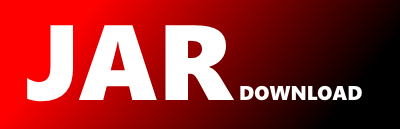
com.pulumi.azurenative.streamanalytics.inputs.AzureSqlReferenceInputDataSourceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.streamanalytics.inputs;
import com.pulumi.azurenative.streamanalytics.enums.RefreshType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes an Azure SQL database reference input data source.
*
*/
public final class AzureSqlReferenceInputDataSourceArgs extends com.pulumi.resources.ResourceArgs {
public static final AzureSqlReferenceInputDataSourceArgs Empty = new AzureSqlReferenceInputDataSourceArgs();
/**
* This element is associated with the datasource element. This is the name of the database that output will be written to.
*
*/
@Import(name="database")
private @Nullable Output database;
/**
* @return This element is associated with the datasource element. This is the name of the database that output will be written to.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy