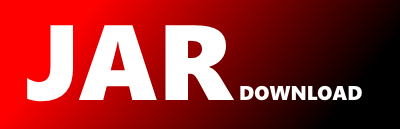
com.pulumi.azurenative.sqlvirtualmachine.outputs.AutoBackupSettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sqlvirtualmachine.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AutoBackupSettingsResponse {
/**
* @return Backup schedule type.
*
*/
private @Nullable String backupScheduleType;
/**
* @return Include or exclude system databases from auto backup.
*
*/
private @Nullable Boolean backupSystemDbs;
/**
* @return Days of the week for the backups when FullBackupFrequency is set to Weekly.
*
*/
private @Nullable List daysOfWeek;
/**
* @return Enable or disable autobackup on SQL virtual machine.
*
*/
private @Nullable Boolean enable;
/**
* @return Enable or disable encryption for backup on SQL virtual machine.
*
*/
private @Nullable Boolean enableEncryption;
/**
* @return Frequency of full backups. In both cases, full backups begin during the next scheduled time window.
*
*/
private @Nullable String fullBackupFrequency;
/**
* @return Start time of a given day during which full backups can take place. 0-23 hours.
*
*/
private @Nullable Integer fullBackupStartTime;
/**
* @return Duration of the time window of a given day during which full backups can take place. 1-23 hours.
*
*/
private @Nullable Integer fullBackupWindowHours;
/**
* @return Frequency of log backups. 5-60 minutes.
*
*/
private @Nullable Integer logBackupFrequency;
/**
* @return Retention period of backup: 1-90 days.
*
*/
private @Nullable Integer retentionPeriod;
/**
* @return Storage account url where backup will be taken to.
*
*/
private @Nullable String storageAccountUrl;
/**
* @return Storage container name where backup will be taken to.
*
*/
private @Nullable String storageContainerName;
private AutoBackupSettingsResponse() {}
/**
* @return Backup schedule type.
*
*/
public Optional backupScheduleType() {
return Optional.ofNullable(this.backupScheduleType);
}
/**
* @return Include or exclude system databases from auto backup.
*
*/
public Optional backupSystemDbs() {
return Optional.ofNullable(this.backupSystemDbs);
}
/**
* @return Days of the week for the backups when FullBackupFrequency is set to Weekly.
*
*/
public List daysOfWeek() {
return this.daysOfWeek == null ? List.of() : this.daysOfWeek;
}
/**
* @return Enable or disable autobackup on SQL virtual machine.
*
*/
public Optional enable() {
return Optional.ofNullable(this.enable);
}
/**
* @return Enable or disable encryption for backup on SQL virtual machine.
*
*/
public Optional enableEncryption() {
return Optional.ofNullable(this.enableEncryption);
}
/**
* @return Frequency of full backups. In both cases, full backups begin during the next scheduled time window.
*
*/
public Optional fullBackupFrequency() {
return Optional.ofNullable(this.fullBackupFrequency);
}
/**
* @return Start time of a given day during which full backups can take place. 0-23 hours.
*
*/
public Optional fullBackupStartTime() {
return Optional.ofNullable(this.fullBackupStartTime);
}
/**
* @return Duration of the time window of a given day during which full backups can take place. 1-23 hours.
*
*/
public Optional fullBackupWindowHours() {
return Optional.ofNullable(this.fullBackupWindowHours);
}
/**
* @return Frequency of log backups. 5-60 minutes.
*
*/
public Optional logBackupFrequency() {
return Optional.ofNullable(this.logBackupFrequency);
}
/**
* @return Retention period of backup: 1-90 days.
*
*/
public Optional retentionPeriod() {
return Optional.ofNullable(this.retentionPeriod);
}
/**
* @return Storage account url where backup will be taken to.
*
*/
public Optional storageAccountUrl() {
return Optional.ofNullable(this.storageAccountUrl);
}
/**
* @return Storage container name where backup will be taken to.
*
*/
public Optional storageContainerName() {
return Optional.ofNullable(this.storageContainerName);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AutoBackupSettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String backupScheduleType;
private @Nullable Boolean backupSystemDbs;
private @Nullable List daysOfWeek;
private @Nullable Boolean enable;
private @Nullable Boolean enableEncryption;
private @Nullable String fullBackupFrequency;
private @Nullable Integer fullBackupStartTime;
private @Nullable Integer fullBackupWindowHours;
private @Nullable Integer logBackupFrequency;
private @Nullable Integer retentionPeriod;
private @Nullable String storageAccountUrl;
private @Nullable String storageContainerName;
public Builder() {}
public Builder(AutoBackupSettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.backupScheduleType = defaults.backupScheduleType;
this.backupSystemDbs = defaults.backupSystemDbs;
this.daysOfWeek = defaults.daysOfWeek;
this.enable = defaults.enable;
this.enableEncryption = defaults.enableEncryption;
this.fullBackupFrequency = defaults.fullBackupFrequency;
this.fullBackupStartTime = defaults.fullBackupStartTime;
this.fullBackupWindowHours = defaults.fullBackupWindowHours;
this.logBackupFrequency = defaults.logBackupFrequency;
this.retentionPeriod = defaults.retentionPeriod;
this.storageAccountUrl = defaults.storageAccountUrl;
this.storageContainerName = defaults.storageContainerName;
}
@CustomType.Setter
public Builder backupScheduleType(@Nullable String backupScheduleType) {
this.backupScheduleType = backupScheduleType;
return this;
}
@CustomType.Setter
public Builder backupSystemDbs(@Nullable Boolean backupSystemDbs) {
this.backupSystemDbs = backupSystemDbs;
return this;
}
@CustomType.Setter
public Builder daysOfWeek(@Nullable List daysOfWeek) {
this.daysOfWeek = daysOfWeek;
return this;
}
public Builder daysOfWeek(String... daysOfWeek) {
return daysOfWeek(List.of(daysOfWeek));
}
@CustomType.Setter
public Builder enable(@Nullable Boolean enable) {
this.enable = enable;
return this;
}
@CustomType.Setter
public Builder enableEncryption(@Nullable Boolean enableEncryption) {
this.enableEncryption = enableEncryption;
return this;
}
@CustomType.Setter
public Builder fullBackupFrequency(@Nullable String fullBackupFrequency) {
this.fullBackupFrequency = fullBackupFrequency;
return this;
}
@CustomType.Setter
public Builder fullBackupStartTime(@Nullable Integer fullBackupStartTime) {
this.fullBackupStartTime = fullBackupStartTime;
return this;
}
@CustomType.Setter
public Builder fullBackupWindowHours(@Nullable Integer fullBackupWindowHours) {
this.fullBackupWindowHours = fullBackupWindowHours;
return this;
}
@CustomType.Setter
public Builder logBackupFrequency(@Nullable Integer logBackupFrequency) {
this.logBackupFrequency = logBackupFrequency;
return this;
}
@CustomType.Setter
public Builder retentionPeriod(@Nullable Integer retentionPeriod) {
this.retentionPeriod = retentionPeriod;
return this;
}
@CustomType.Setter
public Builder storageAccountUrl(@Nullable String storageAccountUrl) {
this.storageAccountUrl = storageAccountUrl;
return this;
}
@CustomType.Setter
public Builder storageContainerName(@Nullable String storageContainerName) {
this.storageContainerName = storageContainerName;
return this;
}
public AutoBackupSettingsResponse build() {
final var _resultValue = new AutoBackupSettingsResponse();
_resultValue.backupScheduleType = backupScheduleType;
_resultValue.backupSystemDbs = backupSystemDbs;
_resultValue.daysOfWeek = daysOfWeek;
_resultValue.enable = enable;
_resultValue.enableEncryption = enableEncryption;
_resultValue.fullBackupFrequency = fullBackupFrequency;
_resultValue.fullBackupStartTime = fullBackupStartTime;
_resultValue.fullBackupWindowHours = fullBackupWindowHours;
_resultValue.logBackupFrequency = logBackupFrequency;
_resultValue.retentionPeriod = retentionPeriod;
_resultValue.storageAccountUrl = storageAccountUrl;
_resultValue.storageContainerName = storageContainerName;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy