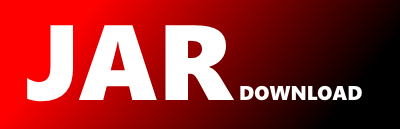
com.pulumi.azurenative.videoanalyzer.inputs.VideoScaleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.inputs;
import com.pulumi.azurenative.videoanalyzer.enums.VideoScaleMode;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The video scaling information.
*
*/
public final class VideoScaleArgs extends com.pulumi.resources.ResourceArgs {
public static final VideoScaleArgs Empty = new VideoScaleArgs();
/**
* The desired output video height.
*
*/
@Import(name="height")
private @Nullable Output height;
/**
* @return The desired output video height.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy