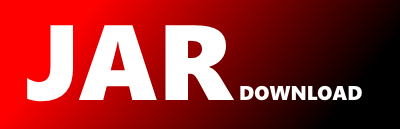
com.pulumi.azurenative.app.outputs.ContainerAppProbeResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.outputs;
import com.pulumi.azurenative.app.outputs.ContainerAppProbeResponseHttpGet;
import com.pulumi.azurenative.app.outputs.ContainerAppProbeResponseTcpSocket;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ContainerAppProbeResponse {
/**
* @return Minimum consecutive failures for the probe to be considered failed after having succeeded. Defaults to 3. Minimum value is 1. Maximum value is 10.
*
*/
private @Nullable Integer failureThreshold;
/**
* @return HTTPGet specifies the http request to perform.
*
*/
private @Nullable ContainerAppProbeResponseHttpGet httpGet;
/**
* @return Number of seconds after the container has started before liveness probes are initiated. Minimum value is 1. Maximum value is 60.
*
*/
private @Nullable Integer initialDelaySeconds;
/**
* @return How often (in seconds) to perform the probe. Default to 10 seconds. Minimum value is 1. Maximum value is 240.
*
*/
private @Nullable Integer periodSeconds;
/**
* @return Minimum consecutive successes for the probe to be considered successful after having failed. Defaults to 1. Must be 1 for liveness and startup. Minimum value is 1. Maximum value is 10.
*
*/
private @Nullable Integer successThreshold;
/**
* @return TCPSocket specifies an action involving a TCP port. TCP hooks not yet supported.
*
*/
private @Nullable ContainerAppProbeResponseTcpSocket tcpSocket;
/**
* @return Optional duration in seconds the pod needs to terminate gracefully upon probe failure. The grace period is the duration in seconds after the processes running in the pod are sent a termination signal and the time when the processes are forcibly halted with a kill signal. Set this value longer than the expected cleanup time for your process. If this value is nil, the pod's terminationGracePeriodSeconds will be used. Otherwise, this value overrides the value provided by the pod spec. Value must be non-negative integer. The value zero indicates stop immediately via the kill signal (no opportunity to shut down). This is an alpha field and requires enabling ProbeTerminationGracePeriod feature gate. Maximum value is 3600 seconds (1 hour)
*
*/
private @Nullable Double terminationGracePeriodSeconds;
/**
* @return Number of seconds after which the probe times out. Defaults to 1 second. Minimum value is 1. Maximum value is 240.
*
*/
private @Nullable Integer timeoutSeconds;
/**
* @return The type of probe.
*
*/
private @Nullable String type;
private ContainerAppProbeResponse() {}
/**
* @return Minimum consecutive failures for the probe to be considered failed after having succeeded. Defaults to 3. Minimum value is 1. Maximum value is 10.
*
*/
public Optional failureThreshold() {
return Optional.ofNullable(this.failureThreshold);
}
/**
* @return HTTPGet specifies the http request to perform.
*
*/
public Optional httpGet() {
return Optional.ofNullable(this.httpGet);
}
/**
* @return Number of seconds after the container has started before liveness probes are initiated. Minimum value is 1. Maximum value is 60.
*
*/
public Optional initialDelaySeconds() {
return Optional.ofNullable(this.initialDelaySeconds);
}
/**
* @return How often (in seconds) to perform the probe. Default to 10 seconds. Minimum value is 1. Maximum value is 240.
*
*/
public Optional periodSeconds() {
return Optional.ofNullable(this.periodSeconds);
}
/**
* @return Minimum consecutive successes for the probe to be considered successful after having failed. Defaults to 1. Must be 1 for liveness and startup. Minimum value is 1. Maximum value is 10.
*
*/
public Optional successThreshold() {
return Optional.ofNullable(this.successThreshold);
}
/**
* @return TCPSocket specifies an action involving a TCP port. TCP hooks not yet supported.
*
*/
public Optional tcpSocket() {
return Optional.ofNullable(this.tcpSocket);
}
/**
* @return Optional duration in seconds the pod needs to terminate gracefully upon probe failure. The grace period is the duration in seconds after the processes running in the pod are sent a termination signal and the time when the processes are forcibly halted with a kill signal. Set this value longer than the expected cleanup time for your process. If this value is nil, the pod's terminationGracePeriodSeconds will be used. Otherwise, this value overrides the value provided by the pod spec. Value must be non-negative integer. The value zero indicates stop immediately via the kill signal (no opportunity to shut down). This is an alpha field and requires enabling ProbeTerminationGracePeriod feature gate. Maximum value is 3600 seconds (1 hour)
*
*/
public Optional terminationGracePeriodSeconds() {
return Optional.ofNullable(this.terminationGracePeriodSeconds);
}
/**
* @return Number of seconds after which the probe times out. Defaults to 1 second. Minimum value is 1. Maximum value is 240.
*
*/
public Optional timeoutSeconds() {
return Optional.ofNullable(this.timeoutSeconds);
}
/**
* @return The type of probe.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ContainerAppProbeResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer failureThreshold;
private @Nullable ContainerAppProbeResponseHttpGet httpGet;
private @Nullable Integer initialDelaySeconds;
private @Nullable Integer periodSeconds;
private @Nullable Integer successThreshold;
private @Nullable ContainerAppProbeResponseTcpSocket tcpSocket;
private @Nullable Double terminationGracePeriodSeconds;
private @Nullable Integer timeoutSeconds;
private @Nullable String type;
public Builder() {}
public Builder(ContainerAppProbeResponse defaults) {
Objects.requireNonNull(defaults);
this.failureThreshold = defaults.failureThreshold;
this.httpGet = defaults.httpGet;
this.initialDelaySeconds = defaults.initialDelaySeconds;
this.periodSeconds = defaults.periodSeconds;
this.successThreshold = defaults.successThreshold;
this.tcpSocket = defaults.tcpSocket;
this.terminationGracePeriodSeconds = defaults.terminationGracePeriodSeconds;
this.timeoutSeconds = defaults.timeoutSeconds;
this.type = defaults.type;
}
@CustomType.Setter
public Builder failureThreshold(@Nullable Integer failureThreshold) {
this.failureThreshold = failureThreshold;
return this;
}
@CustomType.Setter
public Builder httpGet(@Nullable ContainerAppProbeResponseHttpGet httpGet) {
this.httpGet = httpGet;
return this;
}
@CustomType.Setter
public Builder initialDelaySeconds(@Nullable Integer initialDelaySeconds) {
this.initialDelaySeconds = initialDelaySeconds;
return this;
}
@CustomType.Setter
public Builder periodSeconds(@Nullable Integer periodSeconds) {
this.periodSeconds = periodSeconds;
return this;
}
@CustomType.Setter
public Builder successThreshold(@Nullable Integer successThreshold) {
this.successThreshold = successThreshold;
return this;
}
@CustomType.Setter
public Builder tcpSocket(@Nullable ContainerAppProbeResponseTcpSocket tcpSocket) {
this.tcpSocket = tcpSocket;
return this;
}
@CustomType.Setter
public Builder terminationGracePeriodSeconds(@Nullable Double terminationGracePeriodSeconds) {
this.terminationGracePeriodSeconds = terminationGracePeriodSeconds;
return this;
}
@CustomType.Setter
public Builder timeoutSeconds(@Nullable Integer timeoutSeconds) {
this.timeoutSeconds = timeoutSeconds;
return this;
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
public ContainerAppProbeResponse build() {
final var _resultValue = new ContainerAppProbeResponse();
_resultValue.failureThreshold = failureThreshold;
_resultValue.httpGet = httpGet;
_resultValue.initialDelaySeconds = initialDelaySeconds;
_resultValue.periodSeconds = periodSeconds;
_resultValue.successThreshold = successThreshold;
_resultValue.tcpSocket = tcpSocket;
_resultValue.terminationGracePeriodSeconds = terminationGracePeriodSeconds;
_resultValue.timeoutSeconds = timeoutSeconds;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy