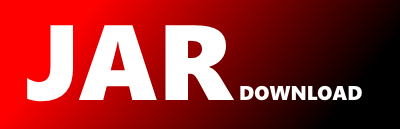
com.pulumi.azurenative.azureplaywrightservice.outputs.GetAccountResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azureplaywrightservice.outputs;
import com.pulumi.azurenative.azureplaywrightservice.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAccountResult {
/**
* @return The Playwright testing dashboard URI for the account resource.
*
*/
private String dashboardUri;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The status of the last operation.
*
*/
private String provisioningState;
/**
* @return This property sets the connection region for Playwright client workers to cloud-hosted browsers. If enabled, workers connect to browsers in the closest Azure region, ensuring lower latency. If disabled, workers connect to browsers in the Azure region in which the workspace was initially created.
*
*/
private @Nullable String regionalAffinity;
/**
* @return When enabled, this feature allows the workspace to upload and display test results, including artifacts like traces and screenshots, in the Playwright portal. This enables faster and more efficient troubleshooting.
*
*/
private @Nullable String reporting;
/**
* @return When enabled, Playwright client workers can connect to cloud-hosted browsers. This can increase the number of parallel workers for a test run, significantly minimizing test completion durations.
*
*/
private @Nullable String scalableExecution;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetAccountResult() {}
/**
* @return The Playwright testing dashboard URI for the account resource.
*
*/
public String dashboardUri() {
return this.dashboardUri;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The status of the last operation.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return This property sets the connection region for Playwright client workers to cloud-hosted browsers. If enabled, workers connect to browsers in the closest Azure region, ensuring lower latency. If disabled, workers connect to browsers in the Azure region in which the workspace was initially created.
*
*/
public Optional regionalAffinity() {
return Optional.ofNullable(this.regionalAffinity);
}
/**
* @return When enabled, this feature allows the workspace to upload and display test results, including artifacts like traces and screenshots, in the Playwright portal. This enables faster and more efficient troubleshooting.
*
*/
public Optional reporting() {
return Optional.ofNullable(this.reporting);
}
/**
* @return When enabled, Playwright client workers can connect to cloud-hosted browsers. This can increase the number of parallel workers for a test run, significantly minimizing test completion durations.
*
*/
public Optional scalableExecution() {
return Optional.ofNullable(this.scalableExecution);
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAccountResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String dashboardUri;
private String id;
private String location;
private String name;
private String provisioningState;
private @Nullable String regionalAffinity;
private @Nullable String reporting;
private @Nullable String scalableExecution;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetAccountResult defaults) {
Objects.requireNonNull(defaults);
this.dashboardUri = defaults.dashboardUri;
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.regionalAffinity = defaults.regionalAffinity;
this.reporting = defaults.reporting;
this.scalableExecution = defaults.scalableExecution;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder dashboardUri(String dashboardUri) {
if (dashboardUri == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "dashboardUri");
}
this.dashboardUri = dashboardUri;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder regionalAffinity(@Nullable String regionalAffinity) {
this.regionalAffinity = regionalAffinity;
return this;
}
@CustomType.Setter
public Builder reporting(@Nullable String reporting) {
this.reporting = reporting;
return this;
}
@CustomType.Setter
public Builder scalableExecution(@Nullable String scalableExecution) {
this.scalableExecution = scalableExecution;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "type");
}
this.type = type;
return this;
}
public GetAccountResult build() {
final var _resultValue = new GetAccountResult();
_resultValue.dashboardUri = dashboardUri;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.regionalAffinity = regionalAffinity;
_resultValue.reporting = reporting;
_resultValue.scalableExecution = scalableExecution;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy