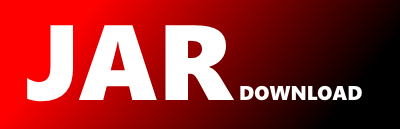
com.pulumi.azurenative.compute.inputs.CreationDataArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.inputs;
import com.pulumi.azurenative.compute.enums.DiskCreateOption;
import com.pulumi.azurenative.compute.inputs.ImageDiskReferenceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Data used when creating a disk.
*
*/
public final class CreationDataArgs extends com.pulumi.resources.ResourceArgs {
public static final CreationDataArgs Empty = new CreationDataArgs();
/**
* This enumerates the possible sources of a disk's creation.
*
*/
@Import(name="createOption", required=true)
private Output> createOption;
/**
* @return This enumerates the possible sources of a disk's creation.
*
*/
public Output> createOption() {
return this.createOption;
}
/**
* Required if creating from a Gallery Image. The id/sharedGalleryImageId/communityGalleryImageId of the ImageDiskReference will be the ARM id of the shared galley image version from which to create a disk.
*
*/
@Import(name="galleryImageReference")
private @Nullable Output galleryImageReference;
/**
* @return Required if creating from a Gallery Image. The id/sharedGalleryImageId/communityGalleryImageId of the ImageDiskReference will be the ARM id of the shared galley image version from which to create a disk.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy