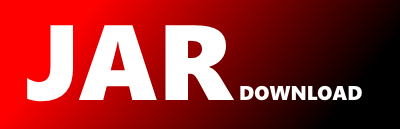
com.pulumi.azurenative.awsconnector.outputs.IpPermissionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.azurenative.awsconnector.outputs.IpRangeResponse;
import com.pulumi.azurenative.awsconnector.outputs.Ipv6RangeResponse;
import com.pulumi.azurenative.awsconnector.outputs.PrefixListIdResponse;
import com.pulumi.azurenative.awsconnector.outputs.UserIdGroupPairResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class IpPermissionResponse {
/**
* @return <p>If the protocol is TCP or UDP, this is the start of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP type or -1 (all ICMP types).</p>
*
*/
private @Nullable Integer fromPort;
/**
* @return <p>The IP protocol name (<code>tcp</code>, <code>udp</code>, <code>icmp</code>, <code>icmpv6</code>) or number (see <a href='http://www.iana.org/assignments/protocol-numbers/protocol-numbers.xhtml'>Protocol Numbers</a>).</p> <p>Use <code>-1</code> to specify all protocols. When authorizing security group rules, specifying <code>-1</code> or a protocol number other than <code>tcp</code>, <code>udp</code>, <code>icmp</code>, or <code>icmpv6</code> allows traffic on all ports, regardless of any port range you specify. For <code>tcp</code>, <code>udp</code>, and <code>icmp</code>, you must specify a port range. For <code>icmpv6</code>, the port range is optional; if you omit the port range, traffic for all types and codes is allowed.</p>
*
*/
private @Nullable String ipProtocol;
/**
* @return <p>The IPv4 address ranges.</p>
*
*/
private @Nullable List ipRanges;
/**
* @return <p>The IPv6 address ranges.</p>
*
*/
private @Nullable List ipv6Ranges;
/**
* @return <p>The prefix list IDs.</p>
*
*/
private @Nullable List prefixListIds;
/**
* @return <p>If the protocol is TCP or UDP, this is the end of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP code or -1 (all ICMP codes). If the start port is -1 (all ICMP types), then the end port must be -1 (all ICMP codes).</p>
*
*/
private @Nullable Integer toPort;
/**
* @return <p>The security group and Amazon Web Services account ID pairs.</p>
*
*/
private @Nullable List userIdGroupPairs;
private IpPermissionResponse() {}
/**
* @return <p>If the protocol is TCP or UDP, this is the start of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP type or -1 (all ICMP types).</p>
*
*/
public Optional fromPort() {
return Optional.ofNullable(this.fromPort);
}
/**
* @return <p>The IP protocol name (<code>tcp</code>, <code>udp</code>, <code>icmp</code>, <code>icmpv6</code>) or number (see <a href='http://www.iana.org/assignments/protocol-numbers/protocol-numbers.xhtml'>Protocol Numbers</a>).</p> <p>Use <code>-1</code> to specify all protocols. When authorizing security group rules, specifying <code>-1</code> or a protocol number other than <code>tcp</code>, <code>udp</code>, <code>icmp</code>, or <code>icmpv6</code> allows traffic on all ports, regardless of any port range you specify. For <code>tcp</code>, <code>udp</code>, and <code>icmp</code>, you must specify a port range. For <code>icmpv6</code>, the port range is optional; if you omit the port range, traffic for all types and codes is allowed.</p>
*
*/
public Optional ipProtocol() {
return Optional.ofNullable(this.ipProtocol);
}
/**
* @return <p>The IPv4 address ranges.</p>
*
*/
public List ipRanges() {
return this.ipRanges == null ? List.of() : this.ipRanges;
}
/**
* @return <p>The IPv6 address ranges.</p>
*
*/
public List ipv6Ranges() {
return this.ipv6Ranges == null ? List.of() : this.ipv6Ranges;
}
/**
* @return <p>The prefix list IDs.</p>
*
*/
public List prefixListIds() {
return this.prefixListIds == null ? List.of() : this.prefixListIds;
}
/**
* @return <p>If the protocol is TCP or UDP, this is the end of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP code or -1 (all ICMP codes). If the start port is -1 (all ICMP types), then the end port must be -1 (all ICMP codes).</p>
*
*/
public Optional toPort() {
return Optional.ofNullable(this.toPort);
}
/**
* @return <p>The security group and Amazon Web Services account ID pairs.</p>
*
*/
public List userIdGroupPairs() {
return this.userIdGroupPairs == null ? List.of() : this.userIdGroupPairs;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IpPermissionResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer fromPort;
private @Nullable String ipProtocol;
private @Nullable List ipRanges;
private @Nullable List ipv6Ranges;
private @Nullable List prefixListIds;
private @Nullable Integer toPort;
private @Nullable List userIdGroupPairs;
public Builder() {}
public Builder(IpPermissionResponse defaults) {
Objects.requireNonNull(defaults);
this.fromPort = defaults.fromPort;
this.ipProtocol = defaults.ipProtocol;
this.ipRanges = defaults.ipRanges;
this.ipv6Ranges = defaults.ipv6Ranges;
this.prefixListIds = defaults.prefixListIds;
this.toPort = defaults.toPort;
this.userIdGroupPairs = defaults.userIdGroupPairs;
}
@CustomType.Setter
public Builder fromPort(@Nullable Integer fromPort) {
this.fromPort = fromPort;
return this;
}
@CustomType.Setter
public Builder ipProtocol(@Nullable String ipProtocol) {
this.ipProtocol = ipProtocol;
return this;
}
@CustomType.Setter
public Builder ipRanges(@Nullable List ipRanges) {
this.ipRanges = ipRanges;
return this;
}
public Builder ipRanges(IpRangeResponse... ipRanges) {
return ipRanges(List.of(ipRanges));
}
@CustomType.Setter
public Builder ipv6Ranges(@Nullable List ipv6Ranges) {
this.ipv6Ranges = ipv6Ranges;
return this;
}
public Builder ipv6Ranges(Ipv6RangeResponse... ipv6Ranges) {
return ipv6Ranges(List.of(ipv6Ranges));
}
@CustomType.Setter
public Builder prefixListIds(@Nullable List prefixListIds) {
this.prefixListIds = prefixListIds;
return this;
}
public Builder prefixListIds(PrefixListIdResponse... prefixListIds) {
return prefixListIds(List.of(prefixListIds));
}
@CustomType.Setter
public Builder toPort(@Nullable Integer toPort) {
this.toPort = toPort;
return this;
}
@CustomType.Setter
public Builder userIdGroupPairs(@Nullable List userIdGroupPairs) {
this.userIdGroupPairs = userIdGroupPairs;
return this;
}
public Builder userIdGroupPairs(UserIdGroupPairResponse... userIdGroupPairs) {
return userIdGroupPairs(List.of(userIdGroupPairs));
}
public IpPermissionResponse build() {
final var _resultValue = new IpPermissionResponse();
_resultValue.fromPort = fromPort;
_resultValue.ipProtocol = ipProtocol;
_resultValue.ipRanges = ipRanges;
_resultValue.ipv6Ranges = ipv6Ranges;
_resultValue.prefixListIds = prefixListIds;
_resultValue.toPort = toPort;
_resultValue.userIdGroupPairs = userIdGroupPairs;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy