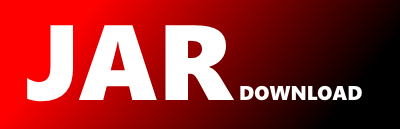
com.pulumi.azurenative.azuredatatransfer.outputs.PipelinePropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azuredatatransfer.outputs;
import com.pulumi.azurenative.azuredatatransfer.outputs.PipelineConnectionResponse;
import com.pulumi.azurenative.azuredatatransfer.outputs.SubscriberResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class PipelinePropertiesResponse {
/**
* @return Connections associated with pipeline
*
*/
private List connections;
/**
* @return Display name of this pipeline
*
*/
private @Nullable String displayName;
/**
* @return The flow types allowed for this pipeline
*
*/
private @Nullable List flowTypes;
/**
* @return The policies for this pipeline
*
*/
private @Nullable List policies;
/**
* @return Provisioning state of the pipeline
*
*/
private String provisioningState;
/**
* @return Remote cloud of the data to be transferred or received
*
*/
private String remoteCloud;
/**
* @return Subscribers of this resource
*
*/
private @Nullable List subscribers;
private PipelinePropertiesResponse() {}
/**
* @return Connections associated with pipeline
*
*/
public List connections() {
return this.connections;
}
/**
* @return Display name of this pipeline
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return The flow types allowed for this pipeline
*
*/
public List flowTypes() {
return this.flowTypes == null ? List.of() : this.flowTypes;
}
/**
* @return The policies for this pipeline
*
*/
public List policies() {
return this.policies == null ? List.of() : this.policies;
}
/**
* @return Provisioning state of the pipeline
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Remote cloud of the data to be transferred or received
*
*/
public String remoteCloud() {
return this.remoteCloud;
}
/**
* @return Subscribers of this resource
*
*/
public List subscribers() {
return this.subscribers == null ? List.of() : this.subscribers;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PipelinePropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List connections;
private @Nullable String displayName;
private @Nullable List flowTypes;
private @Nullable List policies;
private String provisioningState;
private String remoteCloud;
private @Nullable List subscribers;
public Builder() {}
public Builder(PipelinePropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.connections = defaults.connections;
this.displayName = defaults.displayName;
this.flowTypes = defaults.flowTypes;
this.policies = defaults.policies;
this.provisioningState = defaults.provisioningState;
this.remoteCloud = defaults.remoteCloud;
this.subscribers = defaults.subscribers;
}
@CustomType.Setter
public Builder connections(List connections) {
if (connections == null) {
throw new MissingRequiredPropertyException("PipelinePropertiesResponse", "connections");
}
this.connections = connections;
return this;
}
public Builder connections(PipelineConnectionResponse... connections) {
return connections(List.of(connections));
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder flowTypes(@Nullable List flowTypes) {
this.flowTypes = flowTypes;
return this;
}
public Builder flowTypes(String... flowTypes) {
return flowTypes(List.of(flowTypes));
}
@CustomType.Setter
public Builder policies(@Nullable List policies) {
this.policies = policies;
return this;
}
public Builder policies(String... policies) {
return policies(List.of(policies));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("PipelinePropertiesResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder remoteCloud(String remoteCloud) {
if (remoteCloud == null) {
throw new MissingRequiredPropertyException("PipelinePropertiesResponse", "remoteCloud");
}
this.remoteCloud = remoteCloud;
return this;
}
@CustomType.Setter
public Builder subscribers(@Nullable List subscribers) {
this.subscribers = subscribers;
return this;
}
public Builder subscribers(SubscriberResponse... subscribers) {
return subscribers(List.of(subscribers));
}
public PipelinePropertiesResponse build() {
final var _resultValue = new PipelinePropertiesResponse();
_resultValue.connections = connections;
_resultValue.displayName = displayName;
_resultValue.flowTypes = flowTypes;
_resultValue.policies = policies;
_resultValue.provisioningState = provisioningState;
_resultValue.remoteCloud = remoteCloud;
_resultValue.subscribers = subscribers;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy