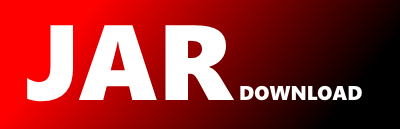
com.pulumi.azurenative.compute.CapacityReservationGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.compute.CapacityReservationGroupArgs;
import com.pulumi.azurenative.compute.outputs.CapacityReservationGroupInstanceViewResponse;
import com.pulumi.azurenative.compute.outputs.SubResourceReadOnlyResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Specifies information about the capacity reservation group that the capacity reservations should be assigned to. Currently, a capacity reservation can only be added to a capacity reservation group at creation time. An existing capacity reservation cannot be added or moved to another capacity reservation group.
* Azure REST API version: 2023-03-01. Prior API version in Azure Native 1.x: 2021-04-01.
*
* Other available API versions: 2023-07-01, 2023-09-01, 2024-03-01, 2024-07-01.
*
* ## Example Usage
* ### Create or update a capacity reservation group.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.CapacityReservationGroup;
* import com.pulumi.azurenative.compute.CapacityReservationGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var capacityReservationGroup = new CapacityReservationGroup("capacityReservationGroup", CapacityReservationGroupArgs.builder()
* .capacityReservationGroupName("myCapacityReservationGroup")
* .location("westus")
* .resourceGroupName("myResourceGroup")
* .tags(Map.of("department", "finance"))
* .zones(
* "1",
* "2")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:compute:CapacityReservationGroup myCapacityReservationGroup /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/capacityReservationGroups/{capacityReservationGroupName}
* ```
*
*/
@ResourceType(type="azure-native:compute:CapacityReservationGroup")
public class CapacityReservationGroup extends com.pulumi.resources.CustomResource {
/**
* A list of all capacity reservation resource ids that belong to capacity reservation group.
*
*/
@Export(name="capacityReservations", refs={List.class,SubResourceReadOnlyResponse.class}, tree="[0,1]")
private Output> capacityReservations;
/**
* @return A list of all capacity reservation resource ids that belong to capacity reservation group.
*
*/
public Output> capacityReservations() {
return this.capacityReservations;
}
/**
* The capacity reservation group instance view which has the list of instance views for all the capacity reservations that belong to the capacity reservation group.
*
*/
@Export(name="instanceView", refs={CapacityReservationGroupInstanceViewResponse.class}, tree="[0]")
private Output instanceView;
/**
* @return The capacity reservation group instance view which has the list of instance views for all the capacity reservations that belong to the capacity reservation group.
*
*/
public Output instanceView() {
return this.instanceView;
}
/**
* Resource location
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource location
*
*/
public Output location() {
return this.location;
}
/**
* Resource name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name
*
*/
public Output name() {
return this.name;
}
/**
* Resource tags
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type
*
*/
public Output type() {
return this.type;
}
/**
* A list of references to all virtual machines associated to the capacity reservation group.
*
*/
@Export(name="virtualMachinesAssociated", refs={List.class,SubResourceReadOnlyResponse.class}, tree="[0,1]")
private Output> virtualMachinesAssociated;
/**
* @return A list of references to all virtual machines associated to the capacity reservation group.
*
*/
public Output> virtualMachinesAssociated() {
return this.virtualMachinesAssociated;
}
/**
* Availability Zones to use for this capacity reservation group. The zones can be assigned only during creation. If not provided, the group supports only regional resources in the region. If provided, enforces each capacity reservation in the group to be in one of the zones.
*
*/
@Export(name="zones", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> zones;
/**
* @return Availability Zones to use for this capacity reservation group. The zones can be assigned only during creation. If not provided, the group supports only regional resources in the region. If provided, enforces each capacity reservation in the group to be in one of the zones.
*
*/
public Output>> zones() {
return Codegen.optional(this.zones);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public CapacityReservationGroup(java.lang.String name) {
this(name, CapacityReservationGroupArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public CapacityReservationGroup(java.lang.String name, CapacityReservationGroupArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public CapacityReservationGroup(java.lang.String name, CapacityReservationGroupArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:compute:CapacityReservationGroup", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private CapacityReservationGroup(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:compute:CapacityReservationGroup", name, null, makeResourceOptions(options, id), false);
}
private static CapacityReservationGroupArgs makeArgs(CapacityReservationGroupArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? CapacityReservationGroupArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:compute/v20210401:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20210701:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20211101:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20220301:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20220801:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20221101:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230301:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230701:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230901:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20240301:CapacityReservationGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20240701:CapacityReservationGroup").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static CapacityReservationGroup get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new CapacityReservationGroup(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy