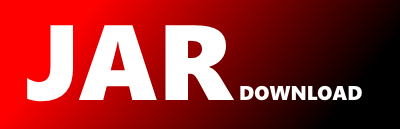
com.pulumi.azurenative.web.inputs.StaticSiteBuildPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Build properties for the static site.
*
*/
public final class StaticSiteBuildPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final StaticSiteBuildPropertiesArgs Empty = new StaticSiteBuildPropertiesArgs();
/**
* A custom command to run during deployment of the Azure Functions API application.
*
*/
@Import(name="apiBuildCommand")
private @Nullable Output apiBuildCommand;
/**
* @return A custom command to run during deployment of the Azure Functions API application.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy