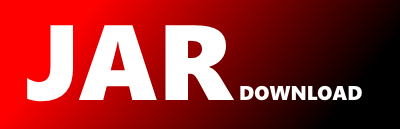
com.pulumi.azurenative.apimanagement.Product Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.apimanagement.ProductArgs;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Product details.
* Azure REST API version: 2022-08-01. Prior API version in Azure Native 1.x: 2020-12-01.
*
* Other available API versions: 2016-07-07, 2016-10-10, 2022-09-01-preview, 2023-03-01-preview, 2023-05-01-preview, 2023-09-01-preview, 2024-05-01.
*
* ## Example Usage
* ### ApiManagementCreateProduct
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.apimanagement.Product;
* import com.pulumi.azurenative.apimanagement.ProductArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var product = new Product("product", ProductArgs.builder()
* .displayName("Test Template ProductName 4")
* .productId("testproduct")
* .resourceGroupName("rg1")
* .serviceName("apimService1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:apimanagement:Product testproduct /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ApiManagement/service/{serviceName}/products/{productId}
* ```
*
*/
@ResourceType(type="azure-native:apimanagement:Product")
public class Product extends com.pulumi.resources.CustomResource {
/**
* whether subscription approval is required. If false, new subscriptions will be approved automatically enabling developers to call the product’s APIs immediately after subscribing. If true, administrators must manually approve the subscription before the developer can any of the product’s APIs. Can be present only if subscriptionRequired property is present and has a value of false.
*
*/
@Export(name="approvalRequired", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> approvalRequired;
/**
* @return whether subscription approval is required. If false, new subscriptions will be approved automatically enabling developers to call the product’s APIs immediately after subscribing. If true, administrators must manually approve the subscription before the developer can any of the product’s APIs. Can be present only if subscriptionRequired property is present and has a value of false.
*
*/
public Output> approvalRequired() {
return Codegen.optional(this.approvalRequired);
}
/**
* Product description. May include HTML formatting tags.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Product description. May include HTML formatting tags.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Product name.
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output displayName;
/**
* @return Product name.
*
*/
public Output displayName() {
return this.displayName;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* whether product is published or not. Published products are discoverable by users of developer portal. Non published products are visible only to administrators. Default state of Product is notPublished.
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> state;
/**
* @return whether product is published or not. Published products are discoverable by users of developer portal. Non published products are visible only to administrators. Default state of Product is notPublished.
*
*/
public Output> state() {
return Codegen.optional(this.state);
}
/**
* Whether a product subscription is required for accessing APIs included in this product. If true, the product is referred to as "protected" and a valid subscription key is required for a request to an API included in the product to succeed. If false, the product is referred to as "open" and requests to an API included in the product can be made without a subscription key. If property is omitted when creating a new product it's value is assumed to be true.
*
*/
@Export(name="subscriptionRequired", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> subscriptionRequired;
/**
* @return Whether a product subscription is required for accessing APIs included in this product. If true, the product is referred to as "protected" and a valid subscription key is required for a request to an API included in the product to succeed. If false, the product is referred to as "open" and requests to an API included in the product can be made without a subscription key. If property is omitted when creating a new product it's value is assumed to be true.
*
*/
public Output> subscriptionRequired() {
return Codegen.optional(this.subscriptionRequired);
}
/**
* Whether the number of subscriptions a user can have to this product at the same time. Set to null or omit to allow unlimited per user subscriptions. Can be present only if subscriptionRequired property is present and has a value of false.
*
*/
@Export(name="subscriptionsLimit", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> subscriptionsLimit;
/**
* @return Whether the number of subscriptions a user can have to this product at the same time. Set to null or omit to allow unlimited per user subscriptions. Can be present only if subscriptionRequired property is present and has a value of false.
*
*/
public Output> subscriptionsLimit() {
return Codegen.optional(this.subscriptionsLimit);
}
/**
* Product terms of use. Developers trying to subscribe to the product will be presented and required to accept these terms before they can complete the subscription process.
*
*/
@Export(name="terms", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> terms;
/**
* @return Product terms of use. Developers trying to subscribe to the product will be presented and required to accept these terms before they can complete the subscription process.
*
*/
public Output> terms() {
return Codegen.optional(this.terms);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Product(java.lang.String name) {
this(name, ProductArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Product(java.lang.String name, ProductArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Product(java.lang.String name, ProductArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:apimanagement:Product", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Product(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:apimanagement:Product", name, null, makeResourceOptions(options, id), false);
}
private static ProductArgs makeArgs(ProductArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ProductArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:apimanagement/v20160707:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20161010:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20170301:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20180101:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20180601preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20190101:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20191201:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20191201preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20200601preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20201201:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20210101preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20210401preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20210801:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20211201preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20220401preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20220801:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20220901preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20230301preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20230501preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20230901preview:Product").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20240501:Product").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Product get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Product(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy