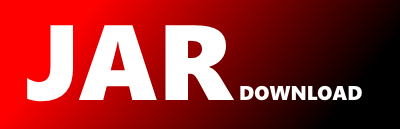
com.pulumi.azurenative.containerinstance.inputs.ContainerArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerinstance.inputs;
import com.pulumi.azurenative.containerinstance.inputs.ConfigMapArgs;
import com.pulumi.azurenative.containerinstance.inputs.ContainerPortArgs;
import com.pulumi.azurenative.containerinstance.inputs.ContainerProbeArgs;
import com.pulumi.azurenative.containerinstance.inputs.EnvironmentVariableArgs;
import com.pulumi.azurenative.containerinstance.inputs.ResourceRequirementsArgs;
import com.pulumi.azurenative.containerinstance.inputs.SecurityContextDefinitionArgs;
import com.pulumi.azurenative.containerinstance.inputs.VolumeMountArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A container instance.
*
*/
public final class ContainerArgs extends com.pulumi.resources.ResourceArgs {
public static final ContainerArgs Empty = new ContainerArgs();
/**
* The commands to execute within the container instance in exec form.
*
*/
@Import(name="command")
private @Nullable Output> command;
/**
* @return The commands to execute within the container instance in exec form.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy