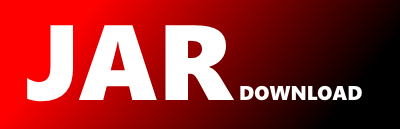
com.pulumi.azurenative.compute.outputs.VirtualMachineRunCommandInstanceViewResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.InstanceViewStatusResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VirtualMachineRunCommandInstanceViewResponse {
/**
* @return Script end time.
*
*/
private @Nullable String endTime;
/**
* @return Script error stream.
*
*/
private @Nullable String error;
/**
* @return Communicate script configuration errors or execution messages.
*
*/
private @Nullable String executionMessage;
/**
* @return Script execution status.
*
*/
private @Nullable String executionState;
/**
* @return Exit code returned from script execution.
*
*/
private @Nullable Integer exitCode;
/**
* @return Script output stream.
*
*/
private @Nullable String output;
/**
* @return Script start time.
*
*/
private @Nullable String startTime;
/**
* @return The resource status information.
*
*/
private @Nullable List statuses;
private VirtualMachineRunCommandInstanceViewResponse() {}
/**
* @return Script end time.
*
*/
public Optional endTime() {
return Optional.ofNullable(this.endTime);
}
/**
* @return Script error stream.
*
*/
public Optional error() {
return Optional.ofNullable(this.error);
}
/**
* @return Communicate script configuration errors or execution messages.
*
*/
public Optional executionMessage() {
return Optional.ofNullable(this.executionMessage);
}
/**
* @return Script execution status.
*
*/
public Optional executionState() {
return Optional.ofNullable(this.executionState);
}
/**
* @return Exit code returned from script execution.
*
*/
public Optional exitCode() {
return Optional.ofNullable(this.exitCode);
}
/**
* @return Script output stream.
*
*/
public Optional output() {
return Optional.ofNullable(this.output);
}
/**
* @return Script start time.
*
*/
public Optional startTime() {
return Optional.ofNullable(this.startTime);
}
/**
* @return The resource status information.
*
*/
public List statuses() {
return this.statuses == null ? List.of() : this.statuses;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VirtualMachineRunCommandInstanceViewResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String endTime;
private @Nullable String error;
private @Nullable String executionMessage;
private @Nullable String executionState;
private @Nullable Integer exitCode;
private @Nullable String output;
private @Nullable String startTime;
private @Nullable List statuses;
public Builder() {}
public Builder(VirtualMachineRunCommandInstanceViewResponse defaults) {
Objects.requireNonNull(defaults);
this.endTime = defaults.endTime;
this.error = defaults.error;
this.executionMessage = defaults.executionMessage;
this.executionState = defaults.executionState;
this.exitCode = defaults.exitCode;
this.output = defaults.output;
this.startTime = defaults.startTime;
this.statuses = defaults.statuses;
}
@CustomType.Setter
public Builder endTime(@Nullable String endTime) {
this.endTime = endTime;
return this;
}
@CustomType.Setter
public Builder error(@Nullable String error) {
this.error = error;
return this;
}
@CustomType.Setter
public Builder executionMessage(@Nullable String executionMessage) {
this.executionMessage = executionMessage;
return this;
}
@CustomType.Setter
public Builder executionState(@Nullable String executionState) {
this.executionState = executionState;
return this;
}
@CustomType.Setter
public Builder exitCode(@Nullable Integer exitCode) {
this.exitCode = exitCode;
return this;
}
@CustomType.Setter
public Builder output(@Nullable String output) {
this.output = output;
return this;
}
@CustomType.Setter
public Builder startTime(@Nullable String startTime) {
this.startTime = startTime;
return this;
}
@CustomType.Setter
public Builder statuses(@Nullable List statuses) {
this.statuses = statuses;
return this;
}
public Builder statuses(InstanceViewStatusResponse... statuses) {
return statuses(List.of(statuses));
}
public VirtualMachineRunCommandInstanceViewResponse build() {
final var _resultValue = new VirtualMachineRunCommandInstanceViewResponse();
_resultValue.endTime = endTime;
_resultValue.error = error;
_resultValue.executionMessage = executionMessage;
_resultValue.executionState = executionState;
_resultValue.exitCode = exitCode;
_resultValue.output = output;
_resultValue.startTime = startTime;
_resultValue.statuses = statuses;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy