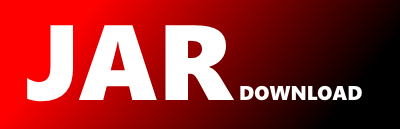
com.pulumi.azurenative.avs.PrivateCloud Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.avs;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.avs.PrivateCloudArgs;
import com.pulumi.azurenative.avs.outputs.AvailabilityPropertiesResponse;
import com.pulumi.azurenative.avs.outputs.CircuitResponse;
import com.pulumi.azurenative.avs.outputs.EncryptionResponse;
import com.pulumi.azurenative.avs.outputs.EndpointsResponse;
import com.pulumi.azurenative.avs.outputs.IdentitySourceResponse;
import com.pulumi.azurenative.avs.outputs.ManagementClusterResponse;
import com.pulumi.azurenative.avs.outputs.PrivateCloudIdentityResponse;
import com.pulumi.azurenative.avs.outputs.SkuResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A private cloud resource
* Azure REST API version: 2022-05-01. Prior API version in Azure Native 1.x: 2020-03-20.
*
* Other available API versions: 2023-03-01, 2023-09-01.
*
* ## Example Usage
* ### PrivateClouds_CreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.avs.PrivateCloud;
* import com.pulumi.azurenative.avs.PrivateCloudArgs;
* import com.pulumi.azurenative.avs.inputs.PrivateCloudIdentityArgs;
* import com.pulumi.azurenative.avs.inputs.ManagementClusterArgs;
* import com.pulumi.azurenative.avs.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var privateCloud = new PrivateCloud("privateCloud", PrivateCloudArgs.builder()
* .identity(PrivateCloudIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .location("eastus2")
* .managementCluster(ManagementClusterArgs.builder()
* .clusterSize(4)
* .build())
* .networkBlock("192.168.48.0/22")
* .privateCloudName("cloud1")
* .resourceGroupName("group1")
* .sku(SkuArgs.builder()
* .name("AV36")
* .build())
* .tags()
* .build());
*
* }
* }
*
* }
*
* ### PrivateClouds_CreateOrUpdate_Stretched
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.avs.PrivateCloud;
* import com.pulumi.azurenative.avs.PrivateCloudArgs;
* import com.pulumi.azurenative.avs.inputs.AvailabilityPropertiesArgs;
* import com.pulumi.azurenative.avs.inputs.ManagementClusterArgs;
* import com.pulumi.azurenative.avs.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var privateCloud = new PrivateCloud("privateCloud", PrivateCloudArgs.builder()
* .availability(AvailabilityPropertiesArgs.builder()
* .secondaryZone(2)
* .strategy("DualZone")
* .zone(1)
* .build())
* .location("eastus2")
* .managementCluster(ManagementClusterArgs.builder()
* .clusterSize(4)
* .build())
* .networkBlock("192.168.48.0/22")
* .privateCloudName("cloud1")
* .resourceGroupName("group1")
* .sku(SkuArgs.builder()
* .name("AV36")
* .build())
* .tags()
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:avs:PrivateCloud cloud1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.AVS/privateClouds/{privateCloudName}
* ```
*
*/
@ResourceType(type="azure-native:avs:PrivateCloud")
public class PrivateCloud extends com.pulumi.resources.CustomResource {
/**
* Properties describing how the cloud is distributed across availability zones
*
*/
@Export(name="availability", refs={AvailabilityPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ AvailabilityPropertiesResponse> availability;
/**
* @return Properties describing how the cloud is distributed across availability zones
*
*/
public Output> availability() {
return Codegen.optional(this.availability);
}
/**
* An ExpressRoute Circuit
*
*/
@Export(name="circuit", refs={CircuitResponse.class}, tree="[0]")
private Output* @Nullable */ CircuitResponse> circuit;
/**
* @return An ExpressRoute Circuit
*
*/
public Output> circuit() {
return Codegen.optional(this.circuit);
}
/**
* Customer managed key encryption, can be enabled or disabled
*
*/
@Export(name="encryption", refs={EncryptionResponse.class}, tree="[0]")
private Output* @Nullable */ EncryptionResponse> encryption;
/**
* @return Customer managed key encryption, can be enabled or disabled
*
*/
public Output> encryption() {
return Codegen.optional(this.encryption);
}
/**
* The endpoints
*
*/
@Export(name="endpoints", refs={EndpointsResponse.class}, tree="[0]")
private Output endpoints;
/**
* @return The endpoints
*
*/
public Output endpoints() {
return this.endpoints;
}
/**
* Array of cloud link IDs from other clouds that connect to this one
*
*/
@Export(name="externalCloudLinks", refs={List.class,String.class}, tree="[0,1]")
private Output> externalCloudLinks;
/**
* @return Array of cloud link IDs from other clouds that connect to this one
*
*/
public Output> externalCloudLinks() {
return this.externalCloudLinks;
}
/**
* The identity of the private cloud, if configured.
*
*/
@Export(name="identity", refs={PrivateCloudIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ PrivateCloudIdentityResponse> identity;
/**
* @return The identity of the private cloud, if configured.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* vCenter Single Sign On Identity Sources
*
*/
@Export(name="identitySources", refs={List.class,IdentitySourceResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> identitySources;
/**
* @return vCenter Single Sign On Identity Sources
*
*/
public Output>> identitySources() {
return Codegen.optional(this.identitySources);
}
/**
* Connectivity to internet is enabled or disabled
*
*/
@Export(name="internet", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> internet;
/**
* @return Connectivity to internet is enabled or disabled
*
*/
public Output> internet() {
return Codegen.optional(this.internet);
}
/**
* Resource location
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource location
*
*/
public Output location() {
return this.location;
}
/**
* The default cluster used for management
*
*/
@Export(name="managementCluster", refs={ManagementClusterResponse.class}, tree="[0]")
private Output managementCluster;
/**
* @return The default cluster used for management
*
*/
public Output managementCluster() {
return this.managementCluster;
}
/**
* Network used to access vCenter Server and NSX-T Manager
*
*/
@Export(name="managementNetwork", refs={String.class}, tree="[0]")
private Output managementNetwork;
/**
* @return Network used to access vCenter Server and NSX-T Manager
*
*/
public Output managementNetwork() {
return this.managementNetwork;
}
/**
* Resource name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name.
*
*/
public Output name() {
return this.name;
}
/**
* The block of addresses should be unique across VNet in your subscription as well as on-premise. Make sure the CIDR format is conformed to (A.B.C.D/X) where A,B,C,D are between 0 and 255, and X is between 0 and 22
*
*/
@Export(name="networkBlock", refs={String.class}, tree="[0]")
private Output networkBlock;
/**
* @return The block of addresses should be unique across VNet in your subscription as well as on-premise. Make sure the CIDR format is conformed to (A.B.C.D/X) where A,B,C,D are between 0 and 255, and X is between 0 and 22
*
*/
public Output networkBlock() {
return this.networkBlock;
}
/**
* Flag to indicate whether the private cloud has the quota for provisioned NSX Public IP count raised from 64 to 1024
*
*/
@Export(name="nsxPublicIpQuotaRaised", refs={String.class}, tree="[0]")
private Output nsxPublicIpQuotaRaised;
/**
* @return Flag to indicate whether the private cloud has the quota for provisioned NSX Public IP count raised from 64 to 1024
*
*/
public Output nsxPublicIpQuotaRaised() {
return this.nsxPublicIpQuotaRaised;
}
/**
* Thumbprint of the NSX-T Manager SSL certificate
*
*/
@Export(name="nsxtCertificateThumbprint", refs={String.class}, tree="[0]")
private Output nsxtCertificateThumbprint;
/**
* @return Thumbprint of the NSX-T Manager SSL certificate
*
*/
public Output nsxtCertificateThumbprint() {
return this.nsxtCertificateThumbprint;
}
/**
* Optionally, set the NSX-T Manager password when the private cloud is created
*
*/
@Export(name="nsxtPassword", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> nsxtPassword;
/**
* @return Optionally, set the NSX-T Manager password when the private cloud is created
*
*/
public Output> nsxtPassword() {
return Codegen.optional(this.nsxtPassword);
}
/**
* Used for virtual machine cold migration, cloning, and snapshot migration
*
*/
@Export(name="provisioningNetwork", refs={String.class}, tree="[0]")
private Output provisioningNetwork;
/**
* @return Used for virtual machine cold migration, cloning, and snapshot migration
*
*/
public Output provisioningNetwork() {
return this.provisioningNetwork;
}
/**
* The provisioning state
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* A secondary expressRoute circuit from a separate AZ. Only present in a stretched private cloud
*
*/
@Export(name="secondaryCircuit", refs={CircuitResponse.class}, tree="[0]")
private Output* @Nullable */ CircuitResponse> secondaryCircuit;
/**
* @return A secondary expressRoute circuit from a separate AZ. Only present in a stretched private cloud
*
*/
public Output> secondaryCircuit() {
return Codegen.optional(this.secondaryCircuit);
}
/**
* The private cloud SKU
*
*/
@Export(name="sku", refs={SkuResponse.class}, tree="[0]")
private Output sku;
/**
* @return The private cloud SKU
*
*/
public Output sku() {
return this.sku;
}
/**
* Resource tags
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type.
*
*/
public Output type() {
return this.type;
}
/**
* Thumbprint of the vCenter Server SSL certificate
*
*/
@Export(name="vcenterCertificateThumbprint", refs={String.class}, tree="[0]")
private Output vcenterCertificateThumbprint;
/**
* @return Thumbprint of the vCenter Server SSL certificate
*
*/
public Output vcenterCertificateThumbprint() {
return this.vcenterCertificateThumbprint;
}
/**
* Optionally, set the vCenter admin password when the private cloud is created
*
*/
@Export(name="vcenterPassword", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> vcenterPassword;
/**
* @return Optionally, set the vCenter admin password when the private cloud is created
*
*/
public Output> vcenterPassword() {
return Codegen.optional(this.vcenterPassword);
}
/**
* Used for live migration of virtual machines
*
*/
@Export(name="vmotionNetwork", refs={String.class}, tree="[0]")
private Output vmotionNetwork;
/**
* @return Used for live migration of virtual machines
*
*/
public Output vmotionNetwork() {
return this.vmotionNetwork;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public PrivateCloud(java.lang.String name) {
this(name, PrivateCloudArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public PrivateCloud(java.lang.String name, PrivateCloudArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public PrivateCloud(java.lang.String name, PrivateCloudArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:avs:PrivateCloud", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private PrivateCloud(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:avs:PrivateCloud", name, null, makeResourceOptions(options, id), false);
}
private static PrivateCloudArgs makeArgs(PrivateCloudArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? PrivateCloudArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:avs/v20200320:PrivateCloud").build()),
Output.of(Alias.builder().type("azure-native:avs/v20200717preview:PrivateCloud").build()),
Output.of(Alias.builder().type("azure-native:avs/v20210101preview:PrivateCloud").build()),
Output.of(Alias.builder().type("azure-native:avs/v20210601:PrivateCloud").build()),
Output.of(Alias.builder().type("azure-native:avs/v20211201:PrivateCloud").build()),
Output.of(Alias.builder().type("azure-native:avs/v20220501:PrivateCloud").build()),
Output.of(Alias.builder().type("azure-native:avs/v20230301:PrivateCloud").build()),
Output.of(Alias.builder().type("azure-native:avs/v20230901:PrivateCloud").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static PrivateCloud get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new PrivateCloud(name, id, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy