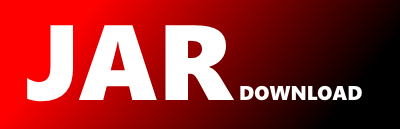
com.pulumi.azurenative.testbase.outputs.DraftPackageIntuneAppMetadataItemResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.testbase.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DraftPackageIntuneAppMetadataItemResponse {
/**
* @return Intune app id.
*
*/
private @Nullable String appId;
/**
* @return Intune app name.
*
*/
private @Nullable String appName;
/**
* @return Creation date of the app.
*
*/
private @Nullable String createDate;
/**
* @return Ids of dependency apps.
*
*/
private @Nullable List dependencyIds;
/**
* @return Count of dependency apps.
*
*/
private @Nullable Integer dependentAppCount;
/**
* @return Description of the app.
*
*/
private @Nullable String description;
/**
* @return Expected exit codes returned from Intune App.
*
*/
private @Nullable List expectedExitCodes;
/**
* @return Install command.
*
*/
private @Nullable String installCommand;
/**
* @return last processed time tickets.
*
*/
private @Nullable Double lastProcessed;
/**
* @return Minimum supported OS. The OS version must be greater than this version to run this app.
*
*/
private @Nullable String minimumSupportedOS;
/**
* @return Owner of the app.
*
*/
private @Nullable String owner;
/**
* @return Publisher of the app.
*
*/
private @Nullable String publisher;
/**
* @return Setup file path.
*
*/
private @Nullable String setupFile;
/**
* @return Extract status.
*
*/
private @Nullable String status;
/**
* @return Uninstall command.
*
*/
private @Nullable String uninstallCommand;
/**
* @return Intune app version.
*
*/
private @Nullable String version;
private DraftPackageIntuneAppMetadataItemResponse() {}
/**
* @return Intune app id.
*
*/
public Optional appId() {
return Optional.ofNullable(this.appId);
}
/**
* @return Intune app name.
*
*/
public Optional appName() {
return Optional.ofNullable(this.appName);
}
/**
* @return Creation date of the app.
*
*/
public Optional createDate() {
return Optional.ofNullable(this.createDate);
}
/**
* @return Ids of dependency apps.
*
*/
public List dependencyIds() {
return this.dependencyIds == null ? List.of() : this.dependencyIds;
}
/**
* @return Count of dependency apps.
*
*/
public Optional dependentAppCount() {
return Optional.ofNullable(this.dependentAppCount);
}
/**
* @return Description of the app.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Expected exit codes returned from Intune App.
*
*/
public List expectedExitCodes() {
return this.expectedExitCodes == null ? List.of() : this.expectedExitCodes;
}
/**
* @return Install command.
*
*/
public Optional installCommand() {
return Optional.ofNullable(this.installCommand);
}
/**
* @return last processed time tickets.
*
*/
public Optional lastProcessed() {
return Optional.ofNullable(this.lastProcessed);
}
/**
* @return Minimum supported OS. The OS version must be greater than this version to run this app.
*
*/
public Optional minimumSupportedOS() {
return Optional.ofNullable(this.minimumSupportedOS);
}
/**
* @return Owner of the app.
*
*/
public Optional owner() {
return Optional.ofNullable(this.owner);
}
/**
* @return Publisher of the app.
*
*/
public Optional publisher() {
return Optional.ofNullable(this.publisher);
}
/**
* @return Setup file path.
*
*/
public Optional setupFile() {
return Optional.ofNullable(this.setupFile);
}
/**
* @return Extract status.
*
*/
public Optional status() {
return Optional.ofNullable(this.status);
}
/**
* @return Uninstall command.
*
*/
public Optional uninstallCommand() {
return Optional.ofNullable(this.uninstallCommand);
}
/**
* @return Intune app version.
*
*/
public Optional version() {
return Optional.ofNullable(this.version);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DraftPackageIntuneAppMetadataItemResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String appId;
private @Nullable String appName;
private @Nullable String createDate;
private @Nullable List dependencyIds;
private @Nullable Integer dependentAppCount;
private @Nullable String description;
private @Nullable List expectedExitCodes;
private @Nullable String installCommand;
private @Nullable Double lastProcessed;
private @Nullable String minimumSupportedOS;
private @Nullable String owner;
private @Nullable String publisher;
private @Nullable String setupFile;
private @Nullable String status;
private @Nullable String uninstallCommand;
private @Nullable String version;
public Builder() {}
public Builder(DraftPackageIntuneAppMetadataItemResponse defaults) {
Objects.requireNonNull(defaults);
this.appId = defaults.appId;
this.appName = defaults.appName;
this.createDate = defaults.createDate;
this.dependencyIds = defaults.dependencyIds;
this.dependentAppCount = defaults.dependentAppCount;
this.description = defaults.description;
this.expectedExitCodes = defaults.expectedExitCodes;
this.installCommand = defaults.installCommand;
this.lastProcessed = defaults.lastProcessed;
this.minimumSupportedOS = defaults.minimumSupportedOS;
this.owner = defaults.owner;
this.publisher = defaults.publisher;
this.setupFile = defaults.setupFile;
this.status = defaults.status;
this.uninstallCommand = defaults.uninstallCommand;
this.version = defaults.version;
}
@CustomType.Setter
public Builder appId(@Nullable String appId) {
this.appId = appId;
return this;
}
@CustomType.Setter
public Builder appName(@Nullable String appName) {
this.appName = appName;
return this;
}
@CustomType.Setter
public Builder createDate(@Nullable String createDate) {
this.createDate = createDate;
return this;
}
@CustomType.Setter
public Builder dependencyIds(@Nullable List dependencyIds) {
this.dependencyIds = dependencyIds;
return this;
}
public Builder dependencyIds(String... dependencyIds) {
return dependencyIds(List.of(dependencyIds));
}
@CustomType.Setter
public Builder dependentAppCount(@Nullable Integer dependentAppCount) {
this.dependentAppCount = dependentAppCount;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder expectedExitCodes(@Nullable List expectedExitCodes) {
this.expectedExitCodes = expectedExitCodes;
return this;
}
public Builder expectedExitCodes(String... expectedExitCodes) {
return expectedExitCodes(List.of(expectedExitCodes));
}
@CustomType.Setter
public Builder installCommand(@Nullable String installCommand) {
this.installCommand = installCommand;
return this;
}
@CustomType.Setter
public Builder lastProcessed(@Nullable Double lastProcessed) {
this.lastProcessed = lastProcessed;
return this;
}
@CustomType.Setter
public Builder minimumSupportedOS(@Nullable String minimumSupportedOS) {
this.minimumSupportedOS = minimumSupportedOS;
return this;
}
@CustomType.Setter
public Builder owner(@Nullable String owner) {
this.owner = owner;
return this;
}
@CustomType.Setter
public Builder publisher(@Nullable String publisher) {
this.publisher = publisher;
return this;
}
@CustomType.Setter
public Builder setupFile(@Nullable String setupFile) {
this.setupFile = setupFile;
return this;
}
@CustomType.Setter
public Builder status(@Nullable String status) {
this.status = status;
return this;
}
@CustomType.Setter
public Builder uninstallCommand(@Nullable String uninstallCommand) {
this.uninstallCommand = uninstallCommand;
return this;
}
@CustomType.Setter
public Builder version(@Nullable String version) {
this.version = version;
return this;
}
public DraftPackageIntuneAppMetadataItemResponse build() {
final var _resultValue = new DraftPackageIntuneAppMetadataItemResponse();
_resultValue.appId = appId;
_resultValue.appName = appName;
_resultValue.createDate = createDate;
_resultValue.dependencyIds = dependencyIds;
_resultValue.dependentAppCount = dependentAppCount;
_resultValue.description = description;
_resultValue.expectedExitCodes = expectedExitCodes;
_resultValue.installCommand = installCommand;
_resultValue.lastProcessed = lastProcessed;
_resultValue.minimumSupportedOS = minimumSupportedOS;
_resultValue.owner = owner;
_resultValue.publisher = publisher;
_resultValue.setupFile = setupFile;
_resultValue.status = status;
_resultValue.uninstallCommand = uninstallCommand;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy