean zoneRedundant) {
this.zoneRedundant = zoneRedundant;
return this;
}
public GetDatabaseResult build() {
final var _resultValue = new GetDatabaseResult();
_resultValue.autoPauseDelay = autoPauseDelay;
_resultValue.catalogCollation = catalogCollation;
_resultValue.collation = collation;
_resultValue.creationDate = creationDate;
_resultValue.currentBackupStorageRedundancy = currentBackupStorageRedundancy;
_resultValue.currentServiceObjectiveName = currentServiceObjectiveName;
_resultValue.currentSku = currentSku;
_resultValue.databaseId = databaseId;
_resultValue.defaultSecondaryLocation = defaultSecondaryLocation;
_resultValue.earliestRestoreDate = earliestRestoreDate;
_resultValue.elasticPoolId = elasticPoolId;
_resultValue.failoverGroupId = failoverGroupId;
_resultValue.federatedClientId = federatedClientId;
_resultValue.highAvailabilityReplicaCount = highAvailabilityReplicaCount;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.isInfraEncryptionEnabled = isInfraEncryptionEnabled;
_resultValue.isLedgerOn = isLedgerOn;
_resultValue.kind = kind;
_resultValue.licenseType = licenseType;
_resultValue.location = location;
_resultValue.maintenanceConfigurationId = maintenanceConfigurationId;
_resultValue.managedBy = managedBy;
_resultValue.maxLogSizeBytes = maxLogSizeBytes;
_resultValue.maxSizeBytes = maxSizeBytes;
_resultValue.minCapacity = minCapacity;
_resultValue.name = name;
_resultValue.pausedDate = pausedDate;
_resultValue.readScale = readScale;
_resultValue.requestedBackupStorageRedundancy = requestedBackupStorageRedundancy;
_resultValue.requestedServiceObjectiveName = requestedServiceObjectiveName;
_resultValue.resumedDate = resumedDate;
_resultValue.secondaryType = secondaryType;
_resultValue.sku = sku;
_resultValue.status = status;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.zoneRedundant = zoneRedundant;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy