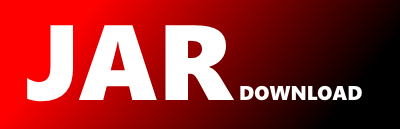
com.pulumi.azurenative.storagepool.outputs.GetDiskPoolResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storagepool.outputs;
import com.pulumi.azurenative.storagepool.outputs.DiskResponse;
import com.pulumi.azurenative.storagepool.outputs.SystemMetadataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDiskPoolResult {
/**
* @return List of additional capabilities for Disk Pool.
*
*/
private @Nullable List additionalCapabilities;
/**
* @return Logical zone for Disk Pool resource; example: ["1"].
*
*/
private List availabilityZones;
/**
* @return List of Azure Managed Disks to attach to a Disk Pool.
*
*/
private @Nullable List disks;
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives.
*
*/
private String location;
/**
* @return Azure resource id. Indicates if this resource is managed by another Azure resource.
*
*/
private String managedBy;
/**
* @return List of Azure resource ids that manage this resource.
*
*/
private List managedByExtended;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return State of the operation on the resource.
*
*/
private String provisioningState;
/**
* @return Operational status of the Disk Pool.
*
*/
private String status;
/**
* @return Azure Resource ID of a Subnet for the Disk Pool.
*
*/
private String subnetId;
/**
* @return Resource metadata required by ARM RPC
*
*/
private SystemMetadataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Sku tier
*
*/
private @Nullable String tier;
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
private String type;
private GetDiskPoolResult() {}
/**
* @return List of additional capabilities for Disk Pool.
*
*/
public List additionalCapabilities() {
return this.additionalCapabilities == null ? List.of() : this.additionalCapabilities;
}
/**
* @return Logical zone for Disk Pool resource; example: ["1"].
*
*/
public List availabilityZones() {
return this.availabilityZones;
}
/**
* @return List of Azure Managed Disks to attach to a Disk Pool.
*
*/
public List disks() {
return this.disks == null ? List.of() : this.disks;
}
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives.
*
*/
public String location() {
return this.location;
}
/**
* @return Azure resource id. Indicates if this resource is managed by another Azure resource.
*
*/
public String managedBy() {
return this.managedBy;
}
/**
* @return List of Azure resource ids that manage this resource.
*
*/
public List managedByExtended() {
return this.managedByExtended;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return State of the operation on the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Operational status of the Disk Pool.
*
*/
public String status() {
return this.status;
}
/**
* @return Azure Resource ID of a Subnet for the Disk Pool.
*
*/
public String subnetId() {
return this.subnetId;
}
/**
* @return Resource metadata required by ARM RPC
*
*/
public SystemMetadataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Sku tier
*
*/
public Optional tier() {
return Optional.ofNullable(this.tier);
}
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDiskPoolResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List additionalCapabilities;
private List availabilityZones;
private @Nullable List disks;
private String id;
private String location;
private String managedBy;
private List managedByExtended;
private String name;
private String provisioningState;
private String status;
private String subnetId;
private SystemMetadataResponse systemData;
private @Nullable Map tags;
private @Nullable String tier;
private String type;
public Builder() {}
public Builder(GetDiskPoolResult defaults) {
Objects.requireNonNull(defaults);
this.additionalCapabilities = defaults.additionalCapabilities;
this.availabilityZones = defaults.availabilityZones;
this.disks = defaults.disks;
this.id = defaults.id;
this.location = defaults.location;
this.managedBy = defaults.managedBy;
this.managedByExtended = defaults.managedByExtended;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.status = defaults.status;
this.subnetId = defaults.subnetId;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.tier = defaults.tier;
this.type = defaults.type;
}
@CustomType.Setter
public Builder additionalCapabilities(@Nullable List additionalCapabilities) {
this.additionalCapabilities = additionalCapabilities;
return this;
}
public Builder additionalCapabilities(String... additionalCapabilities) {
return additionalCapabilities(List.of(additionalCapabilities));
}
@CustomType.Setter
public Builder availabilityZones(List availabilityZones) {
if (availabilityZones == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "availabilityZones");
}
this.availabilityZones = availabilityZones;
return this;
}
public Builder availabilityZones(String... availabilityZones) {
return availabilityZones(List.of(availabilityZones));
}
@CustomType.Setter
public Builder disks(@Nullable List disks) {
this.disks = disks;
return this;
}
public Builder disks(DiskResponse... disks) {
return disks(List.of(disks));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder managedBy(String managedBy) {
if (managedBy == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "managedBy");
}
this.managedBy = managedBy;
return this;
}
@CustomType.Setter
public Builder managedByExtended(List managedByExtended) {
if (managedByExtended == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "managedByExtended");
}
this.managedByExtended = managedByExtended;
return this;
}
public Builder managedByExtended(String... managedByExtended) {
return managedByExtended(List.of(managedByExtended));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder subnetId(String subnetId) {
if (subnetId == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "subnetId");
}
this.subnetId = subnetId;
return this;
}
@CustomType.Setter
public Builder systemData(SystemMetadataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder tier(@Nullable String tier) {
this.tier = tier;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDiskPoolResult", "type");
}
this.type = type;
return this;
}
public GetDiskPoolResult build() {
final var _resultValue = new GetDiskPoolResult();
_resultValue.additionalCapabilities = additionalCapabilities;
_resultValue.availabilityZones = availabilityZones;
_resultValue.disks = disks;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.managedBy = managedBy;
_resultValue.managedByExtended = managedByExtended;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.status = status;
_resultValue.subnetId = subnetId;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.tier = tier;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy