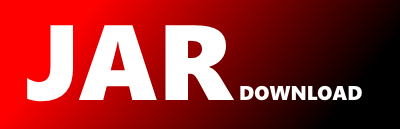
com.pulumi.azurenative.storagesync.outputs.ServerEndpointSyncStatusResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storagesync.outputs;
import com.pulumi.azurenative.storagesync.outputs.ServerEndpointBackgroundDataDownloadActivityResponse;
import com.pulumi.azurenative.storagesync.outputs.ServerEndpointSyncActivityStatusResponse;
import com.pulumi.azurenative.storagesync.outputs.ServerEndpointSyncSessionStatusResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class ServerEndpointSyncStatusResponse {
/**
* @return Background data download activity
*
*/
private ServerEndpointBackgroundDataDownloadActivityResponse backgroundDataDownloadActivity;
/**
* @return Combined Health Status.
*
*/
private String combinedHealth;
/**
* @return Download sync activity
*
*/
private ServerEndpointSyncActivityStatusResponse downloadActivity;
/**
* @return Download Health Status.
*
*/
private String downloadHealth;
/**
* @return Download Status
*
*/
private ServerEndpointSyncSessionStatusResponse downloadStatus;
/**
* @return Last Updated Timestamp
*
*/
private String lastUpdatedTimestamp;
/**
* @return Offline Data Transfer State
*
*/
private String offlineDataTransferStatus;
/**
* @return Sync activity
*
*/
private String syncActivity;
/**
* @return Total count of persistent files not syncing (combined upload + download).
*
*/
private Double totalPersistentFilesNotSyncingCount;
/**
* @return Upload sync activity
*
*/
private ServerEndpointSyncActivityStatusResponse uploadActivity;
/**
* @return Upload Health Status.
*
*/
private String uploadHealth;
/**
* @return Upload Status
*
*/
private ServerEndpointSyncSessionStatusResponse uploadStatus;
private ServerEndpointSyncStatusResponse() {}
/**
* @return Background data download activity
*
*/
public ServerEndpointBackgroundDataDownloadActivityResponse backgroundDataDownloadActivity() {
return this.backgroundDataDownloadActivity;
}
/**
* @return Combined Health Status.
*
*/
public String combinedHealth() {
return this.combinedHealth;
}
/**
* @return Download sync activity
*
*/
public ServerEndpointSyncActivityStatusResponse downloadActivity() {
return this.downloadActivity;
}
/**
* @return Download Health Status.
*
*/
public String downloadHealth() {
return this.downloadHealth;
}
/**
* @return Download Status
*
*/
public ServerEndpointSyncSessionStatusResponse downloadStatus() {
return this.downloadStatus;
}
/**
* @return Last Updated Timestamp
*
*/
public String lastUpdatedTimestamp() {
return this.lastUpdatedTimestamp;
}
/**
* @return Offline Data Transfer State
*
*/
public String offlineDataTransferStatus() {
return this.offlineDataTransferStatus;
}
/**
* @return Sync activity
*
*/
public String syncActivity() {
return this.syncActivity;
}
/**
* @return Total count of persistent files not syncing (combined upload + download).
*
*/
public Double totalPersistentFilesNotSyncingCount() {
return this.totalPersistentFilesNotSyncingCount;
}
/**
* @return Upload sync activity
*
*/
public ServerEndpointSyncActivityStatusResponse uploadActivity() {
return this.uploadActivity;
}
/**
* @return Upload Health Status.
*
*/
public String uploadHealth() {
return this.uploadHealth;
}
/**
* @return Upload Status
*
*/
public ServerEndpointSyncSessionStatusResponse uploadStatus() {
return this.uploadStatus;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ServerEndpointSyncStatusResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private ServerEndpointBackgroundDataDownloadActivityResponse backgroundDataDownloadActivity;
private String combinedHealth;
private ServerEndpointSyncActivityStatusResponse downloadActivity;
private String downloadHealth;
private ServerEndpointSyncSessionStatusResponse downloadStatus;
private String lastUpdatedTimestamp;
private String offlineDataTransferStatus;
private String syncActivity;
private Double totalPersistentFilesNotSyncingCount;
private ServerEndpointSyncActivityStatusResponse uploadActivity;
private String uploadHealth;
private ServerEndpointSyncSessionStatusResponse uploadStatus;
public Builder() {}
public Builder(ServerEndpointSyncStatusResponse defaults) {
Objects.requireNonNull(defaults);
this.backgroundDataDownloadActivity = defaults.backgroundDataDownloadActivity;
this.combinedHealth = defaults.combinedHealth;
this.downloadActivity = defaults.downloadActivity;
this.downloadHealth = defaults.downloadHealth;
this.downloadStatus = defaults.downloadStatus;
this.lastUpdatedTimestamp = defaults.lastUpdatedTimestamp;
this.offlineDataTransferStatus = defaults.offlineDataTransferStatus;
this.syncActivity = defaults.syncActivity;
this.totalPersistentFilesNotSyncingCount = defaults.totalPersistentFilesNotSyncingCount;
this.uploadActivity = defaults.uploadActivity;
this.uploadHealth = defaults.uploadHealth;
this.uploadStatus = defaults.uploadStatus;
}
@CustomType.Setter
public Builder backgroundDataDownloadActivity(ServerEndpointBackgroundDataDownloadActivityResponse backgroundDataDownloadActivity) {
if (backgroundDataDownloadActivity == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "backgroundDataDownloadActivity");
}
this.backgroundDataDownloadActivity = backgroundDataDownloadActivity;
return this;
}
@CustomType.Setter
public Builder combinedHealth(String combinedHealth) {
if (combinedHealth == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "combinedHealth");
}
this.combinedHealth = combinedHealth;
return this;
}
@CustomType.Setter
public Builder downloadActivity(ServerEndpointSyncActivityStatusResponse downloadActivity) {
if (downloadActivity == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "downloadActivity");
}
this.downloadActivity = downloadActivity;
return this;
}
@CustomType.Setter
public Builder downloadHealth(String downloadHealth) {
if (downloadHealth == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "downloadHealth");
}
this.downloadHealth = downloadHealth;
return this;
}
@CustomType.Setter
public Builder downloadStatus(ServerEndpointSyncSessionStatusResponse downloadStatus) {
if (downloadStatus == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "downloadStatus");
}
this.downloadStatus = downloadStatus;
return this;
}
@CustomType.Setter
public Builder lastUpdatedTimestamp(String lastUpdatedTimestamp) {
if (lastUpdatedTimestamp == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "lastUpdatedTimestamp");
}
this.lastUpdatedTimestamp = lastUpdatedTimestamp;
return this;
}
@CustomType.Setter
public Builder offlineDataTransferStatus(String offlineDataTransferStatus) {
if (offlineDataTransferStatus == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "offlineDataTransferStatus");
}
this.offlineDataTransferStatus = offlineDataTransferStatus;
return this;
}
@CustomType.Setter
public Builder syncActivity(String syncActivity) {
if (syncActivity == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "syncActivity");
}
this.syncActivity = syncActivity;
return this;
}
@CustomType.Setter
public Builder totalPersistentFilesNotSyncingCount(Double totalPersistentFilesNotSyncingCount) {
if (totalPersistentFilesNotSyncingCount == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "totalPersistentFilesNotSyncingCount");
}
this.totalPersistentFilesNotSyncingCount = totalPersistentFilesNotSyncingCount;
return this;
}
@CustomType.Setter
public Builder uploadActivity(ServerEndpointSyncActivityStatusResponse uploadActivity) {
if (uploadActivity == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "uploadActivity");
}
this.uploadActivity = uploadActivity;
return this;
}
@CustomType.Setter
public Builder uploadHealth(String uploadHealth) {
if (uploadHealth == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "uploadHealth");
}
this.uploadHealth = uploadHealth;
return this;
}
@CustomType.Setter
public Builder uploadStatus(ServerEndpointSyncSessionStatusResponse uploadStatus) {
if (uploadStatus == null) {
throw new MissingRequiredPropertyException("ServerEndpointSyncStatusResponse", "uploadStatus");
}
this.uploadStatus = uploadStatus;
return this;
}
public ServerEndpointSyncStatusResponse build() {
final var _resultValue = new ServerEndpointSyncStatusResponse();
_resultValue.backgroundDataDownloadActivity = backgroundDataDownloadActivity;
_resultValue.combinedHealth = combinedHealth;
_resultValue.downloadActivity = downloadActivity;
_resultValue.downloadHealth = downloadHealth;
_resultValue.downloadStatus = downloadStatus;
_resultValue.lastUpdatedTimestamp = lastUpdatedTimestamp;
_resultValue.offlineDataTransferStatus = offlineDataTransferStatus;
_resultValue.syncActivity = syncActivity;
_resultValue.totalPersistentFilesNotSyncingCount = totalPersistentFilesNotSyncingCount;
_resultValue.uploadActivity = uploadActivity;
_resultValue.uploadHealth = uploadHealth;
_resultValue.uploadStatus = uploadStatus;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy