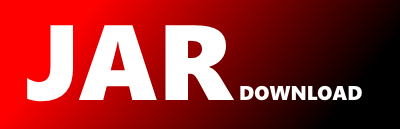
com.pulumi.azurenative.storagecache.ImportJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storagecache;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.storagecache.ImportJobArgs;
import com.pulumi.azurenative.storagecache.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* An import job instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
* Azure REST API version: 2024-03-01.
*
* ## Example Usage
* ### importJobs_CreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storagecache.ImportJob;
* import com.pulumi.azurenative.storagecache.ImportJobArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var importJob = new ImportJob("importJob", ImportJobArgs.builder()
* .amlFilesystemName("fs1")
* .conflictResolutionMode("OverwriteAlways")
* .importJobName("job1")
* .importPrefixes("/")
* .location("eastus")
* .maximumErrors(0)
* .resourceGroupName("scgroup")
* .tags(Map.of("Dept", "ContosoAds"))
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:storagecache:ImportJob job1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.StorageCache/amlFilesystems/{amlFilesystemName}/importJobs/{importJobName}
* ```
*
*/
@ResourceType(type="azure-native:storagecache:ImportJob")
public class ImportJob extends com.pulumi.resources.CustomResource {
/**
* A recent and frequently updated rate of total files, directories, and symlinks imported per second.
*
*/
@Export(name="blobsImportedPerSecond", refs={Double.class}, tree="[0]")
private Output blobsImportedPerSecond;
/**
* @return A recent and frequently updated rate of total files, directories, and symlinks imported per second.
*
*/
public Output blobsImportedPerSecond() {
return this.blobsImportedPerSecond;
}
/**
* A recent and frequently updated rate of blobs walked per second.
*
*/
@Export(name="blobsWalkedPerSecond", refs={Double.class}, tree="[0]")
private Output blobsWalkedPerSecond;
/**
* @return A recent and frequently updated rate of blobs walked per second.
*
*/
public Output blobsWalkedPerSecond() {
return this.blobsWalkedPerSecond;
}
/**
* How the import job will handle conflicts. For example, if the import job is trying to bring in a directory, but a file is at that path, how it handles it. Fail indicates that the import job should stop immediately and not do anything with the conflict. Skip indicates that it should pass over the conflict. OverwriteIfDirty causes the import job to delete and re-import the file or directory if it is a conflicting type, is dirty, or was not previously imported. OverwriteAlways extends OverwriteIfDirty to include releasing files that had been restored but were not dirty. Please reference https://learn.microsoft.com/en-us/azure/azure-managed-lustre/ for a thorough explanation of these resolution modes.
*
*/
@Export(name="conflictResolutionMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> conflictResolutionMode;
/**
* @return How the import job will handle conflicts. For example, if the import job is trying to bring in a directory, but a file is at that path, how it handles it. Fail indicates that the import job should stop immediately and not do anything with the conflict. Skip indicates that it should pass over the conflict. OverwriteIfDirty causes the import job to delete and re-import the file or directory if it is a conflicting type, is dirty, or was not previously imported. OverwriteAlways extends OverwriteIfDirty to include releasing files that had been restored but were not dirty. Please reference https://learn.microsoft.com/en-us/azure/azure-managed-lustre/ for a thorough explanation of these resolution modes.
*
*/
public Output> conflictResolutionMode() {
return Codegen.optional(this.conflictResolutionMode);
}
/**
* An array of blob paths/prefixes that get imported into the cluster namespace. It has '/' as the default value.
*
*/
@Export(name="importPrefixes", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> importPrefixes;
/**
* @return An array of blob paths/prefixes that get imported into the cluster namespace. It has '/' as the default value.
*
*/
public Output>> importPrefixes() {
return Codegen.optional(this.importPrefixes);
}
/**
* The time of the last completed archive operation
*
*/
@Export(name="lastCompletionTime", refs={String.class}, tree="[0]")
private Output lastCompletionTime;
/**
* @return The time of the last completed archive operation
*
*/
public Output lastCompletionTime() {
return this.lastCompletionTime;
}
/**
* The time the latest archive operation started
*
*/
@Export(name="lastStartedTime", refs={String.class}, tree="[0]")
private Output lastStartedTime;
/**
* @return The time the latest archive operation started
*
*/
public Output lastStartedTime() {
return this.lastStartedTime;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* Total non-conflict oriented errors the import job will tolerate before exiting with failure. -1 means infinite. 0 means exit immediately and is the default.
*
*/
@Export(name="maximumErrors", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maximumErrors;
/**
* @return Total non-conflict oriented errors the import job will tolerate before exiting with failure. -1 means infinite. 0 means exit immediately and is the default.
*
*/
public Output> maximumErrors() {
return Codegen.optional(this.maximumErrors);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* ARM provisioning state.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return ARM provisioning state.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The state of the import job. InProgress indicates the import is still running. Canceled indicates it has been canceled by the user. Completed indicates import finished, successfully importing all discovered blobs into the Lustre namespace. CompletedPartial indicates the import finished but some blobs either were found to be conflicting and could not be imported or other errors were encountered. Failed means the import was unable to complete due to a fatal error.
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output state;
/**
* @return The state of the import job. InProgress indicates the import is still running. Canceled indicates it has been canceled by the user. Completed indicates import finished, successfully importing all discovered blobs into the Lustre namespace. CompletedPartial indicates the import finished but some blobs either were found to be conflicting and could not be imported or other errors were encountered. Failed means the import was unable to complete due to a fatal error.
*
*/
public Output state() {
return this.state;
}
/**
* The status message of the import job.
*
*/
@Export(name="statusMessage", refs={String.class}, tree="[0]")
private Output statusMessage;
/**
* @return The status message of the import job.
*
*/
public Output statusMessage() {
return this.statusMessage;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The total blobs that have been imported since import began.
*
*/
@Export(name="totalBlobsImported", refs={Double.class}, tree="[0]")
private Output totalBlobsImported;
/**
* @return The total blobs that have been imported since import began.
*
*/
public Output totalBlobsImported() {
return this.totalBlobsImported;
}
/**
* The total blob objects walked.
*
*/
@Export(name="totalBlobsWalked", refs={Double.class}, tree="[0]")
private Output totalBlobsWalked;
/**
* @return The total blob objects walked.
*
*/
public Output totalBlobsWalked() {
return this.totalBlobsWalked;
}
/**
* Number of conflicts in the import job.
*
*/
@Export(name="totalConflicts", refs={Integer.class}, tree="[0]")
private Output totalConflicts;
/**
* @return Number of conflicts in the import job.
*
*/
public Output totalConflicts() {
return this.totalConflicts;
}
/**
* Number of errors in the import job.
*
*/
@Export(name="totalErrors", refs={Integer.class}, tree="[0]")
private Output totalErrors;
/**
* @return Number of errors in the import job.
*
*/
public Output totalErrors() {
return this.totalErrors;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ImportJob(java.lang.String name) {
this(name, ImportJobArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ImportJob(java.lang.String name, ImportJobArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ImportJob(java.lang.String name, ImportJobArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storagecache:ImportJob", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ImportJob(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storagecache:ImportJob", name, null, makeResourceOptions(options, id), false);
}
private static ImportJobArgs makeArgs(ImportJobArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ImportJobArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:storagecache:importJob").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20240301:ImportJob").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20240301:importJob").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ImportJob get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ImportJob(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy