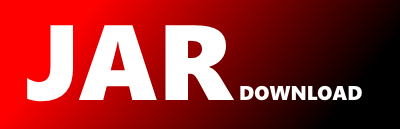
com.pulumi.azurenative.automation.inputs.SUCSchedulePropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.automation.inputs;
import com.pulumi.azurenative.automation.enums.ScheduleFrequency;
import com.pulumi.azurenative.automation.inputs.AdvancedScheduleArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Definition of schedule parameters.
*
*/
public final class SUCSchedulePropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final SUCSchedulePropertiesArgs Empty = new SUCSchedulePropertiesArgs();
/**
* Gets or sets the advanced schedule.
*
*/
@Import(name="advancedSchedule")
private @Nullable Output advancedSchedule;
/**
* @return Gets or sets the advanced schedule.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy