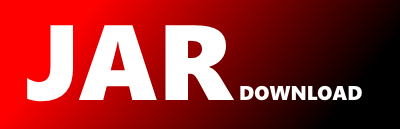
com.pulumi.azurenative.voiceservices.CommunicationsGatewayArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.voiceservices;
import com.pulumi.azurenative.voiceservices.enums.AutoGeneratedDomainNameLabelScope;
import com.pulumi.azurenative.voiceservices.enums.CommunicationsPlatform;
import com.pulumi.azurenative.voiceservices.enums.Connectivity;
import com.pulumi.azurenative.voiceservices.enums.E911Type;
import com.pulumi.azurenative.voiceservices.enums.TeamsCodecs;
import com.pulumi.azurenative.voiceservices.inputs.ManagedServiceIdentityArgs;
import com.pulumi.azurenative.voiceservices.inputs.ServiceRegionPropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CommunicationsGatewayArgs extends com.pulumi.resources.ResourceArgs {
public static final CommunicationsGatewayArgs Empty = new CommunicationsGatewayArgs();
/**
* Details of API bridge functionality, if required
*
*/
@Import(name="apiBridge")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy