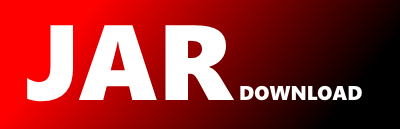
com.pulumi.azurenative.compute.outputs.GetAvailabilitySetResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.InstanceViewStatusResponse;
import com.pulumi.azurenative.compute.outputs.SkuResponse;
import com.pulumi.azurenative.compute.outputs.SubResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAvailabilitySetResult {
/**
* @return Resource Id
*
*/
private String id;
/**
* @return Resource location
*
*/
private String location;
/**
* @return Resource name
*
*/
private String name;
/**
* @return Fault Domain count.
*
*/
private @Nullable Integer platformFaultDomainCount;
/**
* @return Update Domain count.
*
*/
private @Nullable Integer platformUpdateDomainCount;
/**
* @return Specifies information about the proximity placement group that the availability set should be assigned to. Minimum api-version: 2018-04-01.
*
*/
private @Nullable SubResourceResponse proximityPlacementGroup;
/**
* @return Sku of the availability set, only name is required to be set. See AvailabilitySetSkuTypes for possible set of values. Use 'Aligned' for virtual machines with managed disks and 'Classic' for virtual machines with unmanaged disks. Default value is 'Classic'.
*
*/
private @Nullable SkuResponse sku;
/**
* @return The resource status information.
*
*/
private List statuses;
/**
* @return Resource tags
*
*/
private @Nullable Map tags;
/**
* @return Resource type
*
*/
private String type;
/**
* @return A list of references to all virtual machines in the availability set.
*
*/
private @Nullable List virtualMachines;
private GetAvailabilitySetResult() {}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return Resource location
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name
*
*/
public String name() {
return this.name;
}
/**
* @return Fault Domain count.
*
*/
public Optional platformFaultDomainCount() {
return Optional.ofNullable(this.platformFaultDomainCount);
}
/**
* @return Update Domain count.
*
*/
public Optional platformUpdateDomainCount() {
return Optional.ofNullable(this.platformUpdateDomainCount);
}
/**
* @return Specifies information about the proximity placement group that the availability set should be assigned to. Minimum api-version: 2018-04-01.
*
*/
public Optional proximityPlacementGroup() {
return Optional.ofNullable(this.proximityPlacementGroup);
}
/**
* @return Sku of the availability set, only name is required to be set. See AvailabilitySetSkuTypes for possible set of values. Use 'Aligned' for virtual machines with managed disks and 'Classic' for virtual machines with unmanaged disks. Default value is 'Classic'.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return The resource status information.
*
*/
public List statuses() {
return this.statuses;
}
/**
* @return Resource tags
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type
*
*/
public String type() {
return this.type;
}
/**
* @return A list of references to all virtual machines in the availability set.
*
*/
public List virtualMachines() {
return this.virtualMachines == null ? List.of() : this.virtualMachines;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAvailabilitySetResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String id;
private String location;
private String name;
private @Nullable Integer platformFaultDomainCount;
private @Nullable Integer platformUpdateDomainCount;
private @Nullable SubResourceResponse proximityPlacementGroup;
private @Nullable SkuResponse sku;
private List statuses;
private @Nullable Map tags;
private String type;
private @Nullable List virtualMachines;
public Builder() {}
public Builder(GetAvailabilitySetResult defaults) {
Objects.requireNonNull(defaults);
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.platformFaultDomainCount = defaults.platformFaultDomainCount;
this.platformUpdateDomainCount = defaults.platformUpdateDomainCount;
this.proximityPlacementGroup = defaults.proximityPlacementGroup;
this.sku = defaults.sku;
this.statuses = defaults.statuses;
this.tags = defaults.tags;
this.type = defaults.type;
this.virtualMachines = defaults.virtualMachines;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAvailabilitySetResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetAvailabilitySetResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAvailabilitySetResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder platformFaultDomainCount(@Nullable Integer platformFaultDomainCount) {
this.platformFaultDomainCount = platformFaultDomainCount;
return this;
}
@CustomType.Setter
public Builder platformUpdateDomainCount(@Nullable Integer platformUpdateDomainCount) {
this.platformUpdateDomainCount = platformUpdateDomainCount;
return this;
}
@CustomType.Setter
public Builder proximityPlacementGroup(@Nullable SubResourceResponse proximityPlacementGroup) {
this.proximityPlacementGroup = proximityPlacementGroup;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable SkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder statuses(List statuses) {
if (statuses == null) {
throw new MissingRequiredPropertyException("GetAvailabilitySetResult", "statuses");
}
this.statuses = statuses;
return this;
}
public Builder statuses(InstanceViewStatusResponse... statuses) {
return statuses(List.of(statuses));
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetAvailabilitySetResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualMachines(@Nullable List virtualMachines) {
this.virtualMachines = virtualMachines;
return this;
}
public Builder virtualMachines(SubResourceResponse... virtualMachines) {
return virtualMachines(List.of(virtualMachines));
}
public GetAvailabilitySetResult build() {
final var _resultValue = new GetAvailabilitySetResult();
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.platformFaultDomainCount = platformFaultDomainCount;
_resultValue.platformUpdateDomainCount = platformUpdateDomainCount;
_resultValue.proximityPlacementGroup = proximityPlacementGroup;
_resultValue.sku = sku;
_resultValue.statuses = statuses;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.virtualMachines = virtualMachines;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy