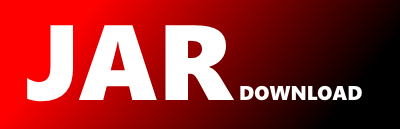
com.pulumi.azurenative.apimanagement.UserArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement;
import com.pulumi.azurenative.apimanagement.enums.AppType;
import com.pulumi.azurenative.apimanagement.enums.Confirmation;
import com.pulumi.azurenative.apimanagement.enums.UserState;
import com.pulumi.azurenative.apimanagement.inputs.UserIdentityContractArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UserArgs extends com.pulumi.resources.ResourceArgs {
public static final UserArgs Empty = new UserArgs();
/**
* Determines the type of application which send the create user request. Default is legacy portal.
*
*/
@Import(name="appType")
private @Nullable Output> appType;
/**
* @return Determines the type of application which send the create user request. Default is legacy portal.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy