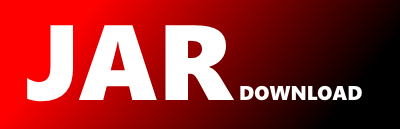
com.pulumi.azurenative.botservice.outputs.ChannelSettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.botservice.outputs;
import com.pulumi.azurenative.botservice.outputs.SiteResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ChannelSettingsResponse {
/**
* @return The bot icon url
*
*/
private @Nullable String botIconUrl;
/**
* @return The bot id
*
*/
private @Nullable String botId;
/**
* @return The channel display name
*
*/
private @Nullable String channelDisplayName;
/**
* @return The channel id
*
*/
private @Nullable String channelId;
/**
* @return Opt-out of local authentication and ensure only MSI and AAD can be used exclusively for authentication.
*
*/
private @Nullable Boolean disableLocalAuth;
/**
* @return The extensionKey1
*
*/
private @Nullable String extensionKey1;
/**
* @return The extensionKey2
*
*/
private @Nullable String extensionKey2;
/**
* @return Whether this channel is enabled for the bot
*
*/
private @Nullable Boolean isEnabled;
/**
* @return Whether customer needs to agree to new terms.
*
*/
private @Nullable Boolean requireTermsAgreement;
/**
* @return The list of sites
*
*/
private @Nullable List sites;
private ChannelSettingsResponse() {}
/**
* @return The bot icon url
*
*/
public Optional botIconUrl() {
return Optional.ofNullable(this.botIconUrl);
}
/**
* @return The bot id
*
*/
public Optional botId() {
return Optional.ofNullable(this.botId);
}
/**
* @return The channel display name
*
*/
public Optional channelDisplayName() {
return Optional.ofNullable(this.channelDisplayName);
}
/**
* @return The channel id
*
*/
public Optional channelId() {
return Optional.ofNullable(this.channelId);
}
/**
* @return Opt-out of local authentication and ensure only MSI and AAD can be used exclusively for authentication.
*
*/
public Optional disableLocalAuth() {
return Optional.ofNullable(this.disableLocalAuth);
}
/**
* @return The extensionKey1
*
*/
public Optional extensionKey1() {
return Optional.ofNullable(this.extensionKey1);
}
/**
* @return The extensionKey2
*
*/
public Optional extensionKey2() {
return Optional.ofNullable(this.extensionKey2);
}
/**
* @return Whether this channel is enabled for the bot
*
*/
public Optional isEnabled() {
return Optional.ofNullable(this.isEnabled);
}
/**
* @return Whether customer needs to agree to new terms.
*
*/
public Optional requireTermsAgreement() {
return Optional.ofNullable(this.requireTermsAgreement);
}
/**
* @return The list of sites
*
*/
public List sites() {
return this.sites == null ? List.of() : this.sites;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ChannelSettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String botIconUrl;
private @Nullable String botId;
private @Nullable String channelDisplayName;
private @Nullable String channelId;
private @Nullable Boolean disableLocalAuth;
private @Nullable String extensionKey1;
private @Nullable String extensionKey2;
private @Nullable Boolean isEnabled;
private @Nullable Boolean requireTermsAgreement;
private @Nullable List sites;
public Builder() {}
public Builder(ChannelSettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.botIconUrl = defaults.botIconUrl;
this.botId = defaults.botId;
this.channelDisplayName = defaults.channelDisplayName;
this.channelId = defaults.channelId;
this.disableLocalAuth = defaults.disableLocalAuth;
this.extensionKey1 = defaults.extensionKey1;
this.extensionKey2 = defaults.extensionKey2;
this.isEnabled = defaults.isEnabled;
this.requireTermsAgreement = defaults.requireTermsAgreement;
this.sites = defaults.sites;
}
@CustomType.Setter
public Builder botIconUrl(@Nullable String botIconUrl) {
this.botIconUrl = botIconUrl;
return this;
}
@CustomType.Setter
public Builder botId(@Nullable String botId) {
this.botId = botId;
return this;
}
@CustomType.Setter
public Builder channelDisplayName(@Nullable String channelDisplayName) {
this.channelDisplayName = channelDisplayName;
return this;
}
@CustomType.Setter
public Builder channelId(@Nullable String channelId) {
this.channelId = channelId;
return this;
}
@CustomType.Setter
public Builder disableLocalAuth(@Nullable Boolean disableLocalAuth) {
this.disableLocalAuth = disableLocalAuth;
return this;
}
@CustomType.Setter
public Builder extensionKey1(@Nullable String extensionKey1) {
this.extensionKey1 = extensionKey1;
return this;
}
@CustomType.Setter
public Builder extensionKey2(@Nullable String extensionKey2) {
this.extensionKey2 = extensionKey2;
return this;
}
@CustomType.Setter
public Builder isEnabled(@Nullable Boolean isEnabled) {
this.isEnabled = isEnabled;
return this;
}
@CustomType.Setter
public Builder requireTermsAgreement(@Nullable Boolean requireTermsAgreement) {
this.requireTermsAgreement = requireTermsAgreement;
return this;
}
@CustomType.Setter
public Builder sites(@Nullable List sites) {
this.sites = sites;
return this;
}
public Builder sites(SiteResponse... sites) {
return sites(List.of(sites));
}
public ChannelSettingsResponse build() {
final var _resultValue = new ChannelSettingsResponse();
_resultValue.botIconUrl = botIconUrl;
_resultValue.botId = botId;
_resultValue.channelDisplayName = channelDisplayName;
_resultValue.channelId = channelId;
_resultValue.disableLocalAuth = disableLocalAuth;
_resultValue.extensionKey1 = extensionKey1;
_resultValue.extensionKey2 = extensionKey2;
_resultValue.isEnabled = isEnabled;
_resultValue.requireTermsAgreement = requireTermsAgreement;
_resultValue.sites = sites;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy