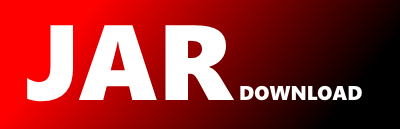
com.pulumi.azurenative.web.AppServicePlanArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web;
import com.pulumi.azurenative.web.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.web.inputs.HostingEnvironmentProfileArgs;
import com.pulumi.azurenative.web.inputs.KubeEnvironmentProfileArgs;
import com.pulumi.azurenative.web.inputs.SkuDescriptionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AppServicePlanArgs extends com.pulumi.resources.ResourceArgs {
public static final AppServicePlanArgs Empty = new AppServicePlanArgs();
/**
* ServerFarm supports ElasticScale. Apps in this plan will scale as if the ServerFarm was ElasticPremium sku
*
*/
@Import(name="elasticScaleEnabled")
private @Nullable Output elasticScaleEnabled;
/**
* @return ServerFarm supports ElasticScale. Apps in this plan will scale as if the ServerFarm was ElasticPremium sku
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy