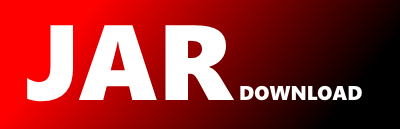
com.pulumi.azurenative.cloudngfw.FirewallArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cloudngfw;
import com.pulumi.azurenative.cloudngfw.enums.BooleanEnum;
import com.pulumi.azurenative.cloudngfw.inputs.AzureResourceManagerManagedIdentityPropertiesArgs;
import com.pulumi.azurenative.cloudngfw.inputs.DNSSettingsArgs;
import com.pulumi.azurenative.cloudngfw.inputs.FrontendSettingArgs;
import com.pulumi.azurenative.cloudngfw.inputs.MarketplaceDetailsArgs;
import com.pulumi.azurenative.cloudngfw.inputs.NetworkProfileArgs;
import com.pulumi.azurenative.cloudngfw.inputs.PanoramaConfigArgs;
import com.pulumi.azurenative.cloudngfw.inputs.PlanDataArgs;
import com.pulumi.azurenative.cloudngfw.inputs.RulestackDetailsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class FirewallArgs extends com.pulumi.resources.ResourceArgs {
public static final FirewallArgs Empty = new FirewallArgs();
/**
* Associated Rulestack
*
*/
@Import(name="associatedRulestack")
private @Nullable Output associatedRulestack;
/**
* @return Associated Rulestack
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy