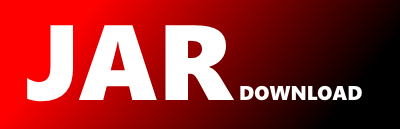
com.pulumi.azurenative.app.ContainerApp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.app.ContainerAppArgs;
import com.pulumi.azurenative.app.outputs.ConfigurationResponse;
import com.pulumi.azurenative.app.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.app.outputs.ManagedServiceIdentityResponse;
import com.pulumi.azurenative.app.outputs.SystemDataResponse;
import com.pulumi.azurenative.app.outputs.TemplateResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Container App.
* Azure REST API version: 2022-10-01. Prior API version in Azure Native 1.x: 2022-03-01.
*
* Other available API versions: 2022-01-01-preview, 2023-04-01-preview, 2023-05-01, 2023-05-02-preview, 2023-08-01-preview, 2023-11-02-preview, 2024-02-02-preview, 2024-03-01, 2024-08-02-preview.
*
* **Note**: the current default Azure API version for this resource, 2022-10-01, has an issue with referencing Key Vault secrets via the `KeyVaultUrl` property. If you encounter the error _"invalid: value or keyVaultUrl and identity should be provided"_ with such a configuration, you can use API version 2023-05-1 instead. In v3 of this provider, we will update the default API version.
*
* ## Example Usage
* ### Create or Update Container App
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.app.ContainerApp;
* import com.pulumi.azurenative.app.ContainerAppArgs;
* import com.pulumi.azurenative.app.inputs.ConfigurationArgs;
* import com.pulumi.azurenative.app.inputs.DaprArgs;
* import com.pulumi.azurenative.app.inputs.IngressArgs;
* import com.pulumi.azurenative.app.inputs.CorsPolicyArgs;
* import com.pulumi.azurenative.app.inputs.TemplateArgs;
* import com.pulumi.azurenative.app.inputs.ScaleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var containerApp = new ContainerApp("containerApp", ContainerAppArgs.builder()
* .configuration(ConfigurationArgs.builder()
* .dapr(DaprArgs.builder()
* .appPort(3000)
* .appProtocol("http")
* .enableApiLogging(true)
* .enabled(true)
* .httpMaxRequestSize(10)
* .httpReadBufferSize(30)
* .logLevel("debug")
* .build())
* .ingress(IngressArgs.builder()
* .clientCertificateMode("accept")
* .corsPolicy(CorsPolicyArgs.builder()
* .allowCredentials(true)
* .allowedHeaders(
* "HEADER1",
* "HEADER2")
* .allowedMethods(
* "GET",
* "POST")
* .allowedOrigins(
* "https://a.test.com",
* "https://b.test.com")
* .exposeHeaders(
* "HEADER3",
* "HEADER4")
* .maxAge(1234)
* .build())
* .customDomains(
* CustomDomainArgs.builder()
* .bindingType("SniEnabled")
* .certificateId("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/rg/providers/Microsoft.App/managedEnvironments/demokube/certificates/my-certificate-for-my-name-dot-com")
* .name("www.my-name.com")
* .build(),
* CustomDomainArgs.builder()
* .bindingType("SniEnabled")
* .certificateId("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/rg/providers/Microsoft.App/managedEnvironments/demokube/certificates/my-certificate-for-my-other-name-dot-com")
* .name("www.my-other-name.com")
* .build())
* .external(true)
* .ipSecurityRestrictions(
* IpSecurityRestrictionRuleArgs.builder()
* .action("Allow")
* .description("Allowing all IP's within the subnet below to access containerapp")
* .ipAddressRange("192.168.1.1/32")
* .name("Allow work IP A subnet")
* .build(),
* IpSecurityRestrictionRuleArgs.builder()
* .action("Allow")
* .description("Allowing all IP's within the subnet below to access containerapp")
* .ipAddressRange("192.168.1.1/8")
* .name("Allow work IP B subnet")
* .build())
* .targetPort(3000)
* .traffic(TrafficWeightArgs.builder()
* .label("production")
* .revisionName("testcontainerapp0-ab1234")
* .weight(100)
* .build())
* .build())
* .maxInactiveRevisions(10)
* .build())
* .containerAppName("testcontainerapp0")
* .environmentId("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/rg/providers/Microsoft.App/managedEnvironments/demokube")
* .location("East US")
* .resourceGroupName("rg")
* .template(TemplateArgs.builder()
* .containers(ContainerArgs.builder()
* .image("repo/testcontainerapp0:v1")
* .name("testcontainerapp0")
* .probes(ContainerAppProbeArgs.builder()
* .httpGet(ContainerAppProbeHttpGetArgs.builder()
* .httpHeaders(ContainerAppProbeHttpHeadersArgs.builder()
* .name("Custom-Header")
* .value("Awesome")
* .build())
* .path("/health")
* .port(8080)
* .build())
* .initialDelaySeconds(3)
* .periodSeconds(3)
* .type("Liveness")
* .build())
* .build())
* .initContainers(InitContainerArgs.builder()
* .args(
* "-c",
* "while true; do echo hello; sleep 10;done")
* .command("/bin/sh")
* .image("repo/testcontainerapp0:v4")
* .name("testinitcontainerApp0")
* .resources(ContainerResourcesArgs.builder()
* .cpu(0.5)
* .memory("1Gi")
* .build())
* .build())
* .scale(ScaleArgs.builder()
* .maxReplicas(5)
* .minReplicas(1)
* .rules(ScaleRuleArgs.builder()
* .custom(CustomScaleRuleArgs.builder()
* .metadata(Map.of("concurrentRequests", "50"))
* .type("http")
* .build())
* .name("httpscalingrule")
* .build())
* .build())
* .build())
* .workloadProfileType("GeneralPurpose")
* .build());
*
* }
* }
*
* }
*
* ### Create or Update Tcp App
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.app.ContainerApp;
* import com.pulumi.azurenative.app.ContainerAppArgs;
* import com.pulumi.azurenative.app.inputs.ConfigurationArgs;
* import com.pulumi.azurenative.app.inputs.IngressArgs;
* import com.pulumi.azurenative.app.inputs.TemplateArgs;
* import com.pulumi.azurenative.app.inputs.ScaleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var containerApp = new ContainerApp("containerApp", ContainerAppArgs.builder()
* .configuration(ConfigurationArgs.builder()
* .ingress(IngressArgs.builder()
* .exposedPort(4000)
* .external(true)
* .targetPort(3000)
* .traffic(TrafficWeightArgs.builder()
* .revisionName("testcontainerapptcp-ab1234")
* .weight(100)
* .build())
* .transport("tcp")
* .build())
* .build())
* .containerAppName("testcontainerapptcp")
* .environmentId("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/rg/providers/Microsoft.App/managedEnvironments/demokube")
* .location("East US")
* .resourceGroupName("rg")
* .template(TemplateArgs.builder()
* .containers(ContainerArgs.builder()
* .image("repo/testcontainerapptcp:v1")
* .name("testcontainerapptcp")
* .probes(ContainerAppProbeArgs.builder()
* .initialDelaySeconds(3)
* .periodSeconds(3)
* .tcpSocket(ContainerAppProbeTcpSocketArgs.builder()
* .port(8080)
* .build())
* .type("Liveness")
* .build())
* .build())
* .scale(ScaleArgs.builder()
* .maxReplicas(5)
* .minReplicas(1)
* .rules(ScaleRuleArgs.builder()
* .name("tcpscalingrule")
* .tcp(TcpScaleRuleArgs.builder()
* .metadata(Map.of("concurrentConnections", "50"))
* .build())
* .build())
* .build())
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:app:ContainerApp testcontainerapptcp /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.App/containerApps/{containerAppName}
* ```
*
*/
@ResourceType(type="azure-native:app:ContainerApp")
public class ContainerApp extends com.pulumi.resources.CustomResource {
/**
* Non versioned Container App configuration properties.
*
*/
@Export(name="configuration", refs={ConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ ConfigurationResponse> configuration;
/**
* @return Non versioned Container App configuration properties.
*
*/
public Output> configuration() {
return Codegen.optional(this.configuration);
}
/**
* Id used to verify domain name ownership
*
*/
@Export(name="customDomainVerificationId", refs={String.class}, tree="[0]")
private Output customDomainVerificationId;
/**
* @return Id used to verify domain name ownership
*
*/
public Output customDomainVerificationId() {
return this.customDomainVerificationId;
}
/**
* Resource ID of environment.
*
*/
@Export(name="environmentId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> environmentId;
/**
* @return Resource ID of environment.
*
*/
public Output> environmentId() {
return Codegen.optional(this.environmentId);
}
/**
* The endpoint of the eventstream of the container app.
*
*/
@Export(name="eventStreamEndpoint", refs={String.class}, tree="[0]")
private Output eventStreamEndpoint;
/**
* @return The endpoint of the eventstream of the container app.
*
*/
public Output eventStreamEndpoint() {
return this.eventStreamEndpoint;
}
/**
* The complex type of the extended location.
*
*/
@Export(name="extendedLocation", refs={ExtendedLocationResponse.class}, tree="[0]")
private Output* @Nullable */ ExtendedLocationResponse> extendedLocation;
/**
* @return The complex type of the extended location.
*
*/
public Output> extendedLocation() {
return Codegen.optional(this.extendedLocation);
}
/**
* managed identities for the Container App to interact with other Azure services without maintaining any secrets or credentials in code.
*
*/
@Export(name="identity", refs={ManagedServiceIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ ManagedServiceIdentityResponse> identity;
/**
* @return managed identities for the Container App to interact with other Azure services without maintaining any secrets or credentials in code.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* Name of the latest ready revision of the Container App.
*
*/
@Export(name="latestReadyRevisionName", refs={String.class}, tree="[0]")
private Output latestReadyRevisionName;
/**
* @return Name of the latest ready revision of the Container App.
*
*/
public Output latestReadyRevisionName() {
return this.latestReadyRevisionName;
}
/**
* Fully Qualified Domain Name of the latest revision of the Container App.
*
*/
@Export(name="latestRevisionFqdn", refs={String.class}, tree="[0]")
private Output latestRevisionFqdn;
/**
* @return Fully Qualified Domain Name of the latest revision of the Container App.
*
*/
public Output latestRevisionFqdn() {
return this.latestRevisionFqdn;
}
/**
* Name of the latest revision of the Container App.
*
*/
@Export(name="latestRevisionName", refs={String.class}, tree="[0]")
private Output latestRevisionName;
/**
* @return Name of the latest revision of the Container App.
*
*/
public Output latestRevisionName() {
return this.latestRevisionName;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* Deprecated. Resource ID of the Container App's environment.
*
*/
@Export(name="managedEnvironmentId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> managedEnvironmentId;
/**
* @return Deprecated. Resource ID of the Container App's environment.
*
*/
public Output> managedEnvironmentId() {
return Codegen.optional(this.managedEnvironmentId);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Outbound IP Addresses for container app.
*
*/
@Export(name="outboundIpAddresses", refs={List.class,String.class}, tree="[0,1]")
private Output> outboundIpAddresses;
/**
* @return Outbound IP Addresses for container app.
*
*/
public Output> outboundIpAddresses() {
return this.outboundIpAddresses;
}
/**
* Provisioning state of the Container App.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state of the Container App.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Container App versioned application definition.
*
*/
@Export(name="template", refs={TemplateResponse.class}, tree="[0]")
private Output* @Nullable */ TemplateResponse> template;
/**
* @return Container App versioned application definition.
*
*/
public Output> template() {
return Codegen.optional(this.template);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* Workload profile type to pin for container app execution.
*
*/
@Export(name="workloadProfileType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> workloadProfileType;
/**
* @return Workload profile type to pin for container app execution.
*
*/
public Output> workloadProfileType() {
return Codegen.optional(this.workloadProfileType);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ContainerApp(java.lang.String name) {
this(name, ContainerAppArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ContainerApp(java.lang.String name, ContainerAppArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ContainerApp(java.lang.String name, ContainerAppArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:app:ContainerApp", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ContainerApp(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:app:ContainerApp", name, null, makeResourceOptions(options, id), false);
}
private static ContainerAppArgs makeArgs(ContainerAppArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ContainerAppArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:app/v20220101preview:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20220301:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20220601preview:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20221001:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20221101preview:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20230401preview:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20230501:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20230502preview:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20230801preview:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20231102preview:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20240202preview:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20240301:ContainerApp").build()),
Output.of(Alias.builder().type("azure-native:app/v20240802preview:ContainerApp").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ContainerApp get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ContainerApp(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy