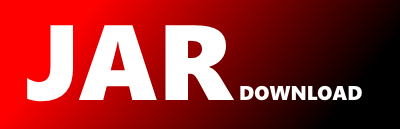
com.pulumi.azurenative.compute.outputs.ImageOSDiskResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.DiskEncryptionSetParametersResponse;
import com.pulumi.azurenative.compute.outputs.SubResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ImageOSDiskResponse {
/**
* @return The Virtual Hard Disk.
*
*/
private @Nullable String blobUri;
/**
* @return Specifies the caching requirements. Possible values are: **None,** **ReadOnly,** **ReadWrite.** The default values are: **None for Standard storage. ReadOnly for Premium storage.**
*
*/
private @Nullable String caching;
/**
* @return Specifies the customer managed disk encryption set resource id for the managed image disk.
*
*/
private @Nullable DiskEncryptionSetParametersResponse diskEncryptionSet;
/**
* @return Specifies the size of empty data disks in gigabytes. This element can be used to overwrite the name of the disk in a virtual machine image. This value cannot be larger than 1023 GB.
*
*/
private @Nullable Integer diskSizeGB;
/**
* @return The managedDisk.
*
*/
private @Nullable SubResourceResponse managedDisk;
/**
* @return The OS State. For managed images, use Generalized.
*
*/
private String osState;
/**
* @return This property allows you to specify the type of the OS that is included in the disk if creating a VM from a custom image. Possible values are: **Windows,** **Linux.**
*
*/
private String osType;
/**
* @return The snapshot.
*
*/
private @Nullable SubResourceResponse snapshot;
/**
* @return Specifies the storage account type for the managed disk. NOTE: UltraSSD_LRS can only be used with data disks, it cannot be used with OS Disk.
*
*/
private @Nullable String storageAccountType;
private ImageOSDiskResponse() {}
/**
* @return The Virtual Hard Disk.
*
*/
public Optional blobUri() {
return Optional.ofNullable(this.blobUri);
}
/**
* @return Specifies the caching requirements. Possible values are: **None,** **ReadOnly,** **ReadWrite.** The default values are: **None for Standard storage. ReadOnly for Premium storage.**
*
*/
public Optional caching() {
return Optional.ofNullable(this.caching);
}
/**
* @return Specifies the customer managed disk encryption set resource id for the managed image disk.
*
*/
public Optional diskEncryptionSet() {
return Optional.ofNullable(this.diskEncryptionSet);
}
/**
* @return Specifies the size of empty data disks in gigabytes. This element can be used to overwrite the name of the disk in a virtual machine image. This value cannot be larger than 1023 GB.
*
*/
public Optional diskSizeGB() {
return Optional.ofNullable(this.diskSizeGB);
}
/**
* @return The managedDisk.
*
*/
public Optional managedDisk() {
return Optional.ofNullable(this.managedDisk);
}
/**
* @return The OS State. For managed images, use Generalized.
*
*/
public String osState() {
return this.osState;
}
/**
* @return This property allows you to specify the type of the OS that is included in the disk if creating a VM from a custom image. Possible values are: **Windows,** **Linux.**
*
*/
public String osType() {
return this.osType;
}
/**
* @return The snapshot.
*
*/
public Optional snapshot() {
return Optional.ofNullable(this.snapshot);
}
/**
* @return Specifies the storage account type for the managed disk. NOTE: UltraSSD_LRS can only be used with data disks, it cannot be used with OS Disk.
*
*/
public Optional storageAccountType() {
return Optional.ofNullable(this.storageAccountType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ImageOSDiskResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String blobUri;
private @Nullable String caching;
private @Nullable DiskEncryptionSetParametersResponse diskEncryptionSet;
private @Nullable Integer diskSizeGB;
private @Nullable SubResourceResponse managedDisk;
private String osState;
private String osType;
private @Nullable SubResourceResponse snapshot;
private @Nullable String storageAccountType;
public Builder() {}
public Builder(ImageOSDiskResponse defaults) {
Objects.requireNonNull(defaults);
this.blobUri = defaults.blobUri;
this.caching = defaults.caching;
this.diskEncryptionSet = defaults.diskEncryptionSet;
this.diskSizeGB = defaults.diskSizeGB;
this.managedDisk = defaults.managedDisk;
this.osState = defaults.osState;
this.osType = defaults.osType;
this.snapshot = defaults.snapshot;
this.storageAccountType = defaults.storageAccountType;
}
@CustomType.Setter
public Builder blobUri(@Nullable String blobUri) {
this.blobUri = blobUri;
return this;
}
@CustomType.Setter
public Builder caching(@Nullable String caching) {
this.caching = caching;
return this;
}
@CustomType.Setter
public Builder diskEncryptionSet(@Nullable DiskEncryptionSetParametersResponse diskEncryptionSet) {
this.diskEncryptionSet = diskEncryptionSet;
return this;
}
@CustomType.Setter
public Builder diskSizeGB(@Nullable Integer diskSizeGB) {
this.diskSizeGB = diskSizeGB;
return this;
}
@CustomType.Setter
public Builder managedDisk(@Nullable SubResourceResponse managedDisk) {
this.managedDisk = managedDisk;
return this;
}
@CustomType.Setter
public Builder osState(String osState) {
if (osState == null) {
throw new MissingRequiredPropertyException("ImageOSDiskResponse", "osState");
}
this.osState = osState;
return this;
}
@CustomType.Setter
public Builder osType(String osType) {
if (osType == null) {
throw new MissingRequiredPropertyException("ImageOSDiskResponse", "osType");
}
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder snapshot(@Nullable SubResourceResponse snapshot) {
this.snapshot = snapshot;
return this;
}
@CustomType.Setter
public Builder storageAccountType(@Nullable String storageAccountType) {
this.storageAccountType = storageAccountType;
return this;
}
public ImageOSDiskResponse build() {
final var _resultValue = new ImageOSDiskResponse();
_resultValue.blobUri = blobUri;
_resultValue.caching = caching;
_resultValue.diskEncryptionSet = diskEncryptionSet;
_resultValue.diskSizeGB = diskSizeGB;
_resultValue.managedDisk = managedDisk;
_resultValue.osState = osState;
_resultValue.osType = osType;
_resultValue.snapshot = snapshot;
_resultValue.storageAccountType = storageAccountType;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy