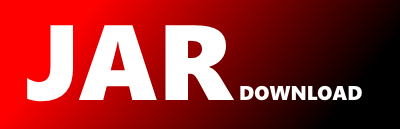
com.pulumi.azurenative.compute.outputs.EncryptionSetIdentityResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.UserAssignedIdentitiesResponseUserAssignedIdentities;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class EncryptionSetIdentityResponse {
/**
* @return The object id of the Managed Identity Resource. This will be sent to the RP from ARM via the x-ms-identity-principal-id header in the PUT request if the resource has a systemAssigned(implicit) identity
*
*/
private String principalId;
/**
* @return The tenant id of the Managed Identity Resource. This will be sent to the RP from ARM via the x-ms-client-tenant-id header in the PUT request if the resource has a systemAssigned(implicit) identity
*
*/
private String tenantId;
/**
* @return The type of Managed Identity used by the DiskEncryptionSet. Only SystemAssigned is supported for new creations. Disk Encryption Sets can be updated with Identity type None during migration of subscription to a new Azure Active Directory tenant; it will cause the encrypted resources to lose access to the keys.
*
*/
private @Nullable String type;
/**
* @return The list of user identities associated with the disk encryption set. The user identity dictionary key references will be ARM resource ids in the form: '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ManagedIdentity/userAssignedIdentities/{identityName}'.
*
*/
private @Nullable Map userAssignedIdentities;
private EncryptionSetIdentityResponse() {}
/**
* @return The object id of the Managed Identity Resource. This will be sent to the RP from ARM via the x-ms-identity-principal-id header in the PUT request if the resource has a systemAssigned(implicit) identity
*
*/
public String principalId() {
return this.principalId;
}
/**
* @return The tenant id of the Managed Identity Resource. This will be sent to the RP from ARM via the x-ms-client-tenant-id header in the PUT request if the resource has a systemAssigned(implicit) identity
*
*/
public String tenantId() {
return this.tenantId;
}
/**
* @return The type of Managed Identity used by the DiskEncryptionSet. Only SystemAssigned is supported for new creations. Disk Encryption Sets can be updated with Identity type None during migration of subscription to a new Azure Active Directory tenant; it will cause the encrypted resources to lose access to the keys.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
/**
* @return The list of user identities associated with the disk encryption set. The user identity dictionary key references will be ARM resource ids in the form: '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ManagedIdentity/userAssignedIdentities/{identityName}'.
*
*/
public Map userAssignedIdentities() {
return this.userAssignedIdentities == null ? Map.of() : this.userAssignedIdentities;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EncryptionSetIdentityResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String principalId;
private String tenantId;
private @Nullable String type;
private @Nullable Map userAssignedIdentities;
public Builder() {}
public Builder(EncryptionSetIdentityResponse defaults) {
Objects.requireNonNull(defaults);
this.principalId = defaults.principalId;
this.tenantId = defaults.tenantId;
this.type = defaults.type;
this.userAssignedIdentities = defaults.userAssignedIdentities;
}
@CustomType.Setter
public Builder principalId(String principalId) {
if (principalId == null) {
throw new MissingRequiredPropertyException("EncryptionSetIdentityResponse", "principalId");
}
this.principalId = principalId;
return this;
}
@CustomType.Setter
public Builder tenantId(String tenantId) {
if (tenantId == null) {
throw new MissingRequiredPropertyException("EncryptionSetIdentityResponse", "tenantId");
}
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
@CustomType.Setter
public Builder userAssignedIdentities(@Nullable Map userAssignedIdentities) {
this.userAssignedIdentities = userAssignedIdentities;
return this;
}
public EncryptionSetIdentityResponse build() {
final var _resultValue = new EncryptionSetIdentityResponse();
_resultValue.principalId = principalId;
_resultValue.tenantId = tenantId;
_resultValue.type = type;
_resultValue.userAssignedIdentities = userAssignedIdentities;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy