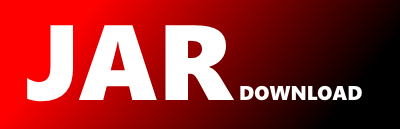
com.pulumi.azurenative.containerregistry.RegistryArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerregistry;
import com.pulumi.azurenative.containerregistry.enums.NetworkRuleBypassOptions;
import com.pulumi.azurenative.containerregistry.enums.PublicNetworkAccess;
import com.pulumi.azurenative.containerregistry.enums.ZoneRedundancy;
import com.pulumi.azurenative.containerregistry.inputs.EncryptionPropertyArgs;
import com.pulumi.azurenative.containerregistry.inputs.IdentityPropertiesArgs;
import com.pulumi.azurenative.containerregistry.inputs.NetworkRuleSetArgs;
import com.pulumi.azurenative.containerregistry.inputs.PoliciesArgs;
import com.pulumi.azurenative.containerregistry.inputs.SkuArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RegistryArgs extends com.pulumi.resources.ResourceArgs {
public static final RegistryArgs Empty = new RegistryArgs();
/**
* The value that indicates whether the admin user is enabled.
*
*/
@Import(name="adminUserEnabled")
private @Nullable Output adminUserEnabled;
/**
* @return The value that indicates whether the admin user is enabled.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy