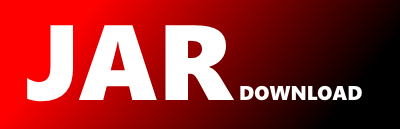
com.pulumi.azurenative.compute.outputs.CreationDataResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.ImageDiskReferenceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CreationDataResponse {
/**
* @return This enumerates the possible sources of a disk's creation.
*
*/
private String createOption;
/**
* @return Required if creating from a Gallery Image. The id/sharedGalleryImageId/communityGalleryImageId of the ImageDiskReference will be the ARM id of the shared galley image version from which to create a disk.
*
*/
private @Nullable ImageDiskReferenceResponse galleryImageReference;
/**
* @return Disk source information for PIR or user images.
*
*/
private @Nullable ImageDiskReferenceResponse imageReference;
/**
* @return Logical sector size in bytes for Ultra disks. Supported values are 512 ad 4096. 4096 is the default.
*
*/
private @Nullable Integer logicalSectorSize;
/**
* @return Set this flag to true to get a boost on the performance target of the disk deployed, see here on the respective performance target. This flag can only be set on disk creation time and cannot be disabled after enabled.
*
*/
private @Nullable Boolean performancePlus;
/**
* @return If createOption is ImportSecure, this is the URI of a blob to be imported into VM guest state.
*
*/
private @Nullable String securityDataUri;
/**
* @return If createOption is Copy, this is the ARM id of the source snapshot or disk.
*
*/
private @Nullable String sourceResourceId;
/**
* @return If this field is set, this is the unique id identifying the source of this resource.
*
*/
private String sourceUniqueId;
/**
* @return If createOption is Import, this is the URI of a blob to be imported into a managed disk.
*
*/
private @Nullable String sourceUri;
/**
* @return Required if createOption is Import. The Azure Resource Manager identifier of the storage account containing the blob to import as a disk.
*
*/
private @Nullable String storageAccountId;
/**
* @return If createOption is Upload, this is the size of the contents of the upload including the VHD footer. This value should be between 20972032 (20 MiB + 512 bytes for the VHD footer) and 35183298347520 bytes (32 TiB + 512 bytes for the VHD footer).
*
*/
private @Nullable Double uploadSizeBytes;
private CreationDataResponse() {}
/**
* @return This enumerates the possible sources of a disk's creation.
*
*/
public String createOption() {
return this.createOption;
}
/**
* @return Required if creating from a Gallery Image. The id/sharedGalleryImageId/communityGalleryImageId of the ImageDiskReference will be the ARM id of the shared galley image version from which to create a disk.
*
*/
public Optional galleryImageReference() {
return Optional.ofNullable(this.galleryImageReference);
}
/**
* @return Disk source information for PIR or user images.
*
*/
public Optional imageReference() {
return Optional.ofNullable(this.imageReference);
}
/**
* @return Logical sector size in bytes for Ultra disks. Supported values are 512 ad 4096. 4096 is the default.
*
*/
public Optional logicalSectorSize() {
return Optional.ofNullable(this.logicalSectorSize);
}
/**
* @return Set this flag to true to get a boost on the performance target of the disk deployed, see here on the respective performance target. This flag can only be set on disk creation time and cannot be disabled after enabled.
*
*/
public Optional performancePlus() {
return Optional.ofNullable(this.performancePlus);
}
/**
* @return If createOption is ImportSecure, this is the URI of a blob to be imported into VM guest state.
*
*/
public Optional securityDataUri() {
return Optional.ofNullable(this.securityDataUri);
}
/**
* @return If createOption is Copy, this is the ARM id of the source snapshot or disk.
*
*/
public Optional sourceResourceId() {
return Optional.ofNullable(this.sourceResourceId);
}
/**
* @return If this field is set, this is the unique id identifying the source of this resource.
*
*/
public String sourceUniqueId() {
return this.sourceUniqueId;
}
/**
* @return If createOption is Import, this is the URI of a blob to be imported into a managed disk.
*
*/
public Optional sourceUri() {
return Optional.ofNullable(this.sourceUri);
}
/**
* @return Required if createOption is Import. The Azure Resource Manager identifier of the storage account containing the blob to import as a disk.
*
*/
public Optional storageAccountId() {
return Optional.ofNullable(this.storageAccountId);
}
/**
* @return If createOption is Upload, this is the size of the contents of the upload including the VHD footer. This value should be between 20972032 (20 MiB + 512 bytes for the VHD footer) and 35183298347520 bytes (32 TiB + 512 bytes for the VHD footer).
*
*/
public Optional uploadSizeBytes() {
return Optional.ofNullable(this.uploadSizeBytes);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CreationDataResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String createOption;
private @Nullable ImageDiskReferenceResponse galleryImageReference;
private @Nullable ImageDiskReferenceResponse imageReference;
private @Nullable Integer logicalSectorSize;
private @Nullable Boolean performancePlus;
private @Nullable String securityDataUri;
private @Nullable String sourceResourceId;
private String sourceUniqueId;
private @Nullable String sourceUri;
private @Nullable String storageAccountId;
private @Nullable Double uploadSizeBytes;
public Builder() {}
public Builder(CreationDataResponse defaults) {
Objects.requireNonNull(defaults);
this.createOption = defaults.createOption;
this.galleryImageReference = defaults.galleryImageReference;
this.imageReference = defaults.imageReference;
this.logicalSectorSize = defaults.logicalSectorSize;
this.performancePlus = defaults.performancePlus;
this.securityDataUri = defaults.securityDataUri;
this.sourceResourceId = defaults.sourceResourceId;
this.sourceUniqueId = defaults.sourceUniqueId;
this.sourceUri = defaults.sourceUri;
this.storageAccountId = defaults.storageAccountId;
this.uploadSizeBytes = defaults.uploadSizeBytes;
}
@CustomType.Setter
public Builder createOption(String createOption) {
if (createOption == null) {
throw new MissingRequiredPropertyException("CreationDataResponse", "createOption");
}
this.createOption = createOption;
return this;
}
@CustomType.Setter
public Builder galleryImageReference(@Nullable ImageDiskReferenceResponse galleryImageReference) {
this.galleryImageReference = galleryImageReference;
return this;
}
@CustomType.Setter
public Builder imageReference(@Nullable ImageDiskReferenceResponse imageReference) {
this.imageReference = imageReference;
return this;
}
@CustomType.Setter
public Builder logicalSectorSize(@Nullable Integer logicalSectorSize) {
this.logicalSectorSize = logicalSectorSize;
return this;
}
@CustomType.Setter
public Builder performancePlus(@Nullable Boolean performancePlus) {
this.performancePlus = performancePlus;
return this;
}
@CustomType.Setter
public Builder securityDataUri(@Nullable String securityDataUri) {
this.securityDataUri = securityDataUri;
return this;
}
@CustomType.Setter
public Builder sourceResourceId(@Nullable String sourceResourceId) {
this.sourceResourceId = sourceResourceId;
return this;
}
@CustomType.Setter
public Builder sourceUniqueId(String sourceUniqueId) {
if (sourceUniqueId == null) {
throw new MissingRequiredPropertyException("CreationDataResponse", "sourceUniqueId");
}
this.sourceUniqueId = sourceUniqueId;
return this;
}
@CustomType.Setter
public Builder sourceUri(@Nullable String sourceUri) {
this.sourceUri = sourceUri;
return this;
}
@CustomType.Setter
public Builder storageAccountId(@Nullable String storageAccountId) {
this.storageAccountId = storageAccountId;
return this;
}
@CustomType.Setter
public Builder uploadSizeBytes(@Nullable Double uploadSizeBytes) {
this.uploadSizeBytes = uploadSizeBytes;
return this;
}
public CreationDataResponse build() {
final var _resultValue = new CreationDataResponse();
_resultValue.createOption = createOption;
_resultValue.galleryImageReference = galleryImageReference;
_resultValue.imageReference = imageReference;
_resultValue.logicalSectorSize = logicalSectorSize;
_resultValue.performancePlus = performancePlus;
_resultValue.securityDataUri = securityDataUri;
_resultValue.sourceResourceId = sourceResourceId;
_resultValue.sourceUniqueId = sourceUniqueId;
_resultValue.sourceUri = sourceUri;
_resultValue.storageAccountId = storageAccountId;
_resultValue.uploadSizeBytes = uploadSizeBytes;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy