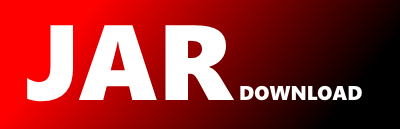
com.pulumi.azurenative.videoanalyzer.LivePipelineArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer;
import com.pulumi.azurenative.videoanalyzer.inputs.ParameterDefinitionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LivePipelineArgs extends com.pulumi.resources.ResourceArgs {
public static final LivePipelineArgs Empty = new LivePipelineArgs();
/**
* The Azure Video Analyzer account name.
*
*/
@Import(name="accountName", required=true)
private Output accountName;
/**
* @return The Azure Video Analyzer account name.
*
*/
public Output accountName() {
return this.accountName;
}
/**
* Maximum bitrate capacity in Kbps reserved for the live pipeline. The allowed range is from 500 to 3000 Kbps in increments of 100 Kbps. If the RTSP camera exceeds this capacity, then the service will disconnect temporarily from the camera. It will retry to re-establish connection (with exponential backoff), checking to see if the camera bitrate is now below the reserved capacity. Doing so will ensure that one 'noisy neighbor' does not affect other live pipelines in your account.
*
*/
@Import(name="bitrateKbps", required=true)
private Output bitrateKbps;
/**
* @return Maximum bitrate capacity in Kbps reserved for the live pipeline. The allowed range is from 500 to 3000 Kbps in increments of 100 Kbps. If the RTSP camera exceeds this capacity, then the service will disconnect temporarily from the camera. It will retry to re-establish connection (with exponential backoff), checking to see if the camera bitrate is now below the reserved capacity. Doing so will ensure that one 'noisy neighbor' does not affect other live pipelines in your account.
*
*/
public Output bitrateKbps() {
return this.bitrateKbps;
}
/**
* An optional description for the pipeline.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return An optional description for the pipeline.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy