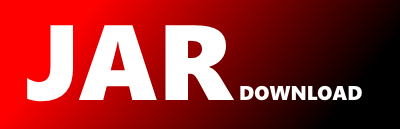
com.pulumi.azurenative.awsconnector.outputs.VpcConfigResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VpcConfigResponse {
/**
* @return <p>The cluster security group that was created by Amazon EKS for the cluster. Managed node groups use this security group for control-plane-to-data-plane communication.</p>
*
*/
private @Nullable String clusterSecurityGroupId;
/**
* @return <p>This parameter indicates whether the Amazon EKS private API server endpoint is enabled. If the Amazon EKS private API server endpoint is enabled, Kubernetes API requests that originate from within your cluster's VPC use the private VPC endpoint instead of traversing the internet. If this value is disabled and you have nodes or Fargate pods in the cluster, then ensure that <code>publicAccessCidrs</code> includes the necessary CIDR blocks for communication with the nodes or Fargate pods. For more information, see <a href='https://docs.aws.amazon.com/eks/latest/userguide/cluster-endpoint.html'>Amazon EKS cluster endpoint access control</a> in the <i> <i>Amazon EKS User Guide</i> </i>.</p>
*
*/
private @Nullable Boolean endpointPrivateAccess;
/**
* @return <p>Whether the public API server endpoint is enabled.</p>
*
*/
private @Nullable Boolean endpointPublicAccess;
/**
* @return Allows outbound IPv6 traffic on VPC functions that are connected to dual-stack subnets.
*
*/
private @Nullable Boolean ipv6AllowedForDualStack;
/**
* @return <p>The CIDR blocks that are allowed access to your cluster's public Kubernetes API server endpoint.</p>
*
*/
private @Nullable List publicAccessCidrs;
/**
* @return A list of VPC security group IDs.
*
*/
private @Nullable List securityGroupIds;
/**
* @return A list of VPC subnet IDs.
*
*/
private @Nullable List subnetIds;
/**
* @return <p>A list of one or more subnet IDs in your Amazon VPC.</p>
*
*/
private @Nullable List subnets;
/**
* @return <p>The VPC associated with your cluster.</p>
*
*/
private @Nullable String vpcId;
private VpcConfigResponse() {}
/**
* @return <p>The cluster security group that was created by Amazon EKS for the cluster. Managed node groups use this security group for control-plane-to-data-plane communication.</p>
*
*/
public Optional clusterSecurityGroupId() {
return Optional.ofNullable(this.clusterSecurityGroupId);
}
/**
* @return <p>This parameter indicates whether the Amazon EKS private API server endpoint is enabled. If the Amazon EKS private API server endpoint is enabled, Kubernetes API requests that originate from within your cluster's VPC use the private VPC endpoint instead of traversing the internet. If this value is disabled and you have nodes or Fargate pods in the cluster, then ensure that <code>publicAccessCidrs</code> includes the necessary CIDR blocks for communication with the nodes or Fargate pods. For more information, see <a href='https://docs.aws.amazon.com/eks/latest/userguide/cluster-endpoint.html'>Amazon EKS cluster endpoint access control</a> in the <i> <i>Amazon EKS User Guide</i> </i>.</p>
*
*/
public Optional endpointPrivateAccess() {
return Optional.ofNullable(this.endpointPrivateAccess);
}
/**
* @return <p>Whether the public API server endpoint is enabled.</p>
*
*/
public Optional endpointPublicAccess() {
return Optional.ofNullable(this.endpointPublicAccess);
}
/**
* @return Allows outbound IPv6 traffic on VPC functions that are connected to dual-stack subnets.
*
*/
public Optional ipv6AllowedForDualStack() {
return Optional.ofNullable(this.ipv6AllowedForDualStack);
}
/**
* @return <p>The CIDR blocks that are allowed access to your cluster's public Kubernetes API server endpoint.</p>
*
*/
public List publicAccessCidrs() {
return this.publicAccessCidrs == null ? List.of() : this.publicAccessCidrs;
}
/**
* @return A list of VPC security group IDs.
*
*/
public List securityGroupIds() {
return this.securityGroupIds == null ? List.of() : this.securityGroupIds;
}
/**
* @return A list of VPC subnet IDs.
*
*/
public List subnetIds() {
return this.subnetIds == null ? List.of() : this.subnetIds;
}
/**
* @return <p>A list of one or more subnet IDs in your Amazon VPC.</p>
*
*/
public List subnets() {
return this.subnets == null ? List.of() : this.subnets;
}
/**
* @return <p>The VPC associated with your cluster.</p>
*
*/
public Optional vpcId() {
return Optional.ofNullable(this.vpcId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VpcConfigResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String clusterSecurityGroupId;
private @Nullable Boolean endpointPrivateAccess;
private @Nullable Boolean endpointPublicAccess;
private @Nullable Boolean ipv6AllowedForDualStack;
private @Nullable List publicAccessCidrs;
private @Nullable List securityGroupIds;
private @Nullable List subnetIds;
private @Nullable List subnets;
private @Nullable String vpcId;
public Builder() {}
public Builder(VpcConfigResponse defaults) {
Objects.requireNonNull(defaults);
this.clusterSecurityGroupId = defaults.clusterSecurityGroupId;
this.endpointPrivateAccess = defaults.endpointPrivateAccess;
this.endpointPublicAccess = defaults.endpointPublicAccess;
this.ipv6AllowedForDualStack = defaults.ipv6AllowedForDualStack;
this.publicAccessCidrs = defaults.publicAccessCidrs;
this.securityGroupIds = defaults.securityGroupIds;
this.subnetIds = defaults.subnetIds;
this.subnets = defaults.subnets;
this.vpcId = defaults.vpcId;
}
@CustomType.Setter
public Builder clusterSecurityGroupId(@Nullable String clusterSecurityGroupId) {
this.clusterSecurityGroupId = clusterSecurityGroupId;
return this;
}
@CustomType.Setter
public Builder endpointPrivateAccess(@Nullable Boolean endpointPrivateAccess) {
this.endpointPrivateAccess = endpointPrivateAccess;
return this;
}
@CustomType.Setter
public Builder endpointPublicAccess(@Nullable Boolean endpointPublicAccess) {
this.endpointPublicAccess = endpointPublicAccess;
return this;
}
@CustomType.Setter
public Builder ipv6AllowedForDualStack(@Nullable Boolean ipv6AllowedForDualStack) {
this.ipv6AllowedForDualStack = ipv6AllowedForDualStack;
return this;
}
@CustomType.Setter
public Builder publicAccessCidrs(@Nullable List publicAccessCidrs) {
this.publicAccessCidrs = publicAccessCidrs;
return this;
}
public Builder publicAccessCidrs(String... publicAccessCidrs) {
return publicAccessCidrs(List.of(publicAccessCidrs));
}
@CustomType.Setter
public Builder securityGroupIds(@Nullable List securityGroupIds) {
this.securityGroupIds = securityGroupIds;
return this;
}
public Builder securityGroupIds(String... securityGroupIds) {
return securityGroupIds(List.of(securityGroupIds));
}
@CustomType.Setter
public Builder subnetIds(@Nullable List subnetIds) {
this.subnetIds = subnetIds;
return this;
}
public Builder subnetIds(String... subnetIds) {
return subnetIds(List.of(subnetIds));
}
@CustomType.Setter
public Builder subnets(@Nullable List subnets) {
this.subnets = subnets;
return this;
}
public Builder subnets(String... subnets) {
return subnets(List.of(subnets));
}
@CustomType.Setter
public Builder vpcId(@Nullable String vpcId) {
this.vpcId = vpcId;
return this;
}
public VpcConfigResponse build() {
final var _resultValue = new VpcConfigResponse();
_resultValue.clusterSecurityGroupId = clusterSecurityGroupId;
_resultValue.endpointPrivateAccess = endpointPrivateAccess;
_resultValue.endpointPublicAccess = endpointPublicAccess;
_resultValue.ipv6AllowedForDualStack = ipv6AllowedForDualStack;
_resultValue.publicAccessCidrs = publicAccessCidrs;
_resultValue.securityGroupIds = securityGroupIds;
_resultValue.subnetIds = subnetIds;
_resultValue.subnets = subnets;
_resultValue.vpcId = vpcId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy