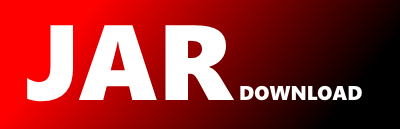
com.pulumi.azurenative.storage.StorageAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.storage.StorageAccountArgs;
import com.pulumi.azurenative.storage.outputs.AzureFilesIdentityBasedAuthenticationResponse;
import com.pulumi.azurenative.storage.outputs.BlobRestoreStatusResponse;
import com.pulumi.azurenative.storage.outputs.CustomDomainResponse;
import com.pulumi.azurenative.storage.outputs.EncryptionResponse;
import com.pulumi.azurenative.storage.outputs.EndpointsResponse;
import com.pulumi.azurenative.storage.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.storage.outputs.GeoReplicationStatsResponse;
import com.pulumi.azurenative.storage.outputs.IdentityResponse;
import com.pulumi.azurenative.storage.outputs.ImmutableStorageAccountResponse;
import com.pulumi.azurenative.storage.outputs.KeyCreationTimeResponse;
import com.pulumi.azurenative.storage.outputs.KeyPolicyResponse;
import com.pulumi.azurenative.storage.outputs.NetworkRuleSetResponse;
import com.pulumi.azurenative.storage.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.storage.outputs.RoutingPreferenceResponse;
import com.pulumi.azurenative.storage.outputs.SasPolicyResponse;
import com.pulumi.azurenative.storage.outputs.SkuResponse;
import com.pulumi.azurenative.storage.outputs.StorageAccountSkuConversionStatusResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The storage account.
* Azure REST API version: 2022-09-01. Prior API version in Azure Native 1.x: 2021-02-01.
*
* Other available API versions: 2015-05-01-preview, 2015-06-15, 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
* ## Example Usage
* ### NfsV3AccountCreate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.NetworkRuleSetArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .enableHttpsTrafficOnly(false)
* .enableNfsV3(true)
* .isHnsEnabled(true)
* .kind("BlockBlobStorage")
* .location("eastus")
* .networkRuleSet(NetworkRuleSetArgs.builder()
* .bypass("AzureServices")
* .defaultAction("Allow")
* .ipRules()
* .virtualNetworkRules(VirtualNetworkRuleArgs.builder()
* .virtualNetworkResourceId("/subscriptions/{subscription-id}/resourceGroups/res9101/providers/Microsoft.Network/virtualNetworks/net123/subnets/subnet12")
* .build())
* .build())
* .resourceGroupName("res9101")
* .sku(SkuArgs.builder()
* .name("Premium_LRS")
* .build())
* .build());
*
* }
* }
*
* }
*
* ### StorageAccountCreate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServicesArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServiceArgs;
* import com.pulumi.azurenative.storage.inputs.ExtendedLocationArgs;
* import com.pulumi.azurenative.storage.inputs.KeyPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.RoutingPreferenceArgs;
* import com.pulumi.azurenative.storage.inputs.SasPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .allowBlobPublicAccess(false)
* .allowSharedKeyAccess(true)
* .defaultToOAuthAuthentication(false)
* .encryption(EncryptionArgs.builder()
* .keySource("Microsoft.Storage")
* .requireInfrastructureEncryption(false)
* .services(EncryptionServicesArgs.builder()
* .blob(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .file(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .build())
* .build())
* .extendedLocation(ExtendedLocationArgs.builder()
* .name("losangeles001")
* .type("EdgeZone")
* .build())
* .isHnsEnabled(true)
* .isSftpEnabled(true)
* .keyPolicy(KeyPolicyArgs.builder()
* .keyExpirationPeriodInDays(20)
* .build())
* .kind("Storage")
* .location("eastus")
* .minimumTlsVersion("TLS1_2")
* .resourceGroupName("res9101")
* .routingPreference(RoutingPreferenceArgs.builder()
* .publishInternetEndpoints(true)
* .publishMicrosoftEndpoints(true)
* .routingChoice("MicrosoftRouting")
* .build())
* .sasPolicy(SasPolicyArgs.builder()
* .expirationAction("Log")
* .sasExpirationPeriod("1.15:59:59")
* .build())
* .sku(SkuArgs.builder()
* .name("Standard_GRS")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .build());
*
* }
* }
*
* }
*
* ### StorageAccountCreateAllowedCopyScopeToAAD
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServicesArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServiceArgs;
* import com.pulumi.azurenative.storage.inputs.KeyPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.RoutingPreferenceArgs;
* import com.pulumi.azurenative.storage.inputs.SasPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .allowBlobPublicAccess(false)
* .allowSharedKeyAccess(true)
* .allowedCopyScope("AAD")
* .encryption(EncryptionArgs.builder()
* .keySource("Microsoft.Storage")
* .requireInfrastructureEncryption(false)
* .services(EncryptionServicesArgs.builder()
* .blob(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .file(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .build())
* .build())
* .isHnsEnabled(true)
* .keyPolicy(KeyPolicyArgs.builder()
* .keyExpirationPeriodInDays(20)
* .build())
* .kind("Storage")
* .location("eastus")
* .minimumTlsVersion("TLS1_2")
* .resourceGroupName("res9101")
* .routingPreference(RoutingPreferenceArgs.builder()
* .publishInternetEndpoints(true)
* .publishMicrosoftEndpoints(true)
* .routingChoice("MicrosoftRouting")
* .build())
* .sasPolicy(SasPolicyArgs.builder()
* .expirationAction("Log")
* .sasExpirationPeriod("1.15:59:59")
* .build())
* .sku(SkuArgs.builder()
* .name("Standard_GRS")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .build());
*
* }
* }
*
* }
*
* ### StorageAccountCreateAllowedCopyScopeToPrivateLink
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServicesArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServiceArgs;
* import com.pulumi.azurenative.storage.inputs.KeyPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.RoutingPreferenceArgs;
* import com.pulumi.azurenative.storage.inputs.SasPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .allowBlobPublicAccess(false)
* .allowSharedKeyAccess(true)
* .allowedCopyScope("PrivateLink")
* .encryption(EncryptionArgs.builder()
* .keySource("Microsoft.Storage")
* .requireInfrastructureEncryption(false)
* .services(EncryptionServicesArgs.builder()
* .blob(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .file(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .build())
* .build())
* .isHnsEnabled(true)
* .keyPolicy(KeyPolicyArgs.builder()
* .keyExpirationPeriodInDays(20)
* .build())
* .kind("Storage")
* .location("eastus")
* .minimumTlsVersion("TLS1_2")
* .resourceGroupName("res9101")
* .routingPreference(RoutingPreferenceArgs.builder()
* .publishInternetEndpoints(true)
* .publishMicrosoftEndpoints(true)
* .routingChoice("MicrosoftRouting")
* .build())
* .sasPolicy(SasPolicyArgs.builder()
* .expirationAction("Log")
* .sasExpirationPeriod("1.15:59:59")
* .build())
* .sku(SkuArgs.builder()
* .name("Standard_GRS")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .build());
*
* }
* }
*
* }
*
* ### StorageAccountCreateDisallowPublicNetworkAccess
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServicesArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServiceArgs;
* import com.pulumi.azurenative.storage.inputs.ExtendedLocationArgs;
* import com.pulumi.azurenative.storage.inputs.KeyPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.RoutingPreferenceArgs;
* import com.pulumi.azurenative.storage.inputs.SasPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .allowBlobPublicAccess(false)
* .allowSharedKeyAccess(true)
* .encryption(EncryptionArgs.builder()
* .keySource("Microsoft.Storage")
* .requireInfrastructureEncryption(false)
* .services(EncryptionServicesArgs.builder()
* .blob(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .file(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .build())
* .build())
* .extendedLocation(ExtendedLocationArgs.builder()
* .name("losangeles001")
* .type("EdgeZone")
* .build())
* .isHnsEnabled(true)
* .keyPolicy(KeyPolicyArgs.builder()
* .keyExpirationPeriodInDays(20)
* .build())
* .kind("Storage")
* .location("eastus")
* .minimumTlsVersion("TLS1_2")
* .publicNetworkAccess("Disabled")
* .resourceGroupName("res9101")
* .routingPreference(RoutingPreferenceArgs.builder()
* .publishInternetEndpoints(true)
* .publishMicrosoftEndpoints(true)
* .routingChoice("MicrosoftRouting")
* .build())
* .sasPolicy(SasPolicyArgs.builder()
* .expirationAction("Log")
* .sasExpirationPeriod("1.15:59:59")
* .build())
* .sku(SkuArgs.builder()
* .name("Standard_GRS")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .build());
*
* }
* }
*
* }
*
* ### StorageAccountCreateDnsEndpointTypeToAzureDnsZone
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServicesArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServiceArgs;
* import com.pulumi.azurenative.storage.inputs.ExtendedLocationArgs;
* import com.pulumi.azurenative.storage.inputs.KeyPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.RoutingPreferenceArgs;
* import com.pulumi.azurenative.storage.inputs.SasPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .allowBlobPublicAccess(false)
* .allowSharedKeyAccess(true)
* .defaultToOAuthAuthentication(false)
* .dnsEndpointType("AzureDnsZone")
* .encryption(EncryptionArgs.builder()
* .keySource("Microsoft.Storage")
* .requireInfrastructureEncryption(false)
* .services(EncryptionServicesArgs.builder()
* .blob(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .file(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .build())
* .build())
* .extendedLocation(ExtendedLocationArgs.builder()
* .name("losangeles001")
* .type("EdgeZone")
* .build())
* .isHnsEnabled(true)
* .isSftpEnabled(true)
* .keyPolicy(KeyPolicyArgs.builder()
* .keyExpirationPeriodInDays(20)
* .build())
* .kind("Storage")
* .location("eastus")
* .minimumTlsVersion("TLS1_2")
* .resourceGroupName("res9101")
* .routingPreference(RoutingPreferenceArgs.builder()
* .publishInternetEndpoints(true)
* .publishMicrosoftEndpoints(true)
* .routingChoice("MicrosoftRouting")
* .build())
* .sasPolicy(SasPolicyArgs.builder()
* .expirationAction("Log")
* .sasExpirationPeriod("1.15:59:59")
* .build())
* .sku(SkuArgs.builder()
* .name("Standard_GRS")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .build());
*
* }
* }
*
* }
*
* ### StorageAccountCreateDnsEndpointTypeToStandard
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServicesArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServiceArgs;
* import com.pulumi.azurenative.storage.inputs.ExtendedLocationArgs;
* import com.pulumi.azurenative.storage.inputs.KeyPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.RoutingPreferenceArgs;
* import com.pulumi.azurenative.storage.inputs.SasPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .allowBlobPublicAccess(false)
* .allowSharedKeyAccess(true)
* .defaultToOAuthAuthentication(false)
* .dnsEndpointType("Standard")
* .encryption(EncryptionArgs.builder()
* .keySource("Microsoft.Storage")
* .requireInfrastructureEncryption(false)
* .services(EncryptionServicesArgs.builder()
* .blob(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .file(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .build())
* .build())
* .extendedLocation(ExtendedLocationArgs.builder()
* .name("losangeles001")
* .type("EdgeZone")
* .build())
* .isHnsEnabled(true)
* .isSftpEnabled(true)
* .keyPolicy(KeyPolicyArgs.builder()
* .keyExpirationPeriodInDays(20)
* .build())
* .kind("Storage")
* .location("eastus")
* .minimumTlsVersion("TLS1_2")
* .resourceGroupName("res9101")
* .routingPreference(RoutingPreferenceArgs.builder()
* .publishInternetEndpoints(true)
* .publishMicrosoftEndpoints(true)
* .routingChoice("MicrosoftRouting")
* .build())
* .sasPolicy(SasPolicyArgs.builder()
* .expirationAction("Log")
* .sasExpirationPeriod("1.15:59:59")
* .build())
* .sku(SkuArgs.builder()
* .name("Standard_GRS")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .build());
*
* }
* }
*
* }
*
* ### StorageAccountCreateEnablePublicNetworkAccess
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServicesArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServiceArgs;
* import com.pulumi.azurenative.storage.inputs.ExtendedLocationArgs;
* import com.pulumi.azurenative.storage.inputs.KeyPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.RoutingPreferenceArgs;
* import com.pulumi.azurenative.storage.inputs.SasPolicyArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .allowBlobPublicAccess(false)
* .allowSharedKeyAccess(true)
* .encryption(EncryptionArgs.builder()
* .keySource("Microsoft.Storage")
* .requireInfrastructureEncryption(false)
* .services(EncryptionServicesArgs.builder()
* .blob(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .file(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .build())
* .build())
* .extendedLocation(ExtendedLocationArgs.builder()
* .name("losangeles001")
* .type("EdgeZone")
* .build())
* .isHnsEnabled(true)
* .keyPolicy(KeyPolicyArgs.builder()
* .keyExpirationPeriodInDays(20)
* .build())
* .kind("Storage")
* .location("eastus")
* .minimumTlsVersion("TLS1_2")
* .publicNetworkAccess("Enabled")
* .resourceGroupName("res9101")
* .routingPreference(RoutingPreferenceArgs.builder()
* .publishInternetEndpoints(true)
* .publishMicrosoftEndpoints(true)
* .routingChoice("MicrosoftRouting")
* .build())
* .sasPolicy(SasPolicyArgs.builder()
* .expirationAction("Log")
* .sasExpirationPeriod("1.15:59:59")
* .build())
* .sku(SkuArgs.builder()
* .name("Standard_GRS")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .build());
*
* }
* }
*
* }
*
* ### StorageAccountCreatePremiumBlockBlobStorage
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServicesArgs;
* import com.pulumi.azurenative.storage.inputs.EncryptionServiceArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .allowSharedKeyAccess(true)
* .encryption(EncryptionArgs.builder()
* .keySource("Microsoft.Storage")
* .requireInfrastructureEncryption(false)
* .services(EncryptionServicesArgs.builder()
* .blob(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .file(EncryptionServiceArgs.builder()
* .enabled(true)
* .keyType("Account")
* .build())
* .build())
* .build())
* .kind("BlockBlobStorage")
* .location("eastus")
* .minimumTlsVersion("TLS1_2")
* .resourceGroupName("res9101")
* .sku(SkuArgs.builder()
* .name("Premium_LRS")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .build());
*
* }
* }
*
* }
*
* ### StorageAccountCreateWithImmutabilityPolicy
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.StorageAccount;
* import com.pulumi.azurenative.storage.StorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.ExtendedLocationArgs;
* import com.pulumi.azurenative.storage.inputs.ImmutableStorageAccountArgs;
* import com.pulumi.azurenative.storage.inputs.AccountImmutabilityPolicyPropertiesArgs;
* import com.pulumi.azurenative.storage.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var storageAccount = new StorageAccount("storageAccount", StorageAccountArgs.builder()
* .accountName("sto4445")
* .extendedLocation(ExtendedLocationArgs.builder()
* .name("losangeles001")
* .type("EdgeZone")
* .build())
* .immutableStorageWithVersioning(ImmutableStorageAccountArgs.builder()
* .enabled(true)
* .immutabilityPolicy(AccountImmutabilityPolicyPropertiesArgs.builder()
* .allowProtectedAppendWrites(true)
* .immutabilityPeriodSinceCreationInDays(15)
* .state("Unlocked")
* .build())
* .build())
* .kind("Storage")
* .location("eastus")
* .resourceGroupName("res9101")
* .sku(SkuArgs.builder()
* .name("Standard_GRS")
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:storage:StorageAccount sto4445 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Storage/storageAccounts/{accountName}
* ```
*
*/
@ResourceType(type="azure-native:storage:StorageAccount")
public class StorageAccount extends com.pulumi.resources.CustomResource {
/**
* Required for storage accounts where kind = BlobStorage. The access tier is used for billing. The 'Premium' access tier is the default value for premium block blobs storage account type and it cannot be changed for the premium block blobs storage account type.
*
*/
@Export(name="accessTier", refs={String.class}, tree="[0]")
private Output accessTier;
/**
* @return Required for storage accounts where kind = BlobStorage. The access tier is used for billing. The 'Premium' access tier is the default value for premium block blobs storage account type and it cannot be changed for the premium block blobs storage account type.
*
*/
public Output accessTier() {
return this.accessTier;
}
/**
* Allow or disallow public access to all blobs or containers in the storage account. The default interpretation is true for this property.
*
*/
@Export(name="allowBlobPublicAccess", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> allowBlobPublicAccess;
/**
* @return Allow or disallow public access to all blobs or containers in the storage account. The default interpretation is true for this property.
*
*/
public Output> allowBlobPublicAccess() {
return Codegen.optional(this.allowBlobPublicAccess);
}
/**
* Allow or disallow cross AAD tenant object replication. The default interpretation is true for this property.
*
*/
@Export(name="allowCrossTenantReplication", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> allowCrossTenantReplication;
/**
* @return Allow or disallow cross AAD tenant object replication. The default interpretation is true for this property.
*
*/
public Output> allowCrossTenantReplication() {
return Codegen.optional(this.allowCrossTenantReplication);
}
/**
* Indicates whether the storage account permits requests to be authorized with the account access key via Shared Key. If false, then all requests, including shared access signatures, must be authorized with Azure Active Directory (Azure AD). The default value is null, which is equivalent to true.
*
*/
@Export(name="allowSharedKeyAccess", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> allowSharedKeyAccess;
/**
* @return Indicates whether the storage account permits requests to be authorized with the account access key via Shared Key. If false, then all requests, including shared access signatures, must be authorized with Azure Active Directory (Azure AD). The default value is null, which is equivalent to true.
*
*/
public Output> allowSharedKeyAccess() {
return Codegen.optional(this.allowSharedKeyAccess);
}
/**
* Restrict copy to and from Storage Accounts within an AAD tenant or with Private Links to the same VNet.
*
*/
@Export(name="allowedCopyScope", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> allowedCopyScope;
/**
* @return Restrict copy to and from Storage Accounts within an AAD tenant or with Private Links to the same VNet.
*
*/
public Output> allowedCopyScope() {
return Codegen.optional(this.allowedCopyScope);
}
/**
* Provides the identity based authentication settings for Azure Files.
*
*/
@Export(name="azureFilesIdentityBasedAuthentication", refs={AzureFilesIdentityBasedAuthenticationResponse.class}, tree="[0]")
private Output* @Nullable */ AzureFilesIdentityBasedAuthenticationResponse> azureFilesIdentityBasedAuthentication;
/**
* @return Provides the identity based authentication settings for Azure Files.
*
*/
public Output> azureFilesIdentityBasedAuthentication() {
return Codegen.optional(this.azureFilesIdentityBasedAuthentication);
}
/**
* Blob restore status
*
*/
@Export(name="blobRestoreStatus", refs={BlobRestoreStatusResponse.class}, tree="[0]")
private Output blobRestoreStatus;
/**
* @return Blob restore status
*
*/
public Output blobRestoreStatus() {
return this.blobRestoreStatus;
}
/**
* Gets the creation date and time of the storage account in UTC.
*
*/
@Export(name="creationTime", refs={String.class}, tree="[0]")
private Output creationTime;
/**
* @return Gets the creation date and time of the storage account in UTC.
*
*/
public Output creationTime() {
return this.creationTime;
}
/**
* Gets the custom domain the user assigned to this storage account.
*
*/
@Export(name="customDomain", refs={CustomDomainResponse.class}, tree="[0]")
private Output customDomain;
/**
* @return Gets the custom domain the user assigned to this storage account.
*
*/
public Output customDomain() {
return this.customDomain;
}
/**
* A boolean flag which indicates whether the default authentication is OAuth or not. The default interpretation is false for this property.
*
*/
@Export(name="defaultToOAuthAuthentication", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> defaultToOAuthAuthentication;
/**
* @return A boolean flag which indicates whether the default authentication is OAuth or not. The default interpretation is false for this property.
*
*/
public Output> defaultToOAuthAuthentication() {
return Codegen.optional(this.defaultToOAuthAuthentication);
}
/**
* Allows you to specify the type of endpoint. Set this to AzureDNSZone to create a large number of accounts in a single subscription, which creates accounts in an Azure DNS Zone and the endpoint URL will have an alphanumeric DNS Zone identifier.
*
*/
@Export(name="dnsEndpointType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dnsEndpointType;
/**
* @return Allows you to specify the type of endpoint. Set this to AzureDNSZone to create a large number of accounts in a single subscription, which creates accounts in an Azure DNS Zone and the endpoint URL will have an alphanumeric DNS Zone identifier.
*
*/
public Output> dnsEndpointType() {
return Codegen.optional(this.dnsEndpointType);
}
/**
* Allows https traffic only to storage service if sets to true.
*
*/
@Export(name="enableHttpsTrafficOnly", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableHttpsTrafficOnly;
/**
* @return Allows https traffic only to storage service if sets to true.
*
*/
public Output> enableHttpsTrafficOnly() {
return Codegen.optional(this.enableHttpsTrafficOnly);
}
/**
* NFS 3.0 protocol support enabled if set to true.
*
*/
@Export(name="enableNfsV3", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableNfsV3;
/**
* @return NFS 3.0 protocol support enabled if set to true.
*
*/
public Output> enableNfsV3() {
return Codegen.optional(this.enableNfsV3);
}
/**
* Encryption settings to be used for server-side encryption for the storage account.
*
*/
@Export(name="encryption", refs={EncryptionResponse.class}, tree="[0]")
private Output encryption;
/**
* @return Encryption settings to be used for server-side encryption for the storage account.
*
*/
public Output encryption() {
return this.encryption;
}
/**
* The extendedLocation of the resource.
*
*/
@Export(name="extendedLocation", refs={ExtendedLocationResponse.class}, tree="[0]")
private Output* @Nullable */ ExtendedLocationResponse> extendedLocation;
/**
* @return The extendedLocation of the resource.
*
*/
public Output> extendedLocation() {
return Codegen.optional(this.extendedLocation);
}
/**
* If the failover is in progress, the value will be true, otherwise, it will be null.
*
*/
@Export(name="failoverInProgress", refs={Boolean.class}, tree="[0]")
private Output failoverInProgress;
/**
* @return If the failover is in progress, the value will be true, otherwise, it will be null.
*
*/
public Output failoverInProgress() {
return this.failoverInProgress;
}
/**
* Geo Replication Stats
*
*/
@Export(name="geoReplicationStats", refs={GeoReplicationStatsResponse.class}, tree="[0]")
private Output geoReplicationStats;
/**
* @return Geo Replication Stats
*
*/
public Output geoReplicationStats() {
return this.geoReplicationStats;
}
/**
* The identity of the resource.
*
*/
@Export(name="identity", refs={IdentityResponse.class}, tree="[0]")
private Output* @Nullable */ IdentityResponse> identity;
/**
* @return The identity of the resource.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* The property is immutable and can only be set to true at the account creation time. When set to true, it enables object level immutability for all the containers in the account by default.
*
*/
@Export(name="immutableStorageWithVersioning", refs={ImmutableStorageAccountResponse.class}, tree="[0]")
private Output* @Nullable */ ImmutableStorageAccountResponse> immutableStorageWithVersioning;
/**
* @return The property is immutable and can only be set to true at the account creation time. When set to true, it enables object level immutability for all the containers in the account by default.
*
*/
public Output> immutableStorageWithVersioning() {
return Codegen.optional(this.immutableStorageWithVersioning);
}
/**
* Account HierarchicalNamespace enabled if sets to true.
*
*/
@Export(name="isHnsEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> isHnsEnabled;
/**
* @return Account HierarchicalNamespace enabled if sets to true.
*
*/
public Output> isHnsEnabled() {
return Codegen.optional(this.isHnsEnabled);
}
/**
* Enables local users feature, if set to true
*
*/
@Export(name="isLocalUserEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> isLocalUserEnabled;
/**
* @return Enables local users feature, if set to true
*
*/
public Output> isLocalUserEnabled() {
return Codegen.optional(this.isLocalUserEnabled);
}
/**
* Enables Secure File Transfer Protocol, if set to true
*
*/
@Export(name="isSftpEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> isSftpEnabled;
/**
* @return Enables Secure File Transfer Protocol, if set to true
*
*/
public Output> isSftpEnabled() {
return Codegen.optional(this.isSftpEnabled);
}
/**
* Storage account keys creation time.
*
*/
@Export(name="keyCreationTime", refs={KeyCreationTimeResponse.class}, tree="[0]")
private Output keyCreationTime;
/**
* @return Storage account keys creation time.
*
*/
public Output keyCreationTime() {
return this.keyCreationTime;
}
/**
* KeyPolicy assigned to the storage account.
*
*/
@Export(name="keyPolicy", refs={KeyPolicyResponse.class}, tree="[0]")
private Output keyPolicy;
/**
* @return KeyPolicy assigned to the storage account.
*
*/
public Output keyPolicy() {
return this.keyPolicy;
}
/**
* Gets the Kind.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return Gets the Kind.
*
*/
public Output kind() {
return this.kind;
}
/**
* Allow large file shares if sets to Enabled. It cannot be disabled once it is enabled.
*
*/
@Export(name="largeFileSharesState", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> largeFileSharesState;
/**
* @return Allow large file shares if sets to Enabled. It cannot be disabled once it is enabled.
*
*/
public Output> largeFileSharesState() {
return Codegen.optional(this.largeFileSharesState);
}
/**
* Gets the timestamp of the most recent instance of a failover to the secondary location. Only the most recent timestamp is retained. This element is not returned if there has never been a failover instance. Only available if the accountType is Standard_GRS or Standard_RAGRS.
*
*/
@Export(name="lastGeoFailoverTime", refs={String.class}, tree="[0]")
private Output lastGeoFailoverTime;
/**
* @return Gets the timestamp of the most recent instance of a failover to the secondary location. Only the most recent timestamp is retained. This element is not returned if there has never been a failover instance. Only available if the accountType is Standard_GRS or Standard_RAGRS.
*
*/
public Output lastGeoFailoverTime() {
return this.lastGeoFailoverTime;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* Set the minimum TLS version to be permitted on requests to storage. The default interpretation is TLS 1.0 for this property.
*
*/
@Export(name="minimumTlsVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> minimumTlsVersion;
/**
* @return Set the minimum TLS version to be permitted on requests to storage. The default interpretation is TLS 1.0 for this property.
*
*/
public Output> minimumTlsVersion() {
return Codegen.optional(this.minimumTlsVersion);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Network rule set
*
*/
@Export(name="networkRuleSet", refs={NetworkRuleSetResponse.class}, tree="[0]")
private Output networkRuleSet;
/**
* @return Network rule set
*
*/
public Output networkRuleSet() {
return this.networkRuleSet;
}
/**
* Gets the URLs that are used to perform a retrieval of a public blob, queue, or table object. Note that Standard_ZRS and Premium_LRS accounts only return the blob endpoint.
*
*/
@Export(name="primaryEndpoints", refs={EndpointsResponse.class}, tree="[0]")
private Output primaryEndpoints;
/**
* @return Gets the URLs that are used to perform a retrieval of a public blob, queue, or table object. Note that Standard_ZRS and Premium_LRS accounts only return the blob endpoint.
*
*/
public Output primaryEndpoints() {
return this.primaryEndpoints;
}
/**
* Gets the location of the primary data center for the storage account.
*
*/
@Export(name="primaryLocation", refs={String.class}, tree="[0]")
private Output primaryLocation;
/**
* @return Gets the location of the primary data center for the storage account.
*
*/
public Output primaryLocation() {
return this.primaryLocation;
}
/**
* List of private endpoint connection associated with the specified storage account
*
*/
@Export(name="privateEndpointConnections", refs={List.class,PrivateEndpointConnectionResponse.class}, tree="[0,1]")
private Output> privateEndpointConnections;
/**
* @return List of private endpoint connection associated with the specified storage account
*
*/
public Output> privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* Gets the status of the storage account at the time the operation was called.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Gets the status of the storage account at the time the operation was called.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Allow or disallow public network access to Storage Account. Value is optional but if passed in, must be 'Enabled' or 'Disabled'.
*
*/
@Export(name="publicNetworkAccess", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publicNetworkAccess;
/**
* @return Allow or disallow public network access to Storage Account. Value is optional but if passed in, must be 'Enabled' or 'Disabled'.
*
*/
public Output> publicNetworkAccess() {
return Codegen.optional(this.publicNetworkAccess);
}
/**
* Maintains information about the network routing choice opted by the user for data transfer
*
*/
@Export(name="routingPreference", refs={RoutingPreferenceResponse.class}, tree="[0]")
private Output* @Nullable */ RoutingPreferenceResponse> routingPreference;
/**
* @return Maintains information about the network routing choice opted by the user for data transfer
*
*/
public Output> routingPreference() {
return Codegen.optional(this.routingPreference);
}
/**
* SasPolicy assigned to the storage account.
*
*/
@Export(name="sasPolicy", refs={SasPolicyResponse.class}, tree="[0]")
private Output sasPolicy;
/**
* @return SasPolicy assigned to the storage account.
*
*/
public Output sasPolicy() {
return this.sasPolicy;
}
/**
* Gets the URLs that are used to perform a retrieval of a public blob, queue, or table object from the secondary location of the storage account. Only available if the SKU name is Standard_RAGRS.
*
*/
@Export(name="secondaryEndpoints", refs={EndpointsResponse.class}, tree="[0]")
private Output secondaryEndpoints;
/**
* @return Gets the URLs that are used to perform a retrieval of a public blob, queue, or table object from the secondary location of the storage account. Only available if the SKU name is Standard_RAGRS.
*
*/
public Output secondaryEndpoints() {
return this.secondaryEndpoints;
}
/**
* Gets the location of the geo-replicated secondary for the storage account. Only available if the accountType is Standard_GRS or Standard_RAGRS.
*
*/
@Export(name="secondaryLocation", refs={String.class}, tree="[0]")
private Output secondaryLocation;
/**
* @return Gets the location of the geo-replicated secondary for the storage account. Only available if the accountType is Standard_GRS or Standard_RAGRS.
*
*/
public Output secondaryLocation() {
return this.secondaryLocation;
}
/**
* Gets the SKU.
*
*/
@Export(name="sku", refs={SkuResponse.class}, tree="[0]")
private Output sku;
/**
* @return Gets the SKU.
*
*/
public Output sku() {
return this.sku;
}
/**
* Gets the status indicating whether the primary location of the storage account is available or unavailable.
*
*/
@Export(name="statusOfPrimary", refs={String.class}, tree="[0]")
private Output statusOfPrimary;
/**
* @return Gets the status indicating whether the primary location of the storage account is available or unavailable.
*
*/
public Output statusOfPrimary() {
return this.statusOfPrimary;
}
/**
* Gets the status indicating whether the secondary location of the storage account is available or unavailable. Only available if the SKU name is Standard_GRS or Standard_RAGRS.
*
*/
@Export(name="statusOfSecondary", refs={String.class}, tree="[0]")
private Output statusOfSecondary;
/**
* @return Gets the status indicating whether the secondary location of the storage account is available or unavailable. Only available if the SKU name is Standard_GRS or Standard_RAGRS.
*
*/
public Output statusOfSecondary() {
return this.statusOfSecondary;
}
/**
* This property is readOnly and is set by server during asynchronous storage account sku conversion operations.
*
*/
@Export(name="storageAccountSkuConversionStatus", refs={StorageAccountSkuConversionStatusResponse.class}, tree="[0]")
private Output* @Nullable */ StorageAccountSkuConversionStatusResponse> storageAccountSkuConversionStatus;
/**
* @return This property is readOnly and is set by server during asynchronous storage account sku conversion operations.
*
*/
public Output> storageAccountSkuConversionStatus() {
return Codegen.optional(this.storageAccountSkuConversionStatus);
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public StorageAccount(java.lang.String name) {
this(name, StorageAccountArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public StorageAccount(java.lang.String name, StorageAccountArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public StorageAccount(java.lang.String name, StorageAccountArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storage:StorageAccount", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private StorageAccount(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storage:StorageAccount", name, null, makeResourceOptions(options, id), false);
}
private static StorageAccountArgs makeArgs(StorageAccountArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? StorageAccountArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:storage/v20150501preview:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20150615:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20160101:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20160501:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20161201:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20170601:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20171001:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20180201:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20180301preview:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20180701:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20181101:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20190401:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20190601:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20200801preview:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210101:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210201:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210401:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210601:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210801:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210901:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20220501:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20220901:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20230101:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20230401:StorageAccount").build()),
Output.of(Alias.builder().type("azure-native:storage/v20230501:StorageAccount").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static StorageAccount get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new StorageAccount(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy