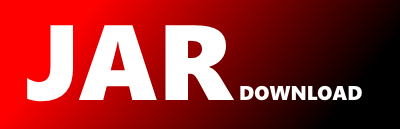
com.pulumi.azurenative.awsconnector.inputs.SeveritySummaryArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Definition of SeveritySummary
*
*/
public final class SeveritySummaryArgs extends com.pulumi.resources.ResourceArgs {
public static final SeveritySummaryArgs Empty = new SeveritySummaryArgs();
/**
* <p>The total number of resources or compliance items that have a severity level of <code>Critical</code>. Critical severity is determined by the organization that published the compliance items.</p>
*
*/
@Import(name="criticalCount")
private @Nullable Output criticalCount;
/**
* @return <p>The total number of resources or compliance items that have a severity level of <code>Critical</code>. Critical severity is determined by the organization that published the compliance items.</p>
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy