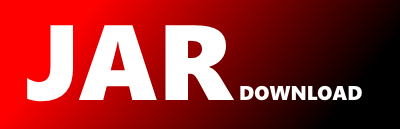
com.pulumi.azurenative.awsconnector.outputs.ElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.azurenative.awsconnector.outputs.AwsElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse {
/**
* @return Amazon Resource Name (ARN)
*
*/
private @Nullable String arn;
/**
* @return AWS Account ID
*
*/
private @Nullable String awsAccountId;
/**
* @return AWS Properties
*
*/
private @Nullable AwsElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse awsProperties;
/**
* @return AWS Region
*
*/
private @Nullable String awsRegion;
/**
* @return AWS Source Schema
*
*/
private @Nullable String awsSourceSchema;
/**
* @return AWS Tags
*
*/
private @Nullable Map awsTags;
/**
* @return The status of the last operation.
*
*/
private String provisioningState;
/**
* @return Public Cloud Connectors Resource ID
*
*/
private @Nullable String publicCloudConnectorsResourceId;
/**
* @return Public Cloud Resource Name
*
*/
private @Nullable String publicCloudResourceName;
private ElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse() {}
/**
* @return Amazon Resource Name (ARN)
*
*/
public Optional arn() {
return Optional.ofNullable(this.arn);
}
/**
* @return AWS Account ID
*
*/
public Optional awsAccountId() {
return Optional.ofNullable(this.awsAccountId);
}
/**
* @return AWS Properties
*
*/
public Optional awsProperties() {
return Optional.ofNullable(this.awsProperties);
}
/**
* @return AWS Region
*
*/
public Optional awsRegion() {
return Optional.ofNullable(this.awsRegion);
}
/**
* @return AWS Source Schema
*
*/
public Optional awsSourceSchema() {
return Optional.ofNullable(this.awsSourceSchema);
}
/**
* @return AWS Tags
*
*/
public Map awsTags() {
return this.awsTags == null ? Map.of() : this.awsTags;
}
/**
* @return The status of the last operation.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Public Cloud Connectors Resource ID
*
*/
public Optional publicCloudConnectorsResourceId() {
return Optional.ofNullable(this.publicCloudConnectorsResourceId);
}
/**
* @return Public Cloud Resource Name
*
*/
public Optional publicCloudResourceName() {
return Optional.ofNullable(this.publicCloudResourceName);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String arn;
private @Nullable String awsAccountId;
private @Nullable AwsElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse awsProperties;
private @Nullable String awsRegion;
private @Nullable String awsSourceSchema;
private @Nullable Map awsTags;
private String provisioningState;
private @Nullable String publicCloudConnectorsResourceId;
private @Nullable String publicCloudResourceName;
public Builder() {}
public Builder(ElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.awsAccountId = defaults.awsAccountId;
this.awsProperties = defaults.awsProperties;
this.awsRegion = defaults.awsRegion;
this.awsSourceSchema = defaults.awsSourceSchema;
this.awsTags = defaults.awsTags;
this.provisioningState = defaults.provisioningState;
this.publicCloudConnectorsResourceId = defaults.publicCloudConnectorsResourceId;
this.publicCloudResourceName = defaults.publicCloudResourceName;
}
@CustomType.Setter
public Builder arn(@Nullable String arn) {
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder awsAccountId(@Nullable String awsAccountId) {
this.awsAccountId = awsAccountId;
return this;
}
@CustomType.Setter
public Builder awsProperties(@Nullable AwsElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse awsProperties) {
this.awsProperties = awsProperties;
return this;
}
@CustomType.Setter
public Builder awsRegion(@Nullable String awsRegion) {
this.awsRegion = awsRegion;
return this;
}
@CustomType.Setter
public Builder awsSourceSchema(@Nullable String awsSourceSchema) {
this.awsSourceSchema = awsSourceSchema;
return this;
}
@CustomType.Setter
public Builder awsTags(@Nullable Map awsTags) {
this.awsTags = awsTags;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("ElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicCloudConnectorsResourceId(@Nullable String publicCloudConnectorsResourceId) {
this.publicCloudConnectorsResourceId = publicCloudConnectorsResourceId;
return this;
}
@CustomType.Setter
public Builder publicCloudResourceName(@Nullable String publicCloudResourceName) {
this.publicCloudResourceName = publicCloudResourceName;
return this;
}
public ElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse build() {
final var _resultValue = new ElasticLoadBalancingv2TargetHealthDescriptionPropertiesResponse();
_resultValue.arn = arn;
_resultValue.awsAccountId = awsAccountId;
_resultValue.awsProperties = awsProperties;
_resultValue.awsRegion = awsRegion;
_resultValue.awsSourceSchema = awsSourceSchema;
_resultValue.awsTags = awsTags;
_resultValue.provisioningState = provisioningState;
_resultValue.publicCloudConnectorsResourceId = publicCloudConnectorsResourceId;
_resultValue.publicCloudResourceName = publicCloudResourceName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy