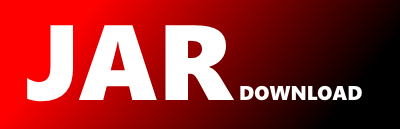
com.pulumi.azurenative.awsconnector.inputs.LoggingConfigArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.inputs;
import com.pulumi.azurenative.awsconnector.enums.LoggingConfigApplicationLogLevel;
import com.pulumi.azurenative.awsconnector.enums.LoggingConfigLogFormat;
import com.pulumi.azurenative.awsconnector.enums.LoggingConfigProtocol;
import com.pulumi.azurenative.awsconnector.enums.LoggingConfigSystemLogLevel;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Definition of LoggingConfig
*
*/
public final class LoggingConfigArgs extends com.pulumi.resources.ResourceArgs {
public static final LoggingConfigArgs Empty = new LoggingConfigArgs();
/**
* Set this property to filter the application logs for your function that Lambda sends to CloudWatch. Lambda only sends application logs at the selected level of detail and lower, where ``TRACE`` is the highest level and ``FATAL`` is the lowest.
*
*/
@Import(name="applicationLogLevel")
private @Nullable Output> applicationLogLevel;
/**
* @return Set this property to filter the application logs for your function that Lambda sends to CloudWatch. Lambda only sends application logs at the selected level of detail and lower, where ``TRACE`` is the highest level and ``FATAL`` is the lowest.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy