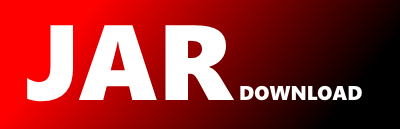
com.pulumi.azurenative.virtualmachineimages.outputs.ImageTemplatePropertiesResponseValidate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.virtualmachineimages.outputs;
import com.pulumi.azurenative.virtualmachineimages.outputs.ImageTemplateFileValidatorResponse;
import com.pulumi.azurenative.virtualmachineimages.outputs.ImageTemplatePowerShellValidatorResponse;
import com.pulumi.azurenative.virtualmachineimages.outputs.ImageTemplateShellValidatorResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Object;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ImageTemplatePropertiesResponseValidate {
/**
* @return If validation fails and this field is set to false, output image(s) will not be distributed. This is the default behavior. If validation fails and this field is set to true, output image(s) will still be distributed. Please use this option with caution as it may result in bad images being distributed for use. In either case (true or false), the end to end image run will be reported as having failed in case of a validation failure. [Note: This field has no effect if validation succeeds.]
*
*/
private @Nullable Boolean continueDistributeOnFailure;
/**
* @return List of validations to be performed.
*
*/
private @Nullable List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy