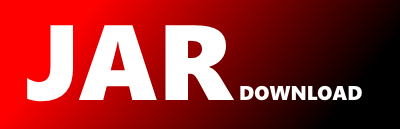
com.pulumi.azurenative.appplatform.inputs.AppResourcePropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.appplatform.inputs;
import com.pulumi.azurenative.appplatform.inputs.AppVNetAddonsArgs;
import com.pulumi.azurenative.appplatform.inputs.CustomPersistentDiskResourceArgs;
import com.pulumi.azurenative.appplatform.inputs.IngressSettingsArgs;
import com.pulumi.azurenative.appplatform.inputs.LoadedCertificateArgs;
import com.pulumi.azurenative.appplatform.inputs.PersistentDiskArgs;
import com.pulumi.azurenative.appplatform.inputs.SecretArgs;
import com.pulumi.azurenative.appplatform.inputs.TemporaryDiskArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* App resource properties payload
*
*/
public final class AppResourcePropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final AppResourcePropertiesArgs Empty = new AppResourcePropertiesArgs();
/**
* Collection of addons
*
*/
@Import(name="addonConfigs")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy